How to Create Back Button in Web Page Using JavaScript
- The Go Back Button in HTML
- Create a Back Button in HTML by Using JavaScript
-
Use the
history.back()
Method to Create the Back Button in JavaScript -
Use the
history.go()
Method to Create the Back Button in JavaScript
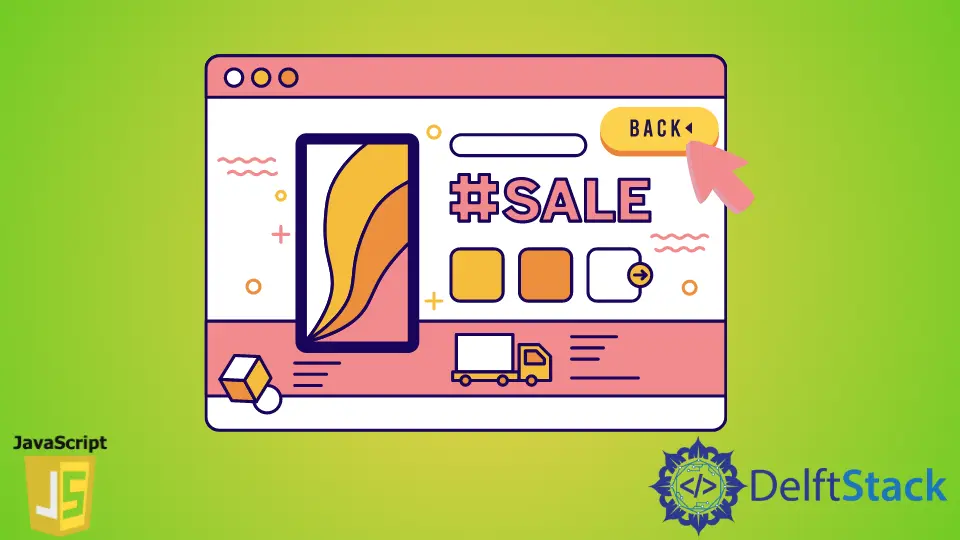
In this JavaScript article, we’ll learn how to create a back button using JavaScript and the need and use of the back button in HTML. We will see how to get the previous page by using the built-in methods of JavaScript.
The Go Back Button in HTML
The browsers we use already have back buttons, so you must have a better reason for needing to put the go back button
on your page. We can add a back button
on a web page using HTML or JavaScript code.
The web page will have a button or a link, and by clicking it, the browser return to the previous page. This can be done using HTML code and a little JavaScript on the client-side.
Create a Back Button in HTML by Using JavaScript
The code for creating an HTML back button
can be placed anywhere inside the page. The back button
we create will work just like the back button in the toolbar of our browser.
Remember that this back button
will not work if the user has no browsing history. Nothing will happen if the user opens the web page and clicks on the back button
.
Use the history.back()
Method to Create the Back Button in JavaScript
We have a built-in JavaScript object called history in web browsers, which contains all the URLs a user has visited in the current browser window. We can use this history.back()
method to tell the web browser to go back to the user’s previous page. To use this built-in JavaScript object is to add it to the onclick event attribute of a button. We create the button using the <form>
element, which contains the button type’s <input>
element, as we see in the following code.
Code - HTML:
<!DOCTYPE html>
<html>
<head>
<title>Back Button</title>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<h3 class="title">Creating Back Button </h3>
<form>
<input type="button" value="Go back!" onclick="history.back()">
</form>
</body>
</html>
Output:
If a user clicks the button, the user goes back to the previous page in the browser.
Use the history.go()
Method to Create the Back Button in JavaScript
If we want to go back to the specific page in the browser, we use the history.go()
method. This built-in JavaScript method tells the browser to go to a specific page in the browsing history.
We can give any specific history by putting a number inside the parentheses, which we call an argument in programming. For example, by giving the number -1
as an argument in the parentheses, the browser goes back one page in the browser’s history.
In the following code, we used history.go(-1)
method instead of history.back()
method. We can even ask the browser to go more than 1 step back or forward by giving the number -2
as an argument in the parentheses like this history.go(-2)
.
Code - HTML:
<!DOCTYPE html>
<html>
<head>
<title>Back Button</title>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<h3 class="title">Creating Back Button </h3>
<form>
<INPUT TYPE="button" VALUE="Go one step back" onClick="history.go(-1);">
</form>
<br>
<form>
<INPUT TYPE="button" VALUE="Go two steps back" onClick="history.go(-2);">
</form>
</body>
</html>
Output:
From the results in the above image, we have two buttons. When a user clicks on the first button, the browser goes back one page in the browser’s history, and if the user clicks on the second button, the browser will go back two pages in the browser’s user history.
The main difference between the history.back()
and history.go()
method is that the back()
only goes back one page, but go()
goes back and forward the number of pages we pass as a parameter in the parentheses relative to our current web page.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn