How to Change Button Text in JavaScript
- JavaScript Change Button Text on Load
- JavaScript Change Button Text on Mouseover
- JavaScript Change Button Text on Click
- JavaScript Change Button Text Using jQuery
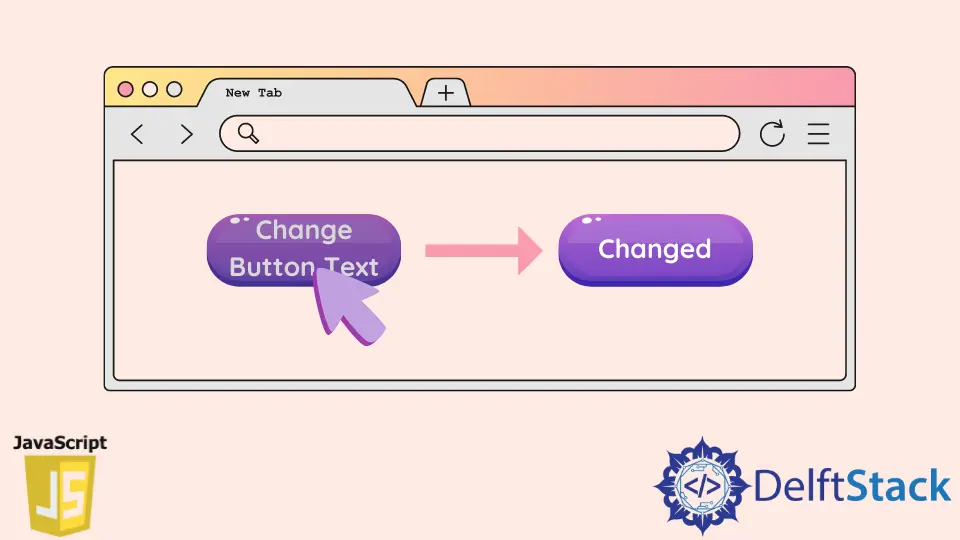
We aim to learn about JavaScript change button text via example code. It shows how the button text changes on load, click, and mouseover. It also exemplifies the use of jQuery to change the button text.
JavaScript Change Button Text on Load
If you have HTML <input>
Element like input[type='button']
or input[type='submit']
then you can change button text in the following way.
HTML Code:
<input id="btn" type="button" value="Update">
<input id="btnSubmit" type="submit" value="Update">
JavaScript Code:
document.querySelector('#btn').value = 'Remove';
document.querySelector('#btnSubmit').value = 'Remove';
You can also change button text of HTML <button>
Element by using any of given methods below (given methods are .innerHTML
, .innerText
, and .textContent
).
HTML Code:
<button id="btn" type="button" value="Show Result">Show Result</button>
<button id="btnSubmit" type="submit" value="Submit Result">Submit Result</button>
JavaScript Code:
// querySelector selects the element whose id's value is btn
document.querySelector('#btn').innerHTML = 'Hide Result';
document.querySelector('#btn').innerText = 'Hide Result';
document.querySelector('#btn').textContent = 'Hide Result';
// querySelector selects the element whose id's value is btnSubmit
document.querySelector('#btnSubmit').innerHTML = 'Hide Result';
document.querySelector('#btnSubmit').innerText = 'Hide Result';
document.querySelector('#btnSubmit').textContent = 'Hide Result';
Can we use .innerHTML
, .innerText
, and .textContent
for HTML <input>
element? No. The reason is <input>
is an empty element while <button>
is a container tag and has .innerHTML
, .innerText
, and .textContent
properties.
Though the goal is achieved by using .innerHTML
, .innerText
, and .textContent
, you must have certain points in your mind.
- You may have to face cross-site security attacks due to using JavaScript
.innerHTML
. - JavaScript
.innerText
reduces the performance because it needs details about the layout system. - JavaScript
.textContent
does not arises any security concerns like.innerHTML
. It also does not have to parse the HTML Content like.innerText
which results in the best performance.
Now, you know the differences between them. So, choose any of these methods that suit your project requirements. You can read more about them here.
JavaScript Change Button Text on Mouseover
HTML Code:
<button class="button">Hide Result</button>
CSS Code:
.button {
background-color: red;
}
.button:hover {
background-color: green;
}
JavaScript Code:
let btn = document.querySelector('.button');
btn.addEventListener('mouseover', function() {
this.textContent = 'Show Result!';
})
btn.addEventListener('mouseout', function() {
this.textContent = 'Hide Result';
})
The code above should show an output where the button’s text and color change when your mouse pointer hovers the button.
The querySelector()
outputs the first element that matches the defined selector. The addEventListener()
attaches an event handler to the given element and sets up a method for triggering a particular event.
We use mouseover
and mouseout
events, and the .textContent
changes the button text.
JavaScript Change Button Text on Click
HTML Code:
<input onclick="changeText()" type="button" value="Hide Result" id="btn">
JavaScript Code:
function changeText() {
let element = document.getElementById('btn');
if (element.value == 'Hide Result')
element.value = 'Show Result';
else
element.value = 'Hide Result';
}
changeText()
runs when you click on the button. This method gets the first element that matches the specified selector using getElementById()
. Then, it checks the element’s value and changes according to the if-else
statement.
JavaScript Change Button Text Using jQuery
HTML Code:
<!DOCTYPE>
<html>
<head>
<title>Change Text</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js"></script>
</head>
<body>
<button id="button" onclick="changeText()">Hide Result</button>
</body>
</html>
JavaScript Code:
function changeText() {
$('#button').html('Show Result');
$('#button').css('background-color', 'green');
}
The code above changes the button’s text from Hide Result
to Show Result
when you click on the button, and it also changes the button’s color to green.
The .html()
sets the content of the selected element while .css()
changes the background-color
to green. Remember, .html()
is used to for HTML <button>
element.
For more detail of these functions, check this link.
You might be thinking about how can we change the text using jQuery if we have HTML <input>
element? The following code is for you to understand.
HTML Code:
<!DOCTYPE>
<html>
<head>
<title>Change Text</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js"></script>
</head>
<body>
<input type="button" id="btnShow" value="Show" onclick="changeText()">
</body>
</html>
JavaScript Code:
function changeText() {
$('#btnShow').attr('value', 'Hide'); // versions older than 1.6
$('#btnShow').prop('value', 'Hide'); // versions newer than 1.6
$('#btnShow').css('background-color', 'yellow');
}
You can use .attr()
or prop()
(depending on jQuery version) to change the button text of HTML <input>
element. Both, .attr()
and .prop()
targets the value
attribute of <input>
element and changes its value according to the second parameter.
In this sample code, the second parameter is Hide
. The changeText()
method also changes the background color to yellow using .css()
function.