从 jQuery 的下拉列表中获取选定的选项
-
使用
.val()
方法从下拉列表中获取选定的选项 -
使用
.find()
方法从下拉列表中获取选定的选项 -
使用
.filter()
方法从下拉列表中获取选定的选项 -
使用
:selected
选择器 jQuery 扩展从下拉列表中获取选定选项 -
使用
.text()
方法获取选定的选项文本
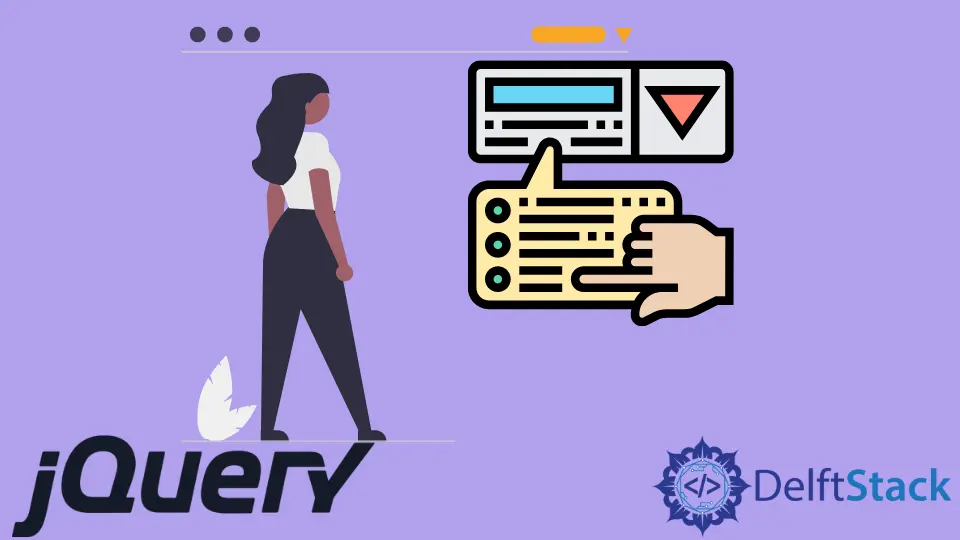
本教程将解决如何使用 jQuery 从下拉列表中获取选定的选项。我们将使用 .val()
、.find()
和 .filter()
方法。
此外,我们将讨论如何使用 .text()
获取选定的选项文本。
使用 .val()
方法从下拉列表中获取选定的选项
使用 .val()
方法,我们获得所选元素集合中第一个元素的值。当你将其应用于下拉选择元素时,它有一个特殊情况,在这种情况下它直接获取所选选项的值。
我们在 jQuery 中使用这种特殊形式的 .val()
方法来获取选定的选项。
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is :
${$("#first-level").val()} <br>`);
});
我们的 #first-level
ID 元素是一个单选选择框。当回调触发时,我们附加一个事件处理程序来监听 "change"
事件。
回调通过在 ID 为 p-first
的 <p>
元素上运行 .html()
方法来显示新选择的选项。
关键执行行是代码:
$("#first-level").val()
.val()
方法在 ID 为 first-level
的选择框上运行,以直接获取所选选项的值。 .val()
方法的另一个特殊情况让我们可以将它的使用扩展到 jQuery 多选选择框的按值选择选项。
当我们在多选框上运行 .val()
方法时,它会返回一个包含所有选定选项值的数组。
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
ID 为 multiple
的多项选择框上的 val()
方法返回所选选项值的数组。然后,我们链接 .map()
方法来迭代它们并返回一个 <span>
元素数组,其值作为文本显示在页面上。
我们还可以使用 jQuery 的 .val()
方法从分组的选择框中获取选定的选项。
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
ID 为 grouped
的分组选择框的选项使用 <optgroup>
标签分成不同的组。我们使用 .val()
方法轻松获得选定的选项。
完整代码:
//gets the selected option with the jQuery val() method
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
// this directly gets the selected option with the jQuery val() method
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
输出:
使用 .find()
方法从下拉列表中获取选定的选项
我们可以将我们的元素集合缩小到仅使用 .find()
方法选择的元素。然后,以一般形式应用 .val()
方法来获取所选选项 jQuery 的值。
.find()
方法返回它运行的与我们传递给它的选择器参数匹配的元素的所有后代。像这样:
$("ul .vegetables").find("li .green")
.find()
将仅返回绿色 li
元素,它们是 .vegetables
类 ul
元素的后代。我们可以使用它从单选下拉列表中获取选定的选项。
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").val()} <br>`);
});
我们使用 :selected
选择器首先找到``first-level
select
框的那些 option
后代元素。然后,我们以一般形式链接 .val()
方法以获取值。
我们还可以将此方法扩展到多项选择框。
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").find(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
我们首先使用 $("multiple").find(:selected)
代码片段找到选定 option
元素的集合。然后我们通过链接 .map()
方法来迭代它们。
回调通过 <span>
元素数组中的 $(this).val()
代码块收集所选选项的值。最后,我们链接 get()
和 join()
方法以在一段漂亮的文本中显示这些值。
我们可以对分组选择框使用相同的方法。
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").find(":selected").val()
}`);
})
完整代码:
// we first select the particular option using the find method and then find its val indirectly here
// first-select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").find(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").find(":selected").val()
}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
输出:
使用 .filter()
方法从下拉列表中获取选定的选项
我们可以使用 .filter()
方法仅收集选定的选项。然后我们可以以通常的形式运行 .val()
方法来获取所选选项 jQuery 的值。
.filter()
方法与 .find()
方法的不同之处在于它不选择后代,而仅从调用它的集合中选择匹配的元素。就像在下面的代码片段中一样,我们可以将它用于 jQuery 按值选择选项。
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level option").filter(":selected").val()} <br>`);
});
我们使用 $("#first-level option")
选择器从 first-level
select
元素中显式收集 option
元素。
请记住,.filter()
不会像 .find()
方法那样自动获取后代元素。我们需要手动完成。
然后,我们链接 .filter(":selected")
方法以获取选定的 option
元素,并将 .val()
方法链接到 jQuery 获取选定选项。我们也可以对多项选择框做同样的事情。
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple option").filter(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
代码与上面的 .find()
解决方案类似,除了我们需要在链接 .filter()
方法之前手动选择带有 $("#multiple option")
块的 option
元素。同样,我们可以将此解决方案用于分组选项并选择下拉菜单。
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped option").filter(":selected").val()
}`);
})
完整代码:
// we can get the selected option with indirect use of val() method and the filter() method
// first-select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level option").filter(":selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple option").filter(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped option").filter(":selected").val()
}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
输出:
使用 :selected
选择器 jQuery 扩展从下拉列表中获取选定选项
我们还可以使用 jQuery :selected
扩展名直接选择仅选定的选项,而不是使用 .find()
或 .filter()
方法。
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level :selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple :selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped :selected").val()}`);
})
代码与上面的其他方法相同,除了我们在链接其他方法之前使用了 $("first-level :selected")
选择器表达式。
输出:
最好先使用 .find()
或 .filter()
因为 :selected
伪选择器是 jQuery 扩展而不是 CSS 的一部分。因此,它不会像纯 CSS 选择器那样从原生 DOM 方法的性能提升中受益。
:checked
选择器在应用于 'select'
元素时获取选定的选项。它是一个纯 CSS 选择器,因此它的性能优于:selected
伪选择器。
使用 .text()
方法获取选定的选项文本
你可能希望在 option
元素而不是 value
中查找文本。你可以使用 jQuery 的 .text()
方法来获取选定的选项文本。
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").text()} <br>`);
});
其余代码与上面的其他方法相同,除了我们在末尾链接 .text()
方法而不是 .val()
方法来获取选定的选项文本。
输出: