How to Print the Content of a Div Element in JavaScript
-
Use the
window
Print Command to Print the Content of adiv
Element in JavaScript -
Use the
outerHTML
Attribute to Print the Content of adiv
Element in JavaScript
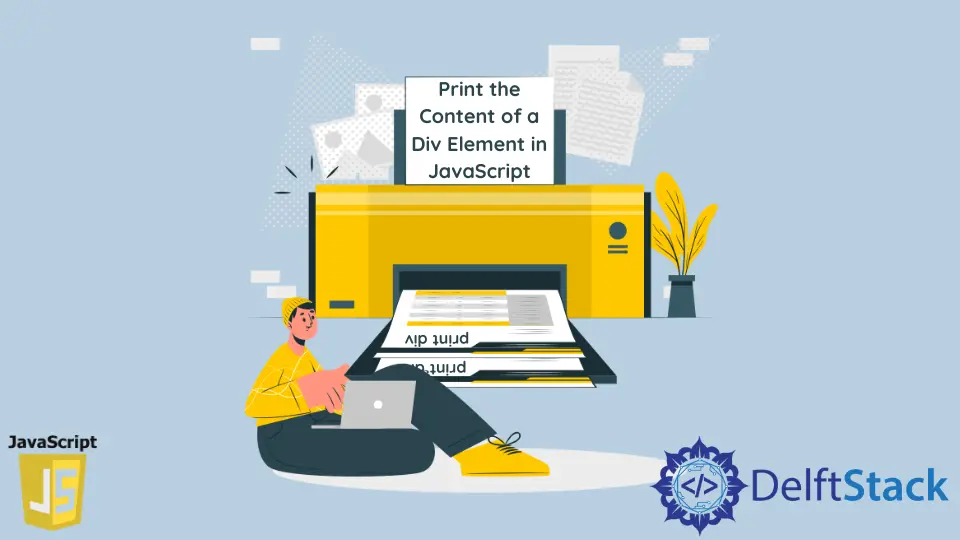
This tutorial enlightens two different approaches used to print the content of a div
element in JavaScript. To do that, we can use the JavaScript window
print command to print the current window’s content.
A Print Dialog Box is opened by the print()
method that lets users choose the desired printing options.
For instance, we can use different destinations there, save as a .pdf
file, Fax, OneNote for Windows 10 and more. On the other hand, the element.outerHTML
attribute prints the content of the div
element on the web page.
Use the window
Print Command to Print the Content of a div
Element in JavaScript
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>
Print the Content of A Div Element in JavaScript
</title>
</head>
<body>
<div id="printContent">
<h2>This is the Heading Inside the div</h2>
<p>
This is a paragraph inside the div and will be printed
on the a separate pdf file as soon as you click the button.
</p>
</div>
<input type="button" value="Click Here" onclick="printDivContent()">
</body>
</html>
JavaScript Code:
function printDivContent() {
var divElementContents = document.getElementById('printContent').innerHTML;
var windows = window.open('', '', 'height=400, width=400');
windows.document.write('<html>');
windows.document.write('<body > <h1>Div\'s Content Are Printed Below<br>');
windows.document.write(divElementContents);
windows.document.write('</body></html>');
windows.document.close();
windows.print();
}
Output:
In this output, the function printDivContent()
is called when clicking on the button.
Within this function, we are saving the innerHTML
(content) of a HTML element whose id’s value is printContent
. In our case, it is a <div>
element.
Depending on the parameter values and browser settings, the window.open()
opens a new tab or a new browser window. It takes three parameters: URL, window name, and a list of window features (height, width, etc.).
Our example code leaves the first two parameters empty and gives a comma-separated list containing height=400
and width=400
. The write()
function writes to an open HTML document stream directly.
We are writing in a new browser window using windows.document.write()
.
The close()
function closes the present window or the particular window on which it was referenced or called. The print()
method prints the content of the current window; it also opens a Print Dialog Box which lets the user select the preferred printing option.
You can find more about the window
command here.
Use the outerHTML
Attribute to Print the Content of a div
Element in JavaScript
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>
Print the Content of A Div Element in JavaScript
</title>
</head>
<body>
<div id="printContent">
<h2>This is the Heading Inside the div</h2>
<p>
This is a paragraph inside the div and will be printed
on the a web page as soon as you click the button.
</p>
</div>
<input type="button" value="Click Here" onclick="printDivContent()">
<p id="demo"></p>
</body>
</html>
JavaScript Code:
function printDivContent() {
var printDivContents = document.getElementById('printContent');
document.getElementById('demo').innerHTML = printDivContents.outerHTML;
}
Output:
The function printDivContent()
is called when clicking on the button. This function gets the first element whose id is printContent
which is a <div>
element.
Further, we replace the innerHTML
(content) of the element having id demo
with printDivContents.outerHTML
. Here, the outerHTML
attribute outputs or sets the particular HTML element and its content, including the start and end tag and its attributes.