jQuery의 드롭다운에서 선택한 옵션 가져오기
-
.val()
메서드를 사용하여 드롭다운에서 선택한 옵션 가져오기 -
.find()
메서드를 사용하여 드롭다운에서 선택한 옵션 가져오기 -
.filter()
메서드를 사용하여 드롭다운에서 선택한 옵션 가져오기 -
:selected
선택기 jQuery 확장을 사용하여 드롭다운에서 선택한 옵션 가져오기 -
.text()
메서드를 사용하여 선택한 옵션 텍스트 가져오기
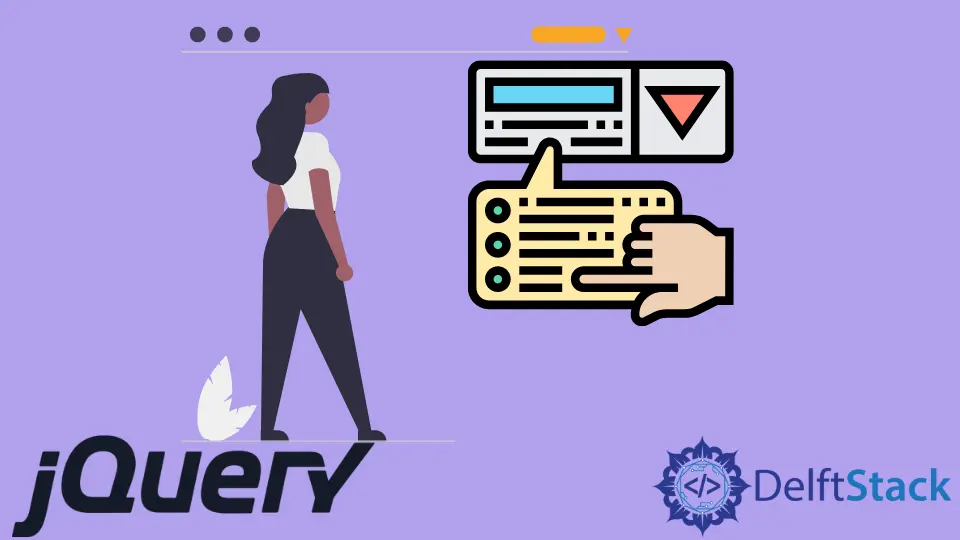
이 자습서에서는 jQuery를 사용하여 드롭다운에서 선택한 옵션을 가져오는 방법을 다룹니다. .val()
, .find()
및 .filter()
메서드를 사용합니다.
또한 .text()
를 사용하여 선택한 옵션 텍스트를 가져오는 방법에 대해 설명합니다.
.val()
메서드를 사용하여 드롭다운에서 선택한 옵션 가져오기
.val()
메서드를 사용하여 선택한 요소 모음에서 첫 번째 요소의 값을 얻습니다. 드롭다운 선택 요소에 적용할 때 특별한 경우가 있으며 이 상황에서 선택한 옵션의 값을 직접 가져옵니다.
jQuery에서 .val()
메소드의 이 특별한 형식을 사용하여 선택한 옵션을 가져옵니다.
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is :
${$("#first-level").val()} <br>`);
});
#first-level
ID 요소는 단일 선택 선택 상자입니다. 콜백이 실행되면 "change"
이벤트를 수신하기 위해 이벤트 핸들러를 연결합니다.
콜백은 ID가 p-first
인 <p>
요소에서 .html()
메서드를 실행하여 새로 선택한 옵션을 표시합니다.
핵심 실행 라인은 다음과 같은 코드입니다.
$("#first-level").val()
.val()
메서드는 first-level
ID의 선택 상자에서 실행되어 선택한 옵션의 값을 직접 가져옵니다. .val()
메소드의 또 다른 특별한 경우는 객관식 선택 상자에 대한 값으로 jQuery 선택 옵션으로 사용을 확장할 수 있습니다.
객관식 선택 상자에서 .val()
메서드를 실행하면 선택한 모든 옵션의 값이 포함된 배열이 반환됩니다.
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
ID가 multiple
인 객관식 선택 상자의 val()
메서드는 선택한 옵션 값의 배열을 반환합니다. 그런 다음 .map()
메서드를 연결하여 반복하고 값을 페이지에 표시할 텍스트로 포함하는 <span>
요소의 배열을 반환합니다.
.val()
메서드 jQuery를 사용하여 그룹화된 선택 상자에서 선택한 옵션을 가져올 수도 있습니다.
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
ID가 grouped
인 그룹화된 선택 상자에는 <optgroup>
태그를 사용하여 여러 그룹으로 구분된 옵션이 있습니다. .val()
메서드를 사용하여 선택한 옵션을 쉽게 얻을 수 있습니다.
전체 코드:
//gets the selected option with the jQuery val() method
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
// this directly gets the selected option with the jQuery val() method
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
출력:
여기에서 jQuery .val() 메서드에 대해 자세히 읽을 수 있습니다
.find()
메서드를 사용하여 드롭다운에서 선택한 옵션 가져오기
.find()
메서드로 선택한 요소로만 요소 컬렉션의 범위를 좁힐 수 있습니다. 그런 다음 일반적인 형식의 .val()
메서드를 적용하여 선택한 jQuery 옵션의 값을 가져옵니다.
.find()
메서드는 우리가 전달한 선택기 인수와 일치하는 요소에서 실행되는 모든 하위 항목을 반환합니다. 이렇게:
$("ul .vegetables").find("li .green")
.find()
는 .vegetables
클래스 ul
요소의 자손인 녹색 li
요소만 반환합니다. 단일 선택 드롭다운에서 선택한 옵션을 가져오는 데 사용할 수 있습니다.
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").val()} <br>`);
});
:selected
선택기를 사용하여 first-level
select
상자의 option
하위 요소만 먼저 “찾습니다”. 그런 다음 .val()
메서드를 일반적인 형식으로 연결하여 값을 얻습니다.
이 방법을 객관식 선택 상자로 확장할 수도 있습니다.
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").find(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
먼저 $("multiple").find(:selected)
코드 조각을 사용하여 선택된 option
요소 컬렉션을 찾습니다. 그런 다음 .map()
메서드를 연결하여 반복합니다.
콜백은 <span>
요소의 배열에서 $(this).val()
코드 청크로 선택한 옵션의 값을 수집합니다. 마지막으로 get()
및 join()
메서드를 연결하여 이러한 값을 멋진 텍스트로 표시합니다.
그룹화된 선택 상자에 대해 동일한 방법을 사용할 수 있습니다.
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").find(":selected").val()
}`);
})
전체 코드:
// we first select the particular option using the find method and then find its val indirectly here
// first-select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").find(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").find(":selected").val()
}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
출력:
.find()
메서드에 대한 자세한 내용은 여기를 참조하세요
.filter()
메서드를 사용하여 드롭다운에서 선택한 옵션 가져오기
.filter()
메서드를 사용하여 선택한 옵션만 수집할 수 있습니다. 그런 다음 일반적인 형식으로 .val()
메서드를 실행하여 선택한 jQuery 옵션의 값을 가져올 수 있습니다.
.filter()
메서드는 하위 항목을 선택하지 않고 호출된 컬렉션에서 일치하는 요소만 선택한다는 점에서 .find()
메서드와 다릅니다. 다음 스니펫과 같이 jQuery를 사용하여 값으로 옵션을 선택할 수 있습니다.
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level option").filter(":selected").val()} <br>`);
});
$("#first-level option")
선택기를 사용하여 first-level
select
요소에서 option
요소를 명시적으로 수집합니다.
.filter()
는 .find()
메서드와 같은 하위 요소를 자동으로 가져오지 않습니다. 수동으로 해야 합니다.
그런 다음 .filter(":selected")
메서드를 연결하여 선택한 option
요소를 가져오고 .val()
메서드를 jQuery get selected 옵션에 연결합니다. 객관식 선택 상자에 대해서도 동일한 작업을 수행할 수 있습니다.
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple option").filter(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
코드는 .filter()
메서드를 연결하기 전에 $("#multiple option")
청크가 있는 option
요소를 수동으로 선택해야 한다는 점을 제외하고 위의 .find()
솔루션과 유사합니다. 마찬가지로 그룹화된 옵션과 선택 드롭다운에 이 솔루션을 사용할 수 있습니다.
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped option").filter(":selected").val()
}`);
})
전체 코드:
// we can get the selected option with indirect use of val() method and the filter() method
// first-select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level option").filter(":selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple option").filter(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped option").filter(":selected").val()
}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
출력:
:selected
선택기 jQuery 확장을 사용하여 드롭다운에서 선택한 옵션 가져오기
.find()
또는 .filter()
메서드를 사용하는 대신 jQuery :selected
확장자를 사용하여 선택한 옵션만 직접 선택할 수도 있습니다.
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level :selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple :selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped :selected").val()}`);
})
코드는 다른 메소드를 연결하기 전에 $("first-level :selected")
selector 표현식을 사용한다는 점을 제외하고 위의 다른 메소드와 동일합니다.
출력:
:selected
의사 선택기는 CSS의 일부가 아닌 jQuery 확장이므로 .find()
또는 .filter()
를 먼저 사용하는 것이 좋습니다. 따라서 순수 CSS 선택기와 같은 기본 DOM 메서드의 성능 향상으로부터 이점을 얻지 못합니다.
:checked
선택기는 'select'
요소에 적용될 때 선택한 옵션을 가져옵니다. 순수한 CSS 선택기이므로 :selected
유사 선택기보다 성능이 좋습니다.
.text()
메서드를 사용하여 선택한 옵션 텍스트 가져오기
value
대신 option
요소 내에서 텍스트를 찾고 싶을 수도 있습니다. jQuery에 .text()
메서드를 사용하여 선택한 옵션 텍스트를 가져올 수 있습니다.
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").text()} <br>`);
});
나머지 코드는 선택한 옵션 텍스트를 가져오기 위해 .val()
메서드 대신 .text()
메서드를 끝에 연결하는 것을 제외하고 위의 다른 메서드와 동일합니다.
출력: