How to Create A Lookup Table in JavaScript
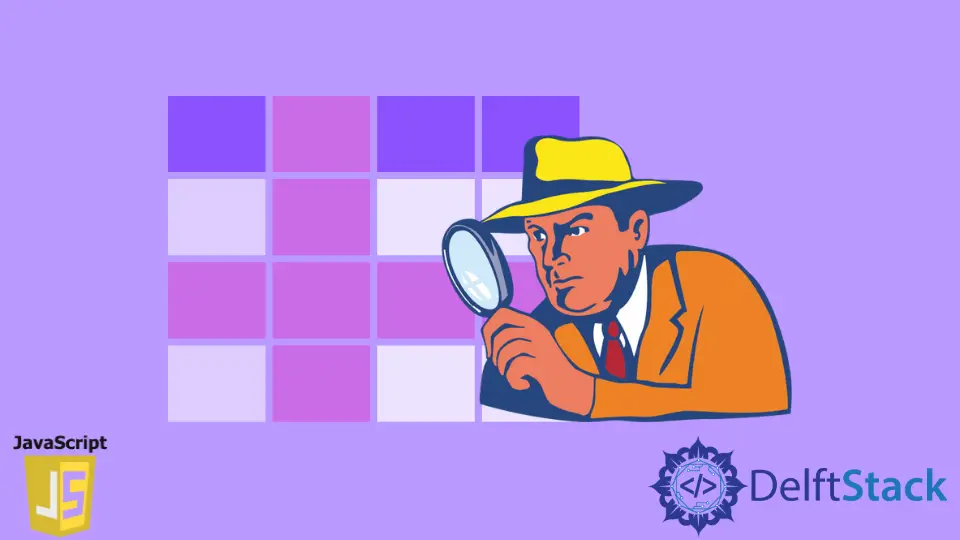
In today’s post, we’ll learn to create a lookup table using a simple way in JavaScript.
A Lookup Table in JavaScript
A lookup table is an array replacing runtime computation with a simpler array indexing operation in the data processing. The process is called direct addressing. JavaScript uses objects for lookup functions.
This is the famous algorithm used to increase the performance of search result queries. Let’s understand it with the following example.
Suppose you run an e-commerce website whose main function is to list products. Based on user-selected filters, display the price of a product.
Querying this output decreases performance each time the server is accessed. You can index the products based on the most used filters to solve this problem.
const DBProductArray = [
{Color: 'Red', Size: 'Small', Price: '$450'},
{Color: 'Red', Size: 'Medium', Price: '$460'},
{Color: 'Red', Size: 'Large', Price: '$460'},
{Color: 'Red', Size: 'Extra-Large', Price: '$470'},
{Color: 'White', Size: 'Small', Price: '$650'},
{Color: 'White', Size: 'Medium', Price: '$660'},
{Color: 'White', Size: 'Large', Price: '$670'},
{Color: 'White', Size: 'Extra-Large', Price: '$680'}
];
const lookupMap = {};
We have a DBProductArray
in the example above due to the server’s List API operation. This array contains product information such as product Color
, Size
, and Price
.
Depending on the filters chosen by the end-user, we need to show the Price
information. To speed up the search, we’ll create a lookup table that has Color
and Size
information as the key
and Price
information as the value
.
const arrayLength = DBProductArray.length;
for (i = 0; i < arrayLength; i++) {
var record = DBProductArray[i];
if (typeof lookupMap[record.Color] === 'undefined')
lookupMap[record.Color] = {};
lookupMap[record.Color][record.Size] = record.Price;
}
console.log(lookupMap);
Output:
{
Red: {
Extra-Large: "$470",
Large: "$460",
Medium: "$460",
Small: "$450"
},
White: {
Extra-Large: "$680",
Large: "$670",
Medium: "$660",
Small: "$650"
}
}
The code above loop through the DBProductArray
and check if the color information is there. We create the color as a key for the nested objects if it exists.
The nested object contains size
as the key and price
as the value. You can now view pricing information for selected products without querying the database, saving response time and speeding up performance.
const selectedColor = 'White';
const selectedSize = 'Large';
console.log(lookupMap[selectedColor][selectedSize]);
Try to run the above code snippet in any browser that supports JavaScript; it will show the below result.
Output:
"$670"
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn