How to Get Selected Option From Dropdown in jQuery
-
Use the
.val()
Method to Get Selected Option From Dropdown -
Use the
.find()
Method to Get Selected Option From Dropdown -
Use the
.filter()
Method to Get Selected Option From Dropdown -
Use the
:selected
Selector jQuery Extension to Get Selected Option From Dropdown -
Use the
.text()
Method to Get Selected Option Text
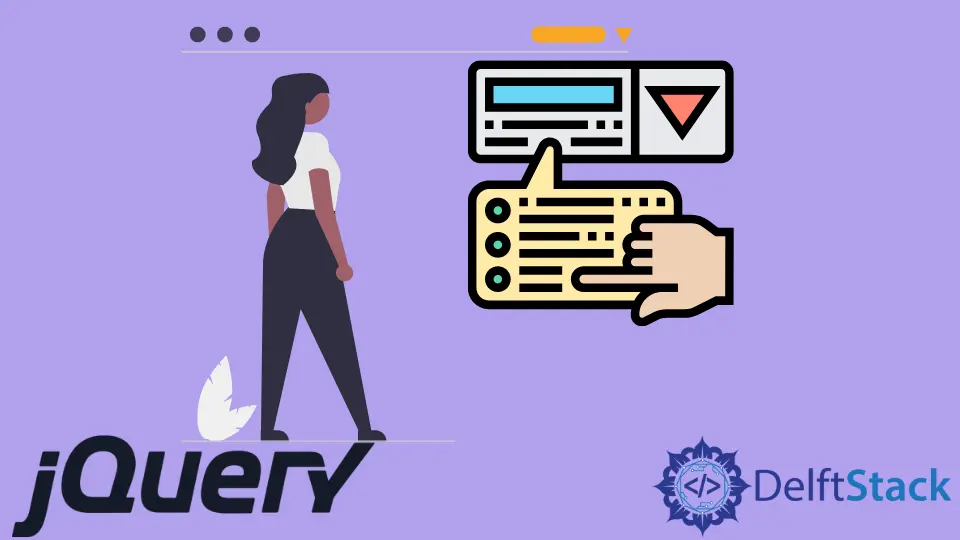
This tutorial will tackle how to get the selected option from the dropdown using jQuery. We will use the .val()
, .find()
, and .filter()
methods.
Also, we’ll discuss how to get the selected option text with .text()
.
Use the .val()
Method to Get Selected Option From Dropdown
Using the.val()
method, we get the value of the first element in our collection of selected elements. It has a special case when you apply it to a dropdown select element, and it directly gets the value of the selected option in this situation.
We use this special form of the .val()
method in jQuery to get the selected option.
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is :
${$("#first-level").val()} <br>`);
});
Our #first-level
ID element is a single-choice select box. When the callback fires, we attach an event handler to listen for the "change"
event.
The callback displays the newly selected option by running the .html()
method on a <p>
element with the ID p-first
.
The key execution line is the code:
$("#first-level").val()
The .val()
method runs on the select box with the ID first-level
to directly get the value of the selected option. Another special case of the .val()
method lets us extend its use to jQuery select option by value for multiple-choice select boxes.
When we run the .val()
method on a multiple-choice select box, it returns an array with the values of all the selected options.
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
The val()
method on the multiple-choice select box with the ID multiple
returns an array of the selected options’ values. We then chain the .map()
method to iterate over them and return an array of <span>
elements with the values as text to display on the page.
We can also use the .val()
method jQuery to get selected options from grouped select boxes.
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
The grouped select box with ID grouped
has its options separated into different groups with the <optgroup>
tag. We easily get the selected option with the .val()
method.
Full Code:
//gets the selected option with the jQuery val() method
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
// this directly gets the selected option with the jQuery val() method
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
Output:
You can read in detail about the jQuery .val() method here
Use the .find()
Method to Get Selected Option From Dropdown
We can narrow down our collection of elements to only those selected with the .find()
method. Then, apply the .val()
method in its general form to get the value of the selected option jQuery.
The .find()
method returns all descendants of the element it runs on that match the selector argument we pass to it. Like so:
$("ul .vegetables").find("li .green")
The .find()
will return only green li
elements that are descendants of the .vegetables
class ul
element. We can use it to get selected options from single-choice dropdowns.
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").val()} <br>`);
});
We use the :selected
selector to first find only those option
descendant elements of the first-level
select
box. We then chain the .val()
method in its general form to get the value.
We can also extend this method to multiple-choice select boxes.
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").find(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
We first find the collection of selected option
elements with the $("multiple").find(:selected)
code snippet. We then iterate them by chaining the .map()
method.
The callback collects the values of the selected options with the $(this).val()
chunk of code in an array of <span>
elements. Lastly, we chain the get()
and join()
methods to display these values in a nice piece of text.
We can use the same method for grouped select boxes.
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").find(":selected").val()
}`);
})
Full Code:
// we first select the particular option using the find method and then find its val indirectly here
// first-select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").find(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").find(":selected").val()
}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
Output:
Read in detail about the .find()
method here
Use the .filter()
Method to Get Selected Option From Dropdown
We can use the .filter()
method to collect only the selected options. Then we can run the .val()
method in its usual form to get the value of the selected option jQuery.
The .filter()
method differs from the .find()
method in that it does not select the descendants but only selects the matched elements from the collection it is called on. Like in the following snippet, we can use it to jQuery select option by value.
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level option").filter(":selected").val()} <br>`);
});
We use $("#first-level option")
selector to explicitly collect the option
elements from the first-level
select
element.
Remember, .filter()
does not automatically get the descendant elements like the .find()
method. We need to do it manually.
Then, we chain the .filter(":selected")
method to get the selected option
element, and chain the .val()
method to jQuery get selected option. We can do the same for multiple-choice select boxes as well.
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple option").filter(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
The code is similar with the .find()
solution above, except that we manually need to select option
elements with the $("#multiple option")
chunk before chaining the .filter()
method. Likewise, we can use this solution for grouped options and select dropdowns.
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped option").filter(":selected").val()
}`);
})
Full Code:
// we can get the selected option with indirect use of val() method and the filter() method
// first-select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level option").filter(":selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple option").filter(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped option").filter(":selected").val()
}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
Output:
Use the :selected
Selector jQuery Extension to Get Selected Option From Dropdown
We can also directly select only the selected options with the jQuery :selected
extension instead of using the .find()
or .filter()
methods.
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level :selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple :selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped :selected").val()}`);
})
The code is the same as the other methods above, except we use the $("first-level :selected")
selector expression before chaining the other methods.
Output:
It is better to use .find()
or .filter()
first because the :selected
pseudo-selector is a jQuery extension and not part of CSS. So, it does not benefit from the performance boost of native DOM methods like pure CSS selectors do.
The :checked
selector gets the selected options when applied to a 'select'
element. It is a pure CSS selector, so it performs better than the:selected
pseudo-selector.
Use the .text()
Method to Get Selected Option Text
You might want to find the text inside the option
element instead of value
. You can use the .text()
method to jQuery to get the selected option text.
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").text()} <br>`);
});
The rest of the code is the same as the other methods above, except we chain the .text()
method at the end instead of the .val()
method to get the selected option text.
Output: