How to Hide Table Rows in JavaScript
-
Use jQuery’s
toggle()
Function to Hide Table Rows in JavaScript -
Use jQuery’s
show()
andhide()
Function to Hide Table Rows in JavaScript - Conclusion
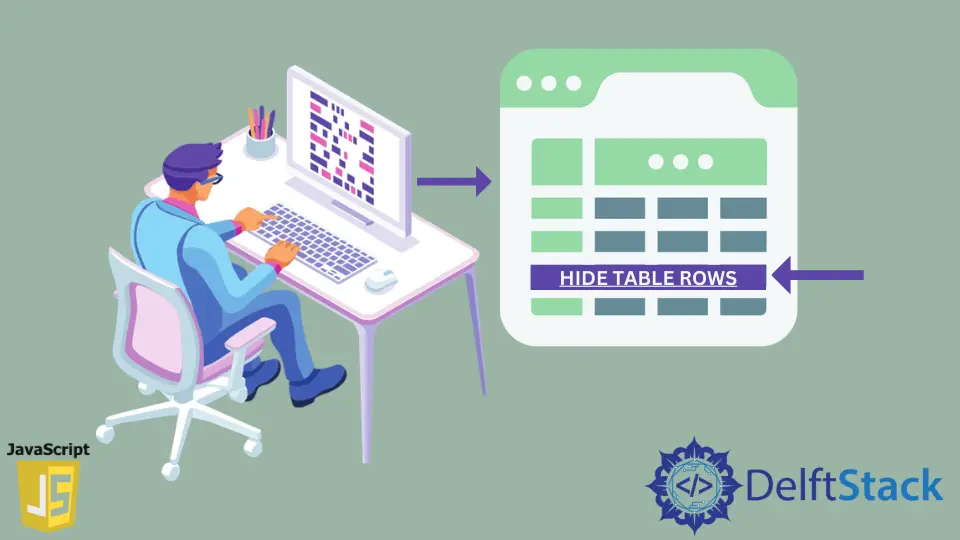
Tables are a fundamental component in web development, often employed to organize and display data in a structured format. However, there are instances where it’s necessary to hide specific table contents or rows dynamically using JavaScript.
This dynamic hiding and showing of table elements can be particularly valuable for creating interactive interfaces and optimizing the information display based on user actions.
In JavaScript, a practical approach involves categorizing specific table data under a common class
.
This class
is associated with a function that dictates the behavior of the table rows when certain triggers, like a button or link tag, activate the function. The function, in turn, manages the hiding and displaying of table rows.
In this article, we will explore how to achieve this functionality using jQuery’s toggle()
function, as well as the show()
and hide()
functions. Both methods allow for dynamic expansion and contraction of rows.
Use jQuery’s toggle()
Function to Hide Table Rows in JavaScript
The toggle()
function in jQuery is a versatile method used to toggle the visibility of elements. It allows you to alternate between hiding and showing elements with a smooth animation or simply toggling their display on and off.
In the example below, we’ll demonstrate how to use toggle()
to toggle the display of table rows when a specific <a>
tag is clicked. When the <a>
tag with the class toggler
is clicked, the corresponding table rows with a specific category class will toggle between being displayed and hidden.
Let’s delve into the code and understand how this works.
HTML Markup:
<table>
<tr>
<td>Oranges</td>
<td>100</td>
<td><a href="#" class="toggler" data-prod-cat="1">Toggle Category 1</a></td>
</tr>
<tr class="cat1" style="display:none">
<td></td>
<td>120</td>
</tr>
<tr class="cat1" style="display:none">
<td></td>
<td>140</td>
</tr>
<tr>
<td>Apples</td>
<td>100</td>
<td><a href="#" class="toggler" data-prod-cat="2">Toggle Category 2</a></td>
</tr>
<tr class="cat2" style="display:none">
<td></td>
<td>120</td>
</tr>
<tr class="cat2" style="display:none">
<td></td>
<td>140</td>
</tr>
</table>
In this markup, there’s an HTML table with rows representing different categories of products: Category 1
and Category 2
. Each category has a toggle link (<a>
tag with the class toggler
) associated with it and a unique data-prod-cat
attribute indicating the category number (1 or 2).
Note that, initially, the rows for each category are hidden (display: none
) using the style
attribute.
When this link is clicked, the corresponding rows for that category should toggle their display between being visible and hidden.
JavaScript:
$(document).ready(function(){
$(".toggler").click(function(e){
e.preventDefault();
$('.cat'+$(this).attr('data-prod-cat')).toggle();
});
});
In this JavaScript code, $(document).ready(function(){...})
ensures that the code inside it executes after the document (the HTML) has been fully loaded. The $(".toggler").click(function(e){...})
sets up a click event listener for elements with the class toggler
(the toggle links).
When a toggle link is clicked, e.preventDefault()
prevents the default behavior of the anchor, which is navigating to a link. The $('.cat'+$(this).attr('data-prod-cat')).toggle()
toggles the display of rows based on the clicked link’s associated category.
The $(this).attr('data-prod-cat')
retrieves the category number from the data-prod-cat
attribute of the clicked link, and the $('.cat'+$(this).attr('data-prod-cat')).toggle()
toggles the visibility of the rows belonging to that category.
Overall, the toggle()
function is crucial in dynamically showing and hiding specific table rows based on the category chosen by the user through the toggle links. It provides a seamless user experience when interacting with the table data.
Complete code snippet:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Toggle Table Rows with jQuery</title>
<script src="https://code.jquery.com/jquery-3.1.0.js"></script>
</head>
<body>
<table>
<tr>
<td>Oranges</td>
<td>100</td>
<td><a href="#" class="toggler" data-prod-cat="1">Toggle Category 1</a></td>
</tr>
<tr class="cat1" style="display:none">
<td></td>
<td>120</td>
</tr>
<tr class="cat1" style="display:none">
<td></td>
<td>140</td>
</tr>
<tr>
<td>Apples</td>
<td>100</td>
<td><a href="#" class="toggler" data-prod-cat="2">Toggle Category 2</a></td>
</tr>
<tr class="cat2" style="display:none">
<td></td>
<td>120</td>
</tr>
<tr class="cat2" style="display:none">
<td></td>
<td>140</td>
</tr>
</table>
<script src="https://code.jquery.com/jquery-3.1.0.js"></script>
<script>
$(document).ready(function(){
$(".toggler").click(function(e){
e.preventDefault();
$('.cat'+$(this).attr('data-prod-cat')).toggle();
});
});
</script>
</body>
</html>
Output:
Overall, the toggle()
function is crucial in dynamically showing and hiding specific table rows based on the category chosen by the user through the toggle links. It provides a seamless user experience when interacting with the table data.
Use jQuery’s show()
and hide()
Function to Hide Table Rows in JavaScript
Here, we’ll delve into using jQuery’s show()
and hide()
functions to toggle the visibility of table rows using two clickable elements: one to show and one to hide the rows. Unlike the previous example, we’ll provide separate functionalities for showing and hiding the rows.
The show()
function in jQuery is used to display elements that are hidden. When invoked on a set of elements, it sets their CSS display
property to the default value, typically either block
or inline
, making them visible on the web page.
In the provided code below, you will see that when the Show Rows
button is clicked ($("#show").click(...)
), the show()
function is called on all elements with the class cat1
, making them visible (display: block
) on the webpage. Here’s the relevant portion of the code:
$("#show").click(function(){
$(".cat1").show();
});
On the other hand, the hide()
function in jQuery is used to hide elements by setting their CSS display
property to none
, making them invisible on the web page.
In the given code, when the Hide Rows
button is clicked ($("#hide").click(...)
), the hide()
function is invoked on all elements with the class cat1
, hiding them from view. Here’s the relevant portion of the code:
$("#hide").click(function(){
$(".cat1").hide();
});
By using these two functions, we can create interactive user experiences where certain elements, in this case, table rows with the class cat1
, can be shown or hidden based on user actions.
Let’s check the code block.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#hide").click(function(){
$(".cat1").hide();
});
$("#show").click(function(){
$(".cat1").show();
});
});
</script>
</head>
<body>
<table>
<tr>
<td>Oranges</td>
<td>100</td>
</tr>
<tr class="cat1" style="display:none">
<td></td>
<td>120</td>
</tr>
<tr class="cat1" style="display:none">
<td></td>
<td>140</td>
</tr>
</table>
<button id="hide">Hide</button>
<button id="show">Show</button>
</body>
</html>
As we can see, the <body>
section contains a table with three rows, each representing a type of fruit and its quantity. Two of the rows belong to the cat1
class and are initially set to be hidden (display:none
).
There are also two buttons, one to hide and one to show the hidden rows.
Now, within the <script>
tag, the $(document).ready()
function ensures that the DOM is fully loaded before executing the code inside it. This is a good practice to ensure that the elements you’re working with are available and ready for manipulation.
The jQuery function $("#hide").click(function(){ ... });
binds a click event to the element with the id
attribute "hide"
(the Hide
button). When this button is clicked, the function inside is triggered.
It uses the $(".cat1").hide()
function to hide all elements with the class cat1
(the rows for fruits in this case).
On the other hand, the jQuery function $("#show").click(function(){ ... });
binds a click event to the element with the id
attribute "show"
(the Show
button). When this button is clicked, the function inside is triggered.
It uses the $(".cat1").show()
function to make all elements with the class cat1
visible again.
Output:
As we can see in the output, when the Hide
button is clicked, rows with the class cat1
are hidden. Conversely, clicking the Show
button makes these rows visible again.
This functionality enhances user interaction, allowing for a more streamlined display of information in the table.
Conclusion
In this article, we have explored two effective approaches: utilizing the toggle()
function provided by jQuery and the show()
and hide()
functions.
The toggle()
function, a powerful feature of jQuery, allows for a seamless toggle between visibility states. When applied to table elements, it enables a smooth transition between displaying and hiding, enhancing interactivity and user engagement.
On the other hand, the show()
and hide()
functions provide a straightforward way to make elements visible or hide them instantly. These functions are particularly useful for cases where you want to control the display of specific table contents or rows based on user actions.