How to Get Height in jQuery
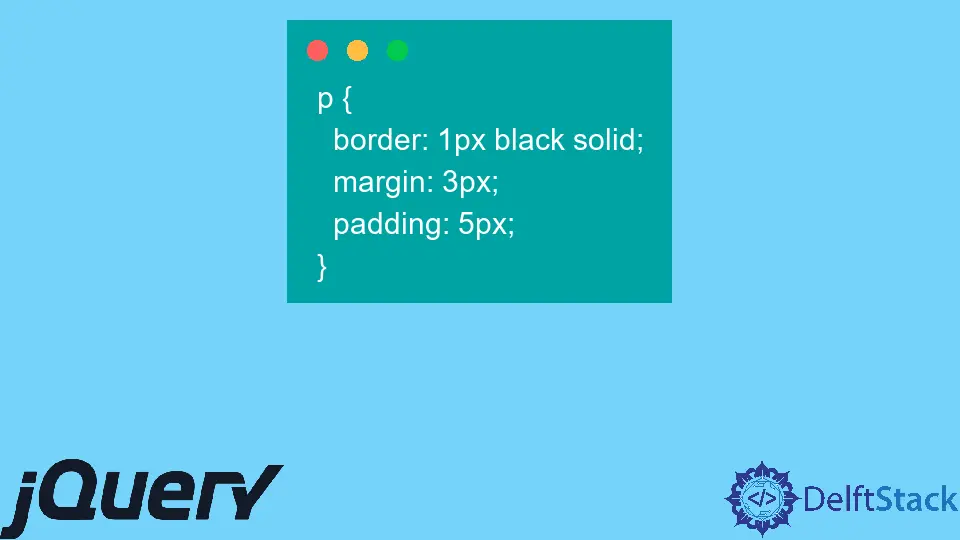
In this post, we will talk about the jQuery methods to get the height of the specified element.
Get Height in jQuery
jQuery provides three different methods to get height of a jQuery element, .height()
, .innerHeight()
or .outerHeight()
. Depending on the requirement, you can choose any of these methods to get the height of a jQuery element.
jQuery helps to get the current computed height for the first item in the group of matching items using these methods. Let’s first understand the difference between these methods.
.height()
- Built-in jQuery method that returns the element’s height excluding border, padding, and margin. This method doesn’t accept any input arguments..innerHeight()
- Built-in jQuery method that returns the element’s height, including the padding but excluding margin and border. This method doesn’t accept any input arguments..outerHeight()
- Built-in jQuery method that returns the height of thediv
element including border but excluding margin. This method accepts a Boolean value as an input argument, which indicates whether to include a margin or not..outerHeight(true)
- Built-in jQuery method that returns the height of thediv
including margin.
Syntax:
.height().innerHeight().outerHeight(val boolean)
The sole difference between .height()
and .css("height")
is that the former returns a pixel value with no units (e.g. 250
) while the latter returns a value with units intact (e.g. 250px
). The .height()
method is recommended when you use an element’s height in a mathematical calculation.
The .height()
always returns the content’s height, irrespective of the value of the CSS box size property. This may require getting the CSS property height plus box-sizing and then subtracting all potential borders and padding for each element if the element is box-sizing: border-box.
Use .css( "height")
instead of .height()
, to avoid this.
Let’s understand it with a simple example below.
<button id="para">Get Height</button>
<p>
Hello Users
</p>
p {
border: 1px black solid;
margin: 3px;
padding: 5px;
}
$('#para').on('click', () => {
console.log('height', $('p').height());
console.log('Inner height', $('p').innerHeight());
console.log('Outer height', $('p').outerHeight());
});
In the above example, we have defined the paragraph tag with with 1px
border, 3px
margin and 5px
padding. Once the user clicks on the button to get the height of the paragraph tag, it will get the .height()
of the p
tag, excluding border, padding, and margin.
The .innerHeight()
method will get the height of the paragraph tag with the padding, and the .outerHeight()
method will get the height of the paragraph tag with the border and margin.
Try and run the above code snippet in any browser that supports jQuery, which will display the result below.
"height", 18.4
"Inner height", 28.4
"Outer height", 30.4
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn