How to Rotate an Image With JavaScript
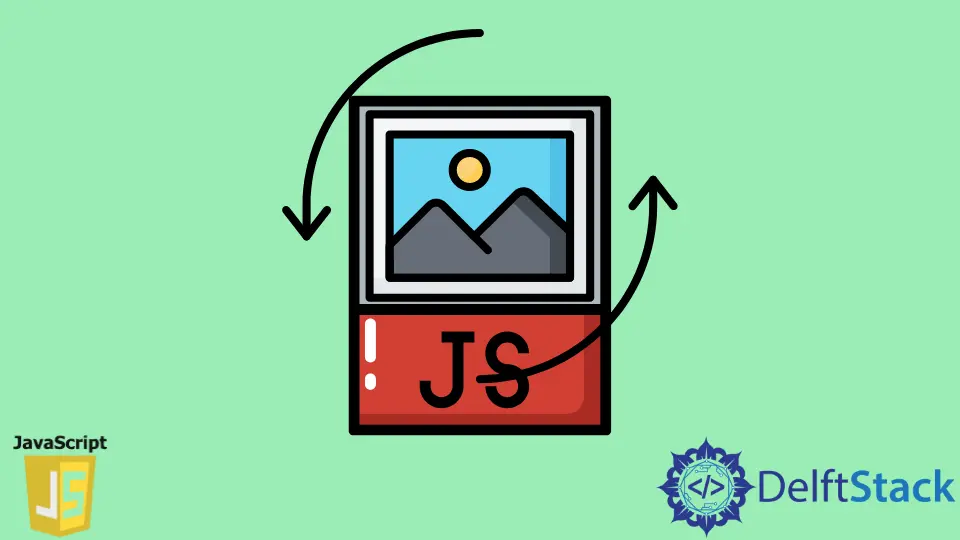
Rotating an HTML element is a common requirement that is performed using CSS. CSS provides a transform property that you can use to accomplish this task.
In this article, we will learn how to rotate images using JavaScript.
Rotate an Image With JavaScript Using CSS Transform
The CSS transform property permits you to rotate, scale, skew or move an element. Change the coordinate space of the CSS visual format model. A stacking context is created if the property has a value other than none. In this case, the element acts as a container block whose position is either set as position: fixed
or position: absolute
depending on elements that it contains.
The transform property can be specified either as a none
keyword or as one or more transform
functions. If perspective()
is one of several function values, it must appear first.
Syntax:
transform: none;
transform: translateX($Xpx) rotate($Ydeg) translateY($Zpx);
Transform values are transform-function
when one or more of the CSS transform functions are to be applied. Transform functions are multiplied from left to right. From right to left compound, transformations are effectively applied. In the syntax above, we use translateX
, which translates an element with a certain pixel horizontally, translateY
, which translates an element with a certain pixel vertically, and rotate
, which rotates an element to a certain degree around a fixed point on the 2D plane.
none
indicates that no transform should be applied. See the transform documentation for more information.
<input id="button" type="button" value="Rotate">
<div id="img_container">
<img src="https://www.google.com/images/srpr/logo3w.png" id="image">
<div>
let angle = 0, img = document.getElementById('img_container');
document.getElementById('button').onclick = function() {
angle = (angle + 90) % 360;
img.className = 'rotate' + angle;
}
#img_container.rotate90 {
width: 100px;
height: 820px
}
#image {
transform-origin: top left;
-webkit-transform-origin: top left;
}
#img_container.rotate90 #image {
transform: rotate(90deg) translateY(-100%);
-webkit-transform: rotate(90deg) translateY(-100%);
}
In the code above, we create a container, and every time the user clicks the rotate button, we add 90 degrees to the current angle and take the modulus of 360 degrees. Once the final angle is found, we can add the predefined classes whose properties have been declared using CSS.
The example only shows a 90-degree rotation, but you can define it to any angle like 45 degrees or 105 degrees and so on. The output of the code above looks like this:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn