Resizable Element in JavaScript
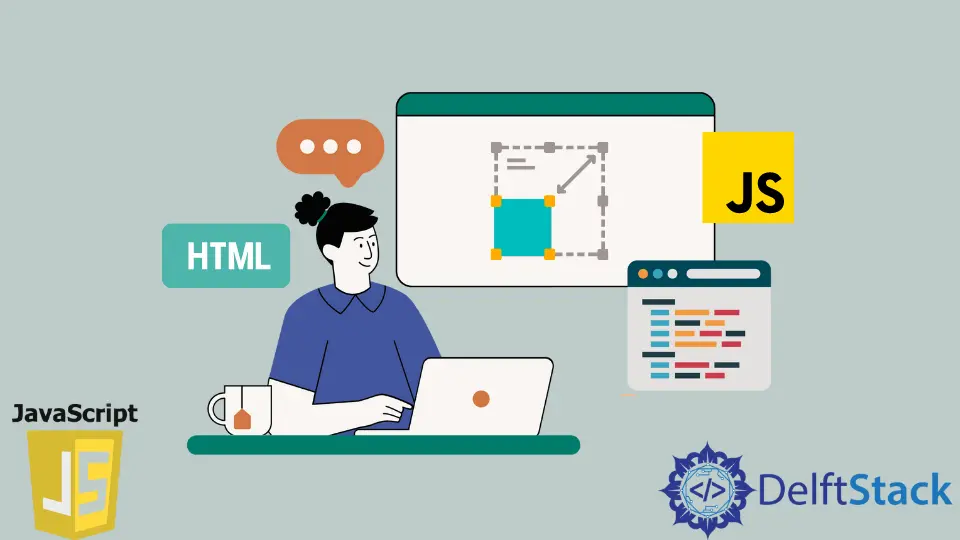
HTML
is made up of elements. The element consists of tags that define the meaning of the content of a document.
This article will show how to make resizable elements in JavaScript.
Using the Resizable Element in JavaScript
JavaScript provides addEventListener
a built-in method of the EventTarget
interface that registers an event listener. For more information, see the addEventListener()
method documentation.
We will use the addEventListener()
function to track when the user clicks on a particular element, the p
element. Once the user clicks on the p
element, the init
function is called.
The init
function appends the class name resizable
to the class name of the existing p
element. Next is to create a new div
element with the same name as the class, then append it to the target element (p
).
let p = document.querySelector('p');
p.addEventListener('click', function init() {
p.removeEventListener('click', init, false);
p.className = p.className + ' resizable';
let resizerElement = document.createElement('div');
resizerElement.className = 'resizerElement';
p.appendChild(resizerElement);
}, false);
The appendChild()
function moves the given child from its current location to the new location if it references an existing node in the document. A new event listener that listens for the mouse down event is attached to the newly created resizerElement
div element.
This mouse down event calls the initDragging
function, which calculates the horizontal (clientX
) and vertical (clientY
) coordinates of the mouse event.
resizerElement.addEventListener('mousedown', initDragging, false);
The getComputedStyle
method returns an object containing the values of all of an element’s CSS properties after applying any active style sheets and performing any basic calculations that those values may contain.
We can get the width and height of the paragraph element through this object.
let initXPoint, initYPoint, initWidth, initHeight;
function initDragging(e) {
initXPoint = e.clientX;
initYPoint = e.clientY;
initWidth = parseInt(document.defaultView.getComputedStyle(p).width, 10);
initHeight = parseInt(document.defaultView.getComputedStyle(p).height, 10);
}
After all, data is captured, the mouse move event listener is logged. When a user moves the mouse, it calculates the final height and width of the paragraph element and adds it.
Full Code Snippet:
CSS
Syntax:
p { background: lime; height: 200px; width: 300px; }
p.resizable { background: cyan; position: relative; }
p .resizer { width: 10px; height: 10px; background: blue; position:absolute; right: 0; bottom: 0; cursor: se-resize; }
JavaScript
Code:
let p = document.querySelector('p');
p.addEventListener('click', function init() {
p.removeEventListener('click', init, false);
p.className = p.className + ' resizable';
let resizer = document.createElement('div');
resizer.className = 'resizer';
p.appendChild(resizer);
resizer.addEventListener('mousedown', initDragging, false);
}, false);
let startX, startY, startWidth, startHeight;
function initDragging(e) {
startX = e.clientX;
startY = e.clientY;
startWidth = parseInt(document.defaultView.getComputedStyle(p).width, 10);
startHeight = parseInt(document.defaultView.getComputedStyle(p).height, 10);
document.documentElement.addEventListener('mousemove', doDragging, false);
document.documentElement.addEventListener('mouseup', stopDragging, false);
}
function doDragging(e) {
p.style.width = (startWidth + e.clientX - startX) + 'px';
p.style.height = (startHeight + e.clientY - startY) + 'px';
}
function stopDragging(e) {
document.documentElement.removeEventListener('mousemove', doDragging, false);
document.documentElement.removeEventListener('mouseup', stopDragging, false);
}
Output:
Click here to run the code sample.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn