How to Get Child Element by Class in JavaScript
-
Get Child Element by
class
in JavaScript -
Use
querySelector()
to Get Child Element byclass
Name in JavaScript -
Use
querySelectorAll()
Method to Get All Child Nodes Having the Sameclass
in JavaScript -
Use
document.getElementById()
With Child Nodes’ Properties in JavaScript -
Use
getElementsByClassName()
Method to Get One or Multiple Child Elements byclass
Name in JavaScript -
Use
:scope
to Get Direct Children in JavaScript
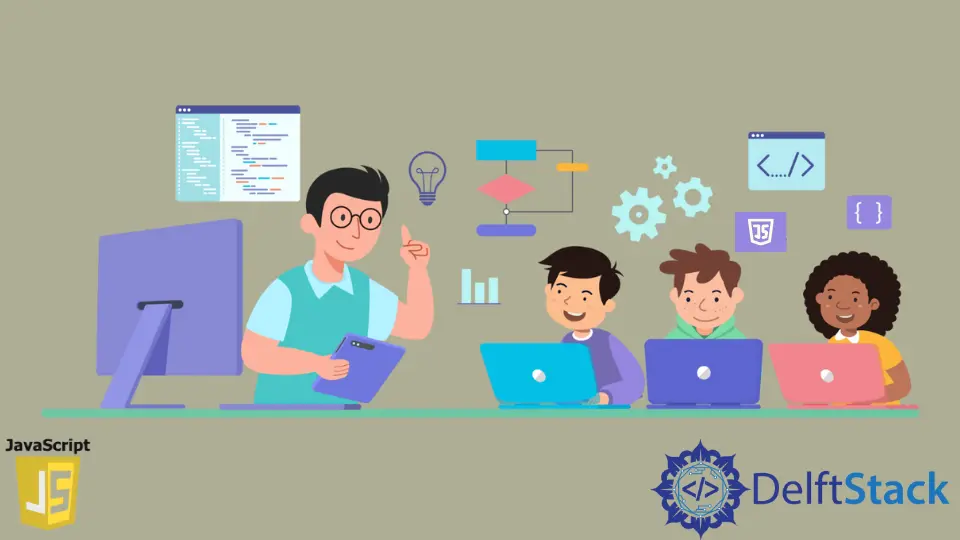
This tutorial shows how to get child elements by class
using JavaScript. It also highlights how to access one child element (first or last child element) or multiple child elements using their class
name.
Let’s start learning them sequentially.
Get Child Element by class
in JavaScript
We can use the following ways to get one or multiple child elements by class
name in JavaScript.
- Use
querySelector()
method. - Get all child nodes having the same
class
usingquerySelectorAll()
. - Use
document.getElementById()
with Child Nodes’ Properties. - Use
getElementsByClassName()
method. - Use
:scope
to get direct children.
Use querySelector()
to Get Child Element by class
Name in JavaScript
HTML Code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Get Child Elements</title>
</head>
<body>
<ul id="parent-element">
<li class="child-element-one">One</li>
<li class="child-element-two">Two</li>
<li class="child-element-three">Three</li>
<li class="child-element-four">Four</li>
</ul>
</body>
</html>
JavaScript Code:
var parent = document.querySelector('#parent-element');
var firstChild = parent.querySelector('.child-element-one');
var lastChild = parent.querySelector('.child-element-four');
document.writeln(
'The innerHTML of First Child Element is "' + firstChild.innerHTML + '"' +
' and The innerHTML of Last Child Element is "' + lastChild.innerHTML +
'"');
Output:
We first use the querySelector()
method to get the parent element. Then, call this method again on the selected parent element to get the first and last child nodes that match the specified selector.
You may have observed that the querySelector()
is scoped to a particular element here (that has the id parent-element
). This is why it only chooses the child of the element it is called.
We can also use document.getElementById()
to select the parent element. See the following example where we are selecting all child elements.
Use querySelectorAll()
Method to Get All Child Nodes Having the Same class
in JavaScript
HTML Code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Get Child Elements</title>
</head>
<body>
<ul id="parent-element">
<li class="child-element">One</li>
<li class="child-element">Two</li>
<li class="child-element">Three</li>
<li class="child-element">Four</li>
</ul>
</body>
</html>
JavaScript Code:
var parent = document.getElementById('parent-element');
var allChildElements = parent.querySelectorAll('.child-element');
for (i = 0; i < allChildElements.length; i++)
document.writeln(allChildElements[i].innerHTML);
Output:
The querySelectorAll()
method selects all child nodes with the same class
name. It returns the node list and shows SYNTAX_ERR
in case of any invalid selector(s).
Use document.getElementById()
With Child Nodes’ Properties in JavaScript
HTML Code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Get Child Elements</title>
</head>
<body>
<ul id="parent-element">
<li class="child-element-one">One</li>
<li class="child-element-two">Two</li>
<li class="child-element-three">Three</li>
<li class="child-element-four">Four</li>
</ul>
</body>
</html>
JavaScript Code:
var parent = document.getElementById('parent-element');
var firstChild = parent.firstElementChild;
var lastChild = parent.lastElementChild;
document.writeln(
'The innerHTML of First Child Element is "' + firstChild.innerHTML + '"' +
' and The innerHTML of Last Child Element is "' + lastChild.innerHTML +
'"');
Output:
Here, the firstElementChild
returns the first child element node and the lastElementChild
returns the last child element node. We can also get all child Element
nodes as follows.
JavaScript Code:
var parent = document.getElementById('parent-element');
var allChild = parent.children;
for (i = 0; i < allChild.length; i++) document.writeln(allChild[i].innerHTML);
Output:
Use getElementsByClassName()
Method to Get One or Multiple Child Elements by class
Name in JavaScript
HTML Code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Get Child Elements</title>
</head>
<body>
<ul id="parent-element">
<li class="child-element">One</li>
<li class="child-element">Two</li>
<li class="child-element">Three</li>
<li class="child-element">Four</li>
</ul>
</body>
</html>
JavaScript Code:
var childs = (document.getElementById('parent-element'))
.getElementsByClassName('child-element');
for (i = 0; i < childs.length; i++) document.writeln(childs[i].innerHTML);
Output:
The getElementsByClassName()
is a read-only property that helps to get all child nodes having the same class
name.
Use :scope
to Get Direct Children in JavaScript
HTML Code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JS Get Child Elements</title>
</head>
<body>
<ul id="parent-element">
<li class="child-element-one">One</li>
<li class="child-element-two">Two</li>
<li class="child-element-three">Three</li>
<li class="child-element-four">Four</li>
</ul>
</body>
</html>
JavaScript Code:
var parent = document.getElementById('parent-element');
var childs = parent.querySelectorAll(':scope > li');
for (i = 0; i < childs.length; i++) document.writeln(childs[i].innerHTML);
Output:
We are using a pseudo-class named :scope
, which is very useful for getting the direct children, which means the direct descendant of an element that we have already accessed.