How to Clear Table in JavaScript
- What is a Table in HTML
-
Use
replaceChild()
to Clear Table in JavaScript -
Use
getElementById()
to Clear Table in JavaScript
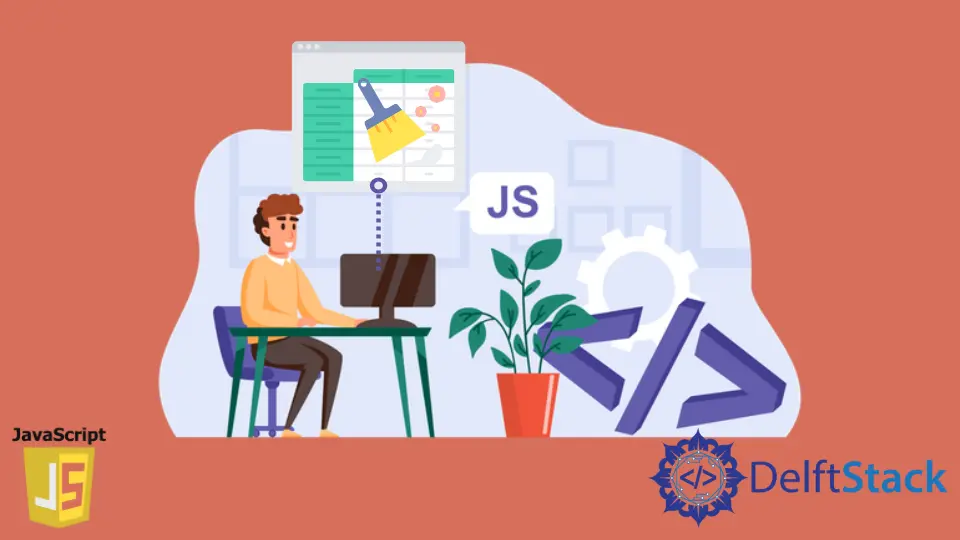
This article shows how to clear an HTML table using pure JavaScript.
What is a Table in HTML
A table is a structured data set of rows and columns (table data). It looks more like a spreadsheet.
In HTML, using tables, you can organize data such as images, text, links, etc., in rows and columns of cells.
Using tables on the web has recently become more popular thanks to HTML table tags that make it easier to create and design.
An HTML table is defined by the <table>
tag. This is the most important tag, which is the main container of the table.
Each row of the table is defined by the <tr>
tag. The <th>
tag defines the head of a table.
Table headings are by default bold and centered. The cell is defined with the <td>
tag.
Use replaceChild()
to Clear Table in JavaScript
The replaceChild()
method of the Node element replaces a child node within the specified (parent) node. This function takes two arguments, newChild
and oldChild
.
Syntax:
replaceChild(newChild, oldChild);
The newChild
is the new node used to replace the oldChild
. If the new node already exists elsewhere in the DOM, it is removed from that location first.
An oldChild
is the child element that is to be replaced. You can find more information in the documentation for replaceChild()
.
So let’s say we have users along with email and names. We want to find out users whose email ends with gmail.com
.
We can create search input, where we can enter the search query.
<button onclick="clearTable()">Clear table</button>
<table id="userTable">
<tbody id="tableBody">
<tr class="header">
<th style="width:60%;">Name</th>
<th style="width:40%;">Email</th>
</tr>
<tr>
<td>Alfreds Futterkiste</td>
<td>alfreds@example.com</td>
</tr>
<tr>
<td>Berglunds snabbkop</td>
<td>snabbkop@gmail.com</td>
</tr>
<tr>
<td>John Doe</td>
<td>john@dummy.com</td>
</tr>
<tr>
<td>Magazzini</td>
<td>magazzini@gmail.com</td>
</tr>
</tbody>
</table>
Now, let’s extract the table body using getElementById()
.
function replaceTable() {
const old_tbody = document.getElementById('tableBody')
const new_tbody = document.createElement('tbody');
old_tbody.parentNode.replaceChild(new_tbody, old_tbody)
}
In the replaceTable
function, we use getElementById
to find the table body present inside the DOM. The next step is to create the new empty tbody
element.
Replace the old tbody
node with the new tbody
node.
Now let’s run the above code and click on the Clear table
button. It will clear the table and looks something like this.
Output:
Use getElementById()
to Clear Table in JavaScript
The getElementById()
is an integrated document method provided by JavaScript that returns the element object whose id matches the specified id.
Syntax:
getElementById($id)
The $id
is a mandatory parameter that specifies the id of an HTML attribute that must match. It returns the corresponding DOM element object if the corresponding element is found; otherwise, it returns null.
Now, let’s extract the table using getElementById()
.
function clearTable() {
console.log('clearing table')
var Table = document.getElementById('userTable');
Table.innerHTML = '';
}
In the clearTable
function, we use getElementById
to find a table present in the DOM. Once the table node is found, remove the innerHTML
with an empty string.
Now let’s run the above code and click on the Clear table
button. It will clear the table and looks something like this.
Output:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn