How to Sort HTML Table in JavaScript
- Understanding the HTML Table Structure
- Method 1: Sorting by Column Header Click
- Method 2: Sorting with a Button Click
- Conclusion
- FAQ
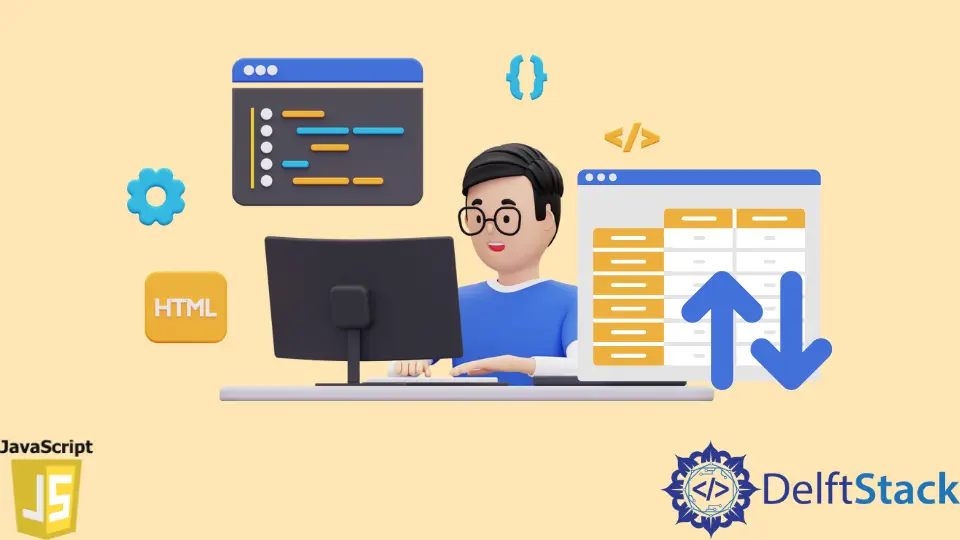
Sorting an HTML table can significantly enhance the user experience by allowing users to view data in a more organized manner. While there are various methods to achieve this, using JavaScript is one of the most effective ways.
In this tutorial, we will delve into different approaches to sorting HTML tables using JavaScript. Whether you’re a beginner or an experienced developer, this guide will provide you with clear, step-by-step instructions and practical examples. By the end, you’ll have the skills to implement table sorting functionality in your web projects effortlessly.
Understanding the HTML Table Structure
Before diving into sorting, let’s first understand the basic structure of an HTML table. An HTML table consists of rows and columns, defined with the <table>
, <tr>
, <th>
, and <td>
tags. Here’s a simple example of an HTML table:
<table id="myTable">
<thead>
<tr>
<th>Name</th>
<th>Age</th>
<th>Country</th>
</tr>
</thead>
<tbody>
<tr>
<td>John</td>
<td>25</td>
<td>USA</td>
</tr>
<tr>
<td>Alice</td>
<td>30</td>
<td>UK</td>
</tr>
<tr>
<td>Mike</td>
<td>22</td>
<td>Canada</td>
</tr>
</tbody>
</table>
This table contains three columns: Name, Age, and Country. Now, let’s explore how to sort this table using JavaScript.
Method 1: Sorting by Column Header Click
One of the most user-friendly ways to sort an HTML table is by allowing users to click on the column headers. This method is straightforward and intuitive. Below is the JavaScript code that enables sorting when a header is clicked.
document.querySelectorAll('th').forEach((header, index) => {
header.addEventListener('click', () => {
const tableBody = document.querySelector('#myTable tbody');
const rows = Array.from(tableBody.querySelectorAll('tr'));
const isAscending = header.classList.contains('asc');
rows.sort((a, b) => {
const aText = a.children[index].textContent.trim();
const bText = b.children[index].textContent.trim();
return isAscending
? aText.localeCompare(bText)
: bText.localeCompare(aText);
});
rows.forEach(row => tableBody.appendChild(row));
header.classList.toggle('asc', !isAscending);
});
});
This code adds an event listener to each header cell. When a header is clicked, it retrieves all rows from the table body and sorts them based on the clicked column. The localeCompare
method is used for string comparison, ensuring that sorting works for text data. The sorted rows are then appended back to the table body, effectively reordering them.
Output:
Rows sorted in ascending or descending order based on the clicked header.
This method is efficient and enhances user interaction with the table. By toggling the sorting order with each click, users can easily switch between ascending and descending views.
Method 2: Sorting with a Button Click
Another effective way to sort an HTML table is by using a button. This method is particularly useful when you want to provide users with a specific sorting option rather than relying on header clicks. Below is an example of how to implement this.
document.getElementById('sortButton').addEventListener('click', () => {
const tableBody = document.querySelector('#myTable tbody');
const rows = Array.from(tableBody.querySelectorAll('tr'));
rows.sort((a, b) => {
const aAge = parseInt(a.children[1].textContent.trim());
const bAge = parseInt(b.children[1].textContent.trim());
return aAge - bAge;
});
rows.forEach(row => tableBody.appendChild(row));
});
In this code, we add an event listener to a button with the ID sortButton
. When the button is clicked, the rows are sorted based on the age column (the second column). We convert the text content of each age cell to an integer using parseInt
for accurate numerical comparison. The sorted rows are then appended back to the table body.
Output:
Rows sorted by age in ascending order.
This method is highly customizable, allowing you to sort based on any criteria you choose. You can easily modify the sorting logic to sort by different columns or in different orders.
Conclusion
Sorting HTML tables using JavaScript is a straightforward process that can greatly enhance the usability of your web applications. Whether you choose to implement sorting through header clicks or button clicks, both methods provide a seamless experience for users. With the examples provided, you can easily integrate sorting functionality into your projects, making data presentation more accessible and engaging. As you become more familiar with JavaScript, you can explore additional features, such as multi-column sorting or integrating sorting libraries for more complex scenarios.
FAQ
-
How do I sort a table numerically in JavaScript?
You can sort a table numerically by parsing the text content of the cells into numbers using functions likeparseInt
orparseFloat
and then comparing them during the sort. -
Can I sort multiple columns at once?
Yes, you can implement multi-column sorting by modifying the sorting logic to compare multiple criteria. You’ll need to adjust the sort function accordingly. -
Is it possible to add a sorting animation?
Yes, you can add animations using CSS transitions or JavaScript libraries to create a more visually appealing sorting experience.
-
What if my table has mixed data types?
When dealing with mixed data types, you can implement custom sorting logic to handle different types appropriately, ensuring that the sorting behaves as expected. -
Are there any libraries that simplify table sorting?
Yes, libraries like DataTables or Sortable.js provide built-in functionalities for sorting tables and can save you time on implementation.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn