從 jQuery 的下拉選單中獲取選定的選項
-
使用
.val()
方法從下拉選單中獲取選定的選項 -
使用
.find()
方法從下拉選單中獲取選定的選項 -
使用
.filter()
方法從下拉選單中獲取選定的選項 -
使用
:selected
選擇器 jQuery 擴充套件從下拉選單中獲取選定選項 -
使用
.text()
方法獲取選定的選項文字
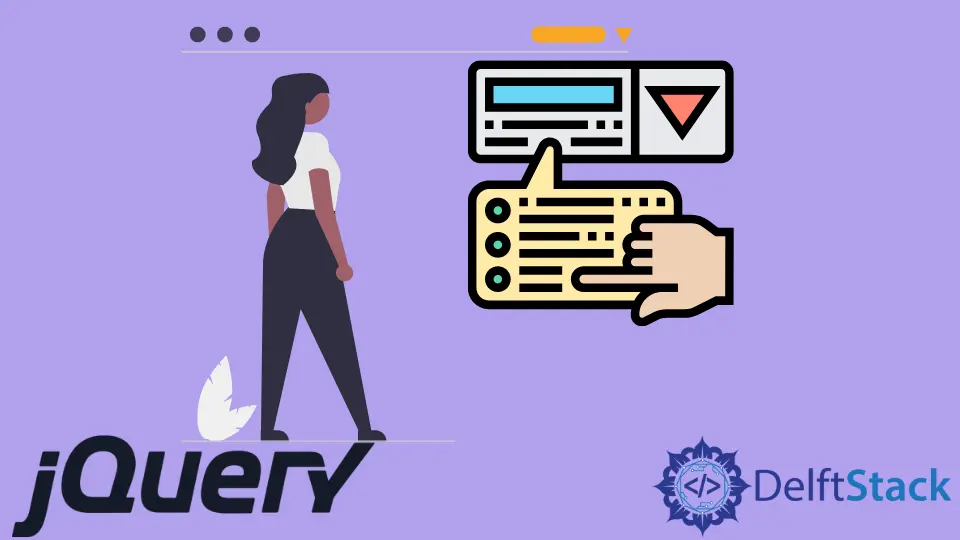
本教程將解決如何使用 jQuery 從下拉選單中獲取選定的選項。我們將使用 .val()
、.find()
和 .filter()
方法。
此外,我們將討論如何使用 .text()
獲取選定的選項文字。
使用 .val()
方法從下拉選單中獲取選定的選項
使用 .val()
方法,我們獲得所選元素集合中第一個元素的值。當你將其應用於下拉選擇元素時,它有一個特殊情況,在這種情況下它直接獲取所選選項的值。
我們在 jQuery 中使用這種特殊形式的 .val()
方法來獲取選定的選項。
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is :
${$("#first-level").val()} <br>`);
});
我們的 #first-level
ID 元素是一個單選選擇框。當回撥觸發時,我們附加一個事件處理程式來監聽 "change"
事件。
回撥通過在 ID 為 p-first
的 <p>
元素上執行 .html()
方法來顯示新選擇的選項。
關鍵執行行是程式碼:
$("#first-level").val()
.val()
方法在 ID 為 first-level
的選擇框上執行,以直接獲取所選選項的值。 .val()
方法的另一個特殊情況讓我們可以將它的使用擴充套件到 jQuery 多選選擇框的按值選擇選項。
當我們在多選框上執行 .val()
方法時,它會返回一個包含所有選定選項值的陣列。
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
ID 為 multiple
的多項選擇框上的 val()
方法返回所選選項值的陣列。然後,我們連結 .map()
方法來迭代它們並返回一個 <span>
元素陣列,其值作為文字顯示在頁面上。
我們還可以使用 jQuery 的 .val()
方法從分組的選擇框中獲取選定的選項。
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
ID 為 grouped
的分組選擇框的選項使用 <optgroup>
標籤分成不同的組。我們使用 .val()
方法輕鬆獲得選定的選項。
完整程式碼:
//gets the selected option with the jQuery val() method
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
// this directly gets the selected option with the jQuery val() method
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
輸出:
使用 .find()
方法從下拉選單中獲取選定的選項
我們可以將我們的元素集合縮小到僅使用 .find()
方法選擇的元素。然後,以一般形式應用 .val()
方法來獲取所選選項 jQuery 的值。
.find()
方法返回它執行的與我們傳遞給它的選擇器引數匹配的元素的所有後代。像這樣:
$("ul .vegetables").find("li .green")
.find()
將僅返回綠色 li
元素,它們是 .vegetables
類 ul
元素的後代。我們可以使用它從單選下拉選單中獲取選定的選項。
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").val()} <br>`);
});
我們使用 :selected
選擇器首先找到``first-level
select
框的那些 option
後代元素。然後,我們以一般形式連結 .val()
方法以獲取值。
我們還可以將此方法擴充套件到多項選擇框。
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").find(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
我們首先使用 $("multiple").find(:selected)
程式碼片段找到選定 option
元素的集合。然後我們通過連結 .map()
方法來迭代它們。
回撥通過 <span>
元素陣列中的 $(this).val()
程式碼塊收集所選選項的值。最後,我們連結 get()
和 join()
方法以在一段漂亮的文字中顯示這些值。
我們可以對分組選擇框使用相同的方法。
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").find(":selected").val()
}`);
})
完整程式碼:
// we first select the particular option using the find method and then find its val indirectly here
// first-select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").find(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").find(":selected").val()
}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
輸出:
使用 .filter()
方法從下拉選單中獲取選定的選項
我們可以使用 .filter()
方法僅收集選定的選項。然後我們可以以通常的形式執行 .val()
方法來獲取所選選項 jQuery 的值。
.filter()
方法與 .find()
方法的不同之處在於它不選擇後代,而僅從呼叫它的集合中選擇匹配的元素。就像在下面的程式碼片段中一樣,我們可以將它用於 jQuery 按值選擇選項。
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level option").filter(":selected").val()} <br>`);
});
我們使用 $("#first-level option")
選擇器從 first-level
select
元素中顯式收集 option
元素。
請記住,.filter()
不會像 .find()
方法那樣自動獲取後代元素。我們需要手動完成。
然後,我們連結 .filter(":selected")
方法以獲取選定的 option
元素,並將 .val()
方法連結到 jQuery 獲取選定選項。我們也可以對多項選擇框做同樣的事情。
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple option").filter(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
程式碼與上面的 .find()
解決方案類似,除了我們需要在連結 .filter()
方法之前手動選擇帶有 $("#multiple option")
塊的 option
元素。同樣,我們可以將此解決方案用於分組選項並選擇下拉選單。
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped option").filter(":selected").val()
}`);
})
完整程式碼:
// we can get the selected option with indirect use of val() method and the filter() method
// first-select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level option").filter(":selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple option").filter(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped option").filter(":selected").val()
}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
輸出:
使用 :selected
選擇器 jQuery 擴充套件從下拉選單中獲取選定選項
我們還可以使用 jQuery :selected
副檔名直接選擇僅選定的選項,而不是使用 .find()
或 .filter()
方法。
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level :selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple :selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped :selected").val()}`);
})
程式碼與上面的其他方法相同,除了我們在連結其他方法之前使用了 $("first-level :selected")
選擇器表示式。
輸出:
最好先使用 .find()
或 .filter()
因為 :selected
偽選擇器是 jQuery 擴充套件而不是 CSS 的一部分。因此,它不會像純 CSS 選擇器那樣從原生 DOM 方法的效能提升中受益。
:checked
選擇器在應用於 'select'
元素時獲取選定的選項。它是一個純 CSS 選擇器,因此它的效能優於:selected
偽選擇器。
使用 .text()
方法獲取選定的選項文字
你可能希望在 option
元素而不是 value
中查詢文字。你可以使用 jQuery 的 .text()
方法來獲取選定的選項文字。
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").text()} <br>`);
});
其餘程式碼與上面的其他方法相同,除了我們在末尾連結 .text()
方法而不是 .val()
方法來獲取選定的選項文字。
輸出: