How to Hide Elements Using Class Name in JavaScript
-
Hide Element by Class Name Using
style.display
andstyle.visibility
Properties in JavaScript - Conclusion
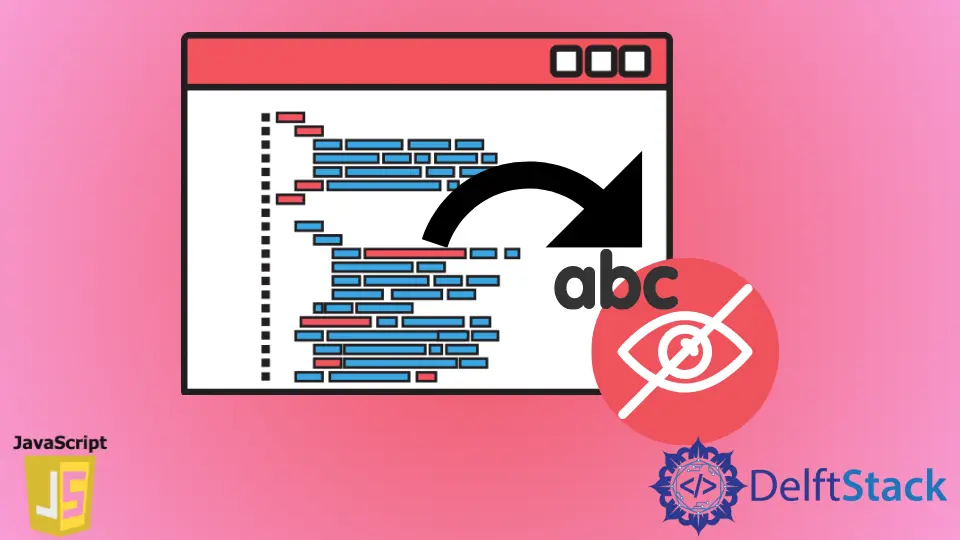
In this tutorial, we’ll look at how to hide elements in JavaScript by using their class name. For this, we will be using the style
property provided by the JavaScript language.
Hide Element by Class Name Using style.display
and style.visibility
Properties in JavaScript
We can either use the display
or visibility
CSS properties to hide the HTML element using its class name. You can only access these properties using the style
property.
Here, we have an HTML document with a style
and a body
tag that contains four div elements. The second and fourth elements have a hide-me
class.
Code Snippet - HTML:
<!DOCTYPE html>
<html>
<head>
<style>
.hide-me{
border: 1px solid #000;
padding: 5px;
margin: 10px;
}
</style>
<body>
<div>1</div>
<div class="hide-me">2</div>
<div>3</div>
<div class="hide-me">4</div>
<script src="./script.js">
</script>
</body>
</head>
</html>
We want to hide inside a variable elements
using their class name hide-me
. The getElementsByClassName()
method will return an object and this object will be stored inside the elements
method.
We utilize the for
loop to iterate over this object. Then using the style.display
and set its value to none
will hide the HTML element.
Code Snippet - JavaScript:
var elements = document.getElementsByClassName('hide-me');
for (let i = 0; i < elements.length; i++) {
elements[i].style.display = 'none';
}
Output:
The display
and visibility
properties hide the HTML element. The only difference between them is that the display
CSS property will completely hide the element from the page and the space occupied by that element, as shown above.
In contrast, the visibility
CSS property will hide the element and still occupy the web page space. To implement this, we just have to replace the display = "none"
inside the for
loop with visibility = "hidden"
as shown below.
Code Snippet - JavaScript:
for (let i = 0; i < elements.length; i++) {
elements[i].style.visibility = 'hidden';
}
Conclusion
The only way to hide an element in JavaScript is by accessing and modifying the HTML element’s CSS properties. The style
will return all the CSS properties supported by the HTML element in the form of the CSSStyleDeclaration
object.
We only have to use either the display
property or the visibility
property of all these CSS properties.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn