How to Find the NTH Child Using JavaScript
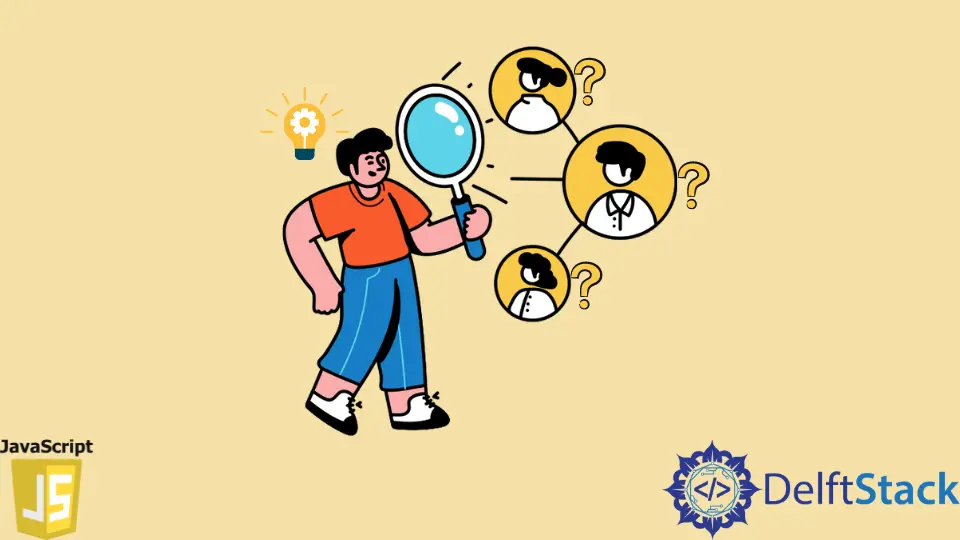
All websites are built with HTML and CSS. Web pages contain several similar elements, and sometimes we want to find certain elements among all the matching elements.
In today’s post, we’ll learn how to find the nth-child of an element using JavaScript.
Find the NTH Child of an Element With :nth-child()
JavaScript
The :nth-child()
is a CSS pseudo-class that matches elements based on their position in a group of matching elements/siblings.
A CSS pseudo-class is a keyword added to a selector that specifies a specific state of the selected items. For example, :hover
can change the color of a button when the user’s mouse cursor hovers over it.
Pseudo-classes allow you to style an element relative to the content of the document tree and relative to external factors such as browsing history (e.g., visited).
The state of its content (e.g., activated on certain form elements) or the mouse position (e.g., hover, which lets you know whether the mouse is over an element or not).
/* Selects the first <li> element in a list */
li:nth-child(1) {
color: red;
}
/* Selects every third element among any group of elements/siblings */
:nth-child(3n) {
color: red;
}
The :nth-child()
takes a single argument describing a pattern for matching the indices of elements in a sibling list.
Element indices are based on 1
. You can pass even or odd numbers as needed.
Now we know what is :nth-child
pseudo-class is. Let’s understand what is querySelector()
and querySelectorAll()
in order to find out the matching element.
The querySelectorAll()
is a built-in document
method provided by JavaScript that returns Element/NodeList
objects.
Whose selectors match the specified selectors. Even more, selectors can be passed.
See the querySelectorAll
method documentation for more information.
The querySelector()
is an intrinsic document
technique provided by JavaScript that returns the Objects/NodeList part whose selector
matches the desired selector
. See the querySelector
method documentation for more information.
The only difference between querySelectorAll()
and querySelector()
is that all objects in the matched element are returned.
Then the single object in the matched element is returned. SyntaxError
is thrown if an invalid selector is passed.
<div>
<a href="https://www.delftstack.com/howto/python/">Python</a>
<a href="https://www.delftstack.com/howto/angular/">Angular</a>
<a href="https://www.delftstack.com/howto/react/">React</a>
<a href="https://www.delftstack.com/howto/node.js/">Node.js</a>
<a href="https://www.delftstack.com/howto/php/">PHP</a>
<a href="https://www.delftstack.com/howto/java/">Java</a>
<a href="https://www.delftstack.com/howto/c/">c</a>
</div>
const thirdLink = document.querySelector('a:nth-child(3)');
console.log(thirdLink);
const everyThirdElements = document.querySelectorAll('a:nth-child(3n)');
console.log(everyThirdElements);
In the example above, we find a third anchor link using querySelector
.Try to find the first matching link and return the result.
In another example, we use querySelectorAll()
to search for all multiples of the third element. Attempts to match all matching links and returns the result in object format.
Output:
<a href="https://www.delftstack.com/howto/react/">React</a>
{
"0": <a href="https://www.delftstack.com/howto/react/">,
"1": <a href="https://www.delftstack.com/howto/java/">Java</a>,
...
}
To check the complete working code, click here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn