How to Append Leading Zeros in JavaScript
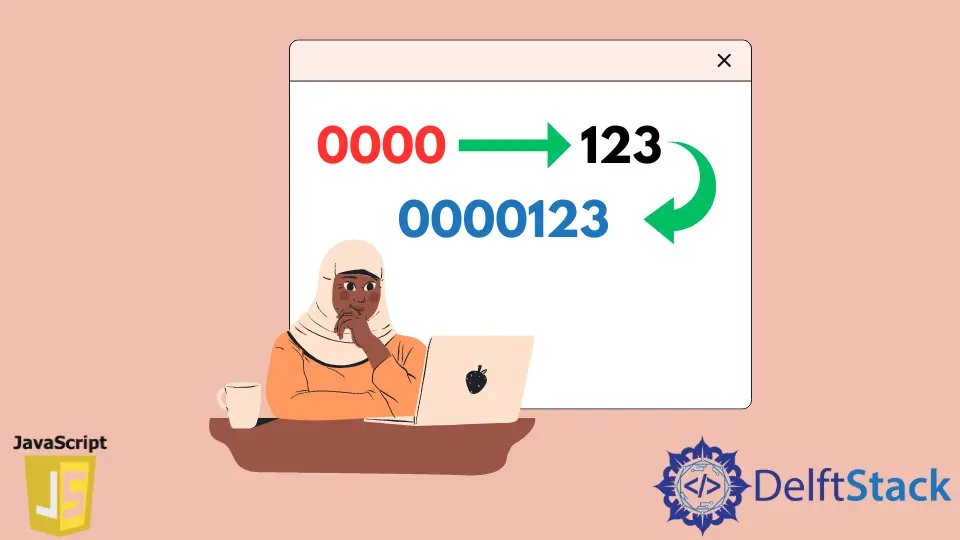
Sometimes we need to sort the strings similar to numbers. For example, 10 comes alphabetically before 2, but after 02.
Leading zeros are used to match ascending order of numbers with alphabetical order. This post will teach how to append leading zeros in JavaScript.
Append Leading Zeros to Numbers in JavaScript
The padStart()
method is an in-built method provided by JavaScript. This method pads or concatenate the current string with another string until the resulting string reaches the specified length.
If it’s necessary, it appends it multiple times. The padding is applied from the beginning of the input string, and it returns a string of the specified target length with padString
applied from the beginning.
Syntax:
padStart(targetLength)
padStart(targetLength, padString)
The String.padStart
method accepts the following 2 parameters:
- The
target length
for the string up to this length, the string is padded with the padding string. A decimal point or a minus sign is also taken into account. - The
pad string
, the substring to apply at the beginning of the string.
The padStart
method works only on strings, so to use the padStart
method, first, we had to convert the number to a string. Find more information in the documentation for padStart()
.
Steps to add leading zeros to a number:
- Convert the given number to a new string.
- Call the
padStart()
method of JavaScript to append/concat zeros to the start of the string. - The
padStart
method returns a new string with the specified padding string applied from the beginning.
function addLeadingNumberZeros(number, totalLength) {
return String(number).padStart(totalLength, '0');
}
console.log(addLeadingNumberZeros(4, 2));
console.log(addLeadingNumberZeros(4, 3));
console.log(addLeadingNumberZeros(4, 4));
Output:
"04"
"004"
"0004"
const num = '00' + 8;
console.log(num);
Output:
008
All leading zeros are automatically removed if you convert the padded string back to a number because 008 === 8
. JavaScript doesn’t keep leading zeros insignificant.
If the input string length exceeds the specified target length, the entire string returns from the padStart
method. If you need to deal with negative numbers, you need to add an if
statement that adds the minus sign after adding the leading zeros.
function addLeadingNumberZeros(number, totalLength) {
if (number < 0) {
const withoutNegativeSign = String(number).slice(1);
return '-' + withoutNegativeSign.padStart(totalLength, '0');
}
return String(number).padStart(totalLength, '0');
}
console.log(addLeadingNumberZeros(3, 2));
console.log(addLeadingNumberZeros(-3, 3));
Output:
"03"
"-003"
Add an if
statement to check if a negative number is fed to the function. Notice that we intentionally didn’t include the minus sign in the target length of the new string.
To deal with negative numbers, we had to remove the minus sign, add the leading zeros, and add the minus sign to the beginning of the string. The best alternative and simpler approach use the addition operator (+
).
const positiveNumber = '00' + 7;
console.log(positiveNumber);
const negativeNumber = '-' +
'00' + String(-7).slice(1);
console.log(negativeNumber);
When we run the code above in any browser, you will get the following output.
Output:
"007"
"-007"
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn