How to Disable Button Click in JavaScript
-
Use JavaScript
disabled
Property to Disable Button Click -
Use jQuery
disabled
Attribute to Disable a Button Click
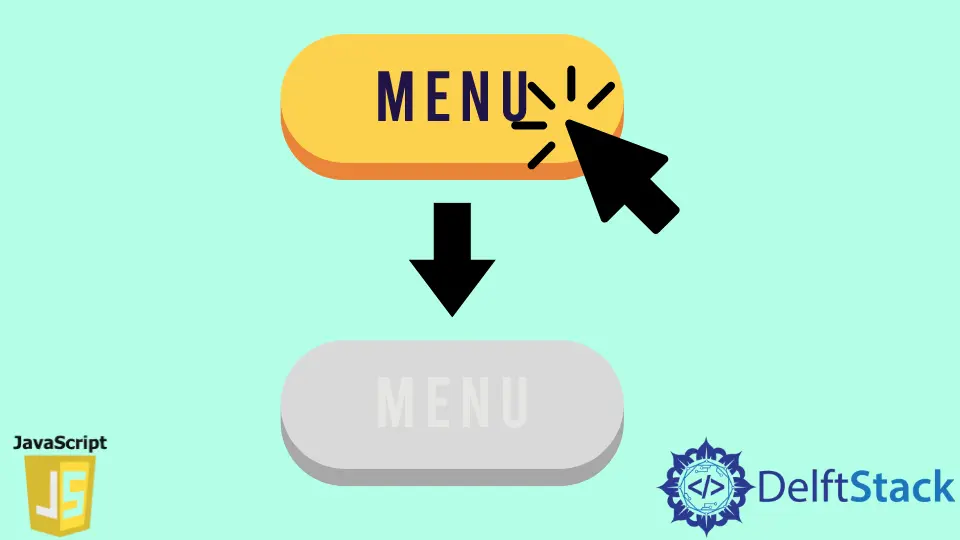
In JavaScript, the disabled
property only works fine for the buttons rooted as type="submit"
. Otherwise, it will not function like a "one submit button"
; rather, it will accept multiple submissions.
Similarly, the jQuery attribute disabled
works for the button with a submit
type. Also, the form submission method is very important in this regard.
In our example sets, we will see the implementation of the JavaScript disabled
property and jQuery disabled
attribute.
Use JavaScript disabled
Property to Disable Button Click
Generally, we define a form and its method to ensure the purpose of the submissions. Regardless, JavaScript’s property disabled
lets us make a button inactive.
Here, we will initiate an input
field denoting the type submit
. Later taking an instance of the input
tag, we will fire the disabled
property.
Code Snippet:
<body>
<p>Click the button to submit data!</p>
<p>
<input type='submit' value='Submit' id='btClickMe'
onclick='save();'>
</p>
<p id="msg"></p>
</body>
<script>
function save() {
var dis = document.getElementById('btClickMe').disabled = true;
var msg = document.getElementById('msg');
msg.innerHTML = 'Data submitted and the button disabled ☺';
}
</script>
</html>
Output:
Use jQuery disabled
Attribute to Disable a Button Click
In jQuery, we will have a similar use case of the disabled
attribute. The difference here is we will initiate the input
field (submit
type button) inside a form
tag.
We will ensure if that works inside the form as well.
Code Snippet:
<body>
<form id="fABC" action="#" method="POST">
<input type="submit" id="btnSubmit" value="Submit">
</form>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
<input type="button" value="i am normal button" id="btnTest">
<script>
$(document).ready(function () {
$("#fABC").submit(function (e) {
e.preventDefault();
$("#btnSubmit").attr("disabled", true);
$("#btnTest").attr("disabled", true);
return true;
});
});
</script>
</body>
</html>
Output:
The jQuery way of disabling a button is similar to JavaScript. In the example, we have added a general button with the type=button
, which cannot be handled by the disabled
attribute or property.
This is how the JavaScript or jQuery disabled
property works to inactive a submit button.