How to Preload Image in JavaScript
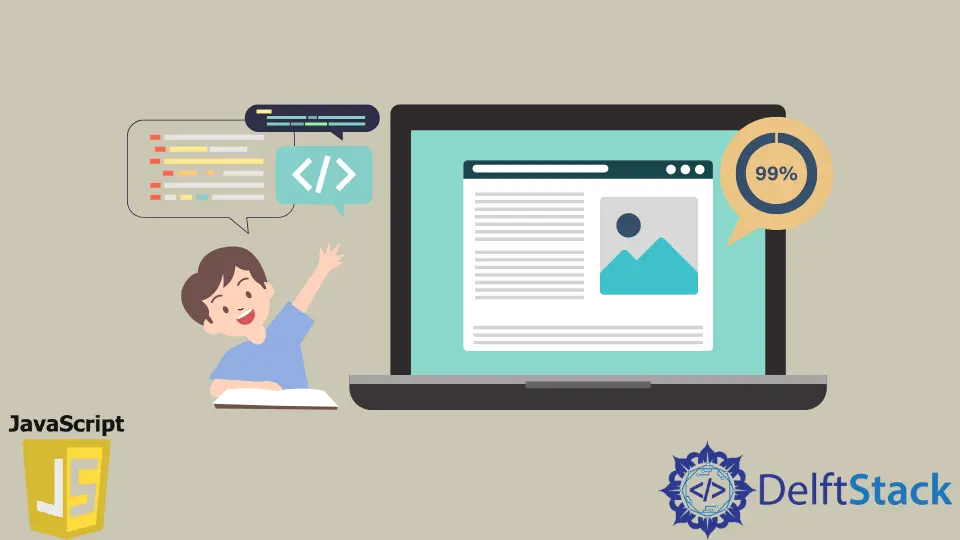
This tutorial teaches how to preload images with JavaScript.
Before talking about how to preload images, let’s first understand why we need to preload images. When we define an image using the <image>
tag in HTML, the browser sees that image, downloads it, and caches it for us before the page loads. It makes displaying images fairly simple. But when we have some dynamic loading of images triggered by JavaScript code, the browser has no prior information about it. So, such images cannot be downloaded and cached. These images will be loaded on the fly and would cause a delay in the display as the image downloads first. It makes a website look very bad, and hence these images must be preloaded.
For example: If we want to show a rollover effect, i.e., change the image displayed when we roll over the mouse on it, we have to make sure the second image is preloaded; otherwise, the image will take lots of time to load, which makes the website seem ugly and unresponsive.
Preload Images With JavaScript
Procedure
-
Initiate a variable
preloaded
to store the number of images loaded during the preloading phase and an array of imagesimages
to store the address of all the images to be preloaded. -
We define a function
preLoader()
to create image objects for all images that have to be preloaded and store thesrc
property on the object the arrayimages
. We also attach a functionprogress()
to maintain the count of images preloaded successfully and perform any action after all the images have been preloaded. -
We add an event listener to the windows calling our
preLoader()
when DOM content is loaded.
Code
var preloaded = 0;
var images = new Array("img1.jpg", "img2.jpg", "img3.jpg");
function preLoader(e) {
for (var i = 0; i < images.length; i++) {
var tempImage = new Image();
tempImage.addEventListener("load", progress, true);
tempImage.src = imageArray[i];
}
}
function progress() {
preloaded++;
if (preloaded == imageArray.length) {
//ALL Images have been loaded, perform the desired action
}
}
this.addEventListener("DOMContentLoaded", preLoader, true);
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn