JavaScript 排序 HTML 表格
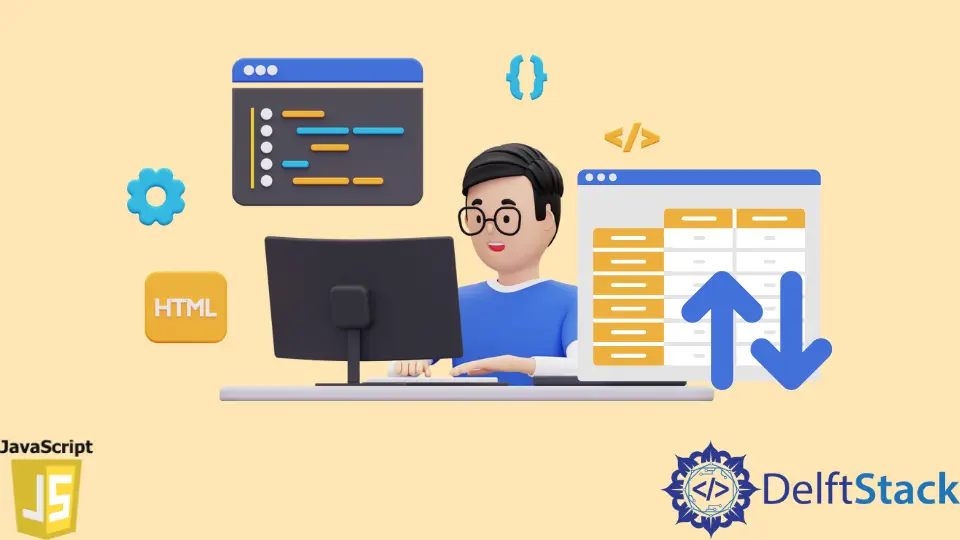
我们经常遇到希望使表格具有交互性的情况。我们可能只想通过单击列或任何其他方法来对它们进行排序。HTML 没有任何编程能力,因此我们必须使用 JavaScript 对 HTML 表进行排序。不幸的是,没有内置的方法可以帮助我们对数组进行排序。因此,我们编写了利用 DOM 操作功能和比较功能的自定义方法。本教程教授如何使用 JavaScript 对 HTML 表进行排序。
使用 JavaScript 对 HTML 表格进行排序
用户定义的方法使用以下两个辅助函数对数组进行排序。
getCellValue()
一个函数,用于从给定表格行和列索引的单元格中获取内容。
我们使用表格行的 children 属性通过 tr.children[i]
选择第 i 列,然后使用其 innerText
或 textContent
属性来获取存储的实际内容。一旦我们获得了单元格中的实际内容,我们就可以使用自定义比较器对这些值进行比较和排序。
comparer()
用于比较给定表中两个元素的值的函数。
我们首先使用具有指定行和列索引的 getCellValue()
函数获取单元格的内容。然后我们检查两者是否都是有效的整数或字符串,然后比较它们以找到正确的顺序。
算法
-
通过向表中添加事件侦听器来使表中的所有标题都可点击。
-
查找所选列中除第一行之外的所有行。
-
使用 JavaScript 的
sort()
函数和comparer()
对元素进行排序。 -
将排序后的行插入回表中以获取最终排序表作为输出。
代码
const getCellValue = (tableRow, columnIndex) =>
tableRow.children[columnIndex].innerText ||
tableRow.children[columnIndex].textContent;
const comparer = (idx, asc) => (r1, r2) =>
((el1, el2) => el1 !== '' && el2 !== '' && !isNaN(el1) && !isNaN(el2) ?
el1 - el2 :
el1.toString().localeCompare(el2))(
getCellValue(asc ? r1 : r2, idx), getCellValue(asc ? r2 : r1, idx));
// do the work...
document.querySelectorAll('th').forEach(
th => th.addEventListener(
'click', (() => {
const table = th.closest('table');
Array.from(table.querySelectorAll('tr:nth-child(n+2)'))
.sort(comparer(
Array.from(th.parentNode.children).indexOf(th),
this.asc = !this.asc))
.forEach(tr => table.appendChild(tr));
})));
所有主要浏览器都支持上面讨论的所有方法。
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn