How to Hide Button in JavaScript
- Use Visibility Property to Hide Button in JavaScript
- Use Display Property to Hide Button in JavaScript
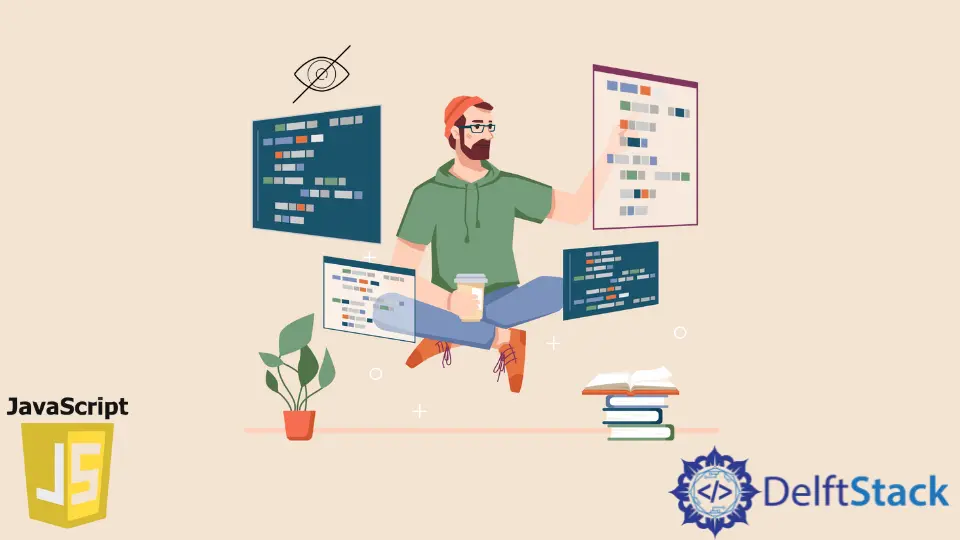
In today’s post, we’ll learn about hiding buttons in JavaScript.
Use Visibility Property to Hide Button in JavaScript
The CSS property visibility
shows or hides an element without affecting the layout of a document.
Syntax:
visibility: hidden;
The element box is invisible but affects the layout as usual. The element’s descendants are visible if their visibility is set to visible
.
Using a hidden
visibility value for an element removes it from the accessibility tree. As a result, the screen reader technology no longer announces the element and all of its children.
Visibility values are toggled between visible
and invisible
. Therefore, one of the start or end values must be visible, or no interpolation can be performed.
The value is interpolated as a discrete step, assigning time-function values between 0 and 1 to the visible endpoint and other time-function values to the nearest endpoint.
You can find more information about the property in the Visibility
documentation.
Let’s take an example of showing and hiding the button in JavaScript using the visibility
property.
<input type="button" id="btn-1" value="Show" onClick="hideAction()" />
<input type="button" id="btn-2" value="Hide" onClick="hideAction()"/>
let hidden = false;
function hideAction() {
hidden = !hidden;
if (hidden) {
document.getElementById('btn-1').style.visibility = 'hidden';
} else {
document.getElementById('btn-1').style.visibility = 'visible';
}
}
We have defined two buttons in the example above. The first button will be shown and hidden based on the toggle button value.
The second button will toggle the visibility of the previous button.
Run the code snippet above in any browser that supports JavaScript; it will show the below result.
Output:
Hide Button:
Use Display Property to Hide Button in JavaScript
The CSS display
property determines whether an element is treated as a block or inline element and the layout used for its child elements, e.g., B. Flow, Grid, or Flex layout.
Syntax:
display: block;
display: none;
The Display
property specifies an element’s interior and exterior display types. The external type determines an element’s participation in the flow layout; the inner type sets the child’s design.
Some display values are fully defined in their specifications. For example, what happens when the display: flex
is declared is defined in the CSS flexbox model specification.
You can find more information about the property in the Display
documentation.
Let’s take an example of showing and hiding the button in JavaScript using the display
property.
<input type="button" id="btn-3" value="Show" onClick="displayAction()" />
<input type="button" id="btn-4" value="Toggle" onClick="displayAction()"/>
function displayAction() {
hidden = !hidden;
if (hidden) {
document.getElementById('btn-3').style.display = 'none';
} else {
document.getElementById('btn-3').style.display = 'block';
}
}
We have defined two buttons in the example above. The first button will be shown and hidden based on the toggle button value.
The second button will toggle the display
property of the previous button.
Run the code snippet above in any browser that supports JavaScript; it will show the below result.
Output:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn