How to Toggle a Button in JavaScript
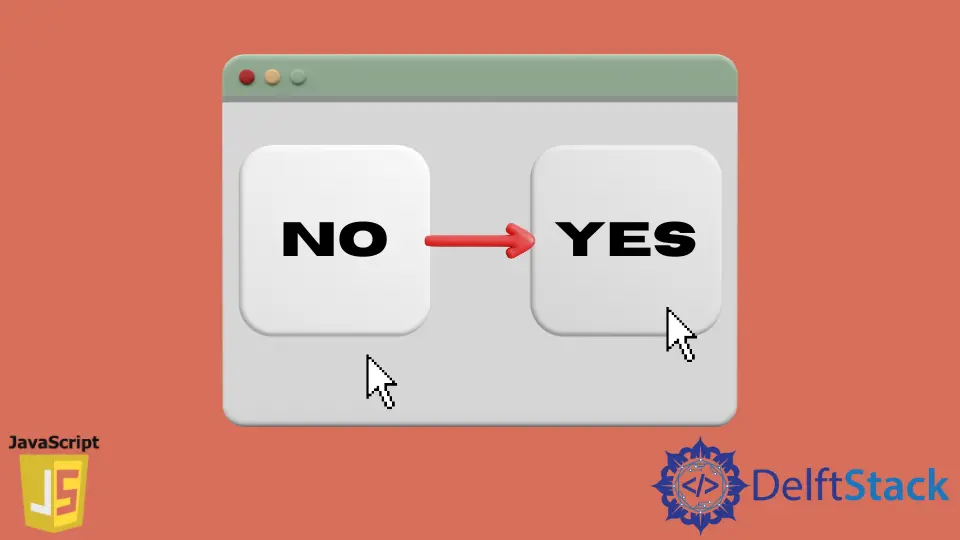
This tutorial will discuss how to toggle a button using conditional statements in JavaScript.
Toggle a Button Using Conditional Statements in JavaScript
We can toggle a button using conditional statements like if-else
statement in JavaScript. We can toggle almost all the properties of an element like its value, class, id, and color in JavaScript.
To change any property of an element, we need to get the element using its id or class. Additionally, using the property name, we can change the property value.
For example, let’s make a button and change its inner text using the if-else
statement and the value
property. See the code below.
<!DOCTYPE html>
<html>
<head></head>
<body>
<form>
<input type="button" id="myButton" value="NO"
onclick="Buttontoggle();">
</form>
</body>
<script type="text/javascript">
function Buttontoggle()
{
var t = document.getElementById("myButton");
if(t.value=="YES"){
t.value="NO";}
else if(t.value=="NO"){
t.value="YES";}
}
</script>
</html>
In the program above, we created the Buttontoggle()
function, which will be called every time the user clicks on the button. It will toggle the text inside the button.
To run this code, you need to copy this code in an HTML file and open the file with any browser, and you will see the button. In the same way, we can toggle any property of an element. We can also use a switch
statement instead of an if-else
statement. Now, let’s toggle the text using a button and if-else
statement. See the code below.
<!DOCTYPE html>
<html>
<head></head>
<body>
<button onclick="Buttontoggle()">Click to Toggle Text</button>
<div id="123">Some Text</div>
</body>
<script type="text/javascript">
function Buttontoggle()
{
var t = document.getElementById("123");
if(t.innerHTML=="Some Text"){
t.innerHTML="Toggled Text";}
else{
t.innerHTML="Some Text";}
}
</script>
</html>
In the code above, we used a button to toggle the text of another element using its id. The getElementById
property is used to get the element using its id, and the innerHTML
property is used to get the text of the div
element. We can also toggle the class of an element using the classList.toggle()
property.
For example, let’s make a text element and a style sheet where we will set some class myClass
properties like width, padding, background color, color, and font size. Now we can use the myClass
class and the classList.toggle()
property to toggle the class of the div
element. See the code below.
<!DOCTYPE html>
<html>
<head></head>
<body>
<button onclick="Buttontoggle()">Click to Toggle Class</button>
<div id="123">Some Text</div>
</body>
<style type="text/css">
.myClass {
width: 50%;
padding: 33px;
background-color: green;
color: white;
font-size: 33px;
}
</style>
<script type="text/javascript">
function Buttontoggle()
{
var t = document.getElementById("123");
t.classList.toggle("myClass");
}
</script>
</html>
In the program above, we created a button that will be used to toggle the class and a div
element whose class will be toggled. We used the style sheet to set some properties of the class, which will be applied to the text when the toggle button is clicked.