How to Format a JavaScript Date
-
Format a JavaScript Data Using
toTimeString()
in JavaScript -
Format a JavaScript Data Using
toUTCString()
in JavaScript -
Format a JavaScript Data Using
toDateString()
in JavaScript -
Format a JavaScript Data Using
toLocaleString()
in JavaScript -
Format a JavaScript Data Using
toLocaleTimeString()
in JavaScript - Use the Custom Functions to Format the Date in JavaScript
-
Use
Moment.js
Package WithNode.js
to Format the Date in JavaScript -
Use the
dateformat
Package WithNode.js
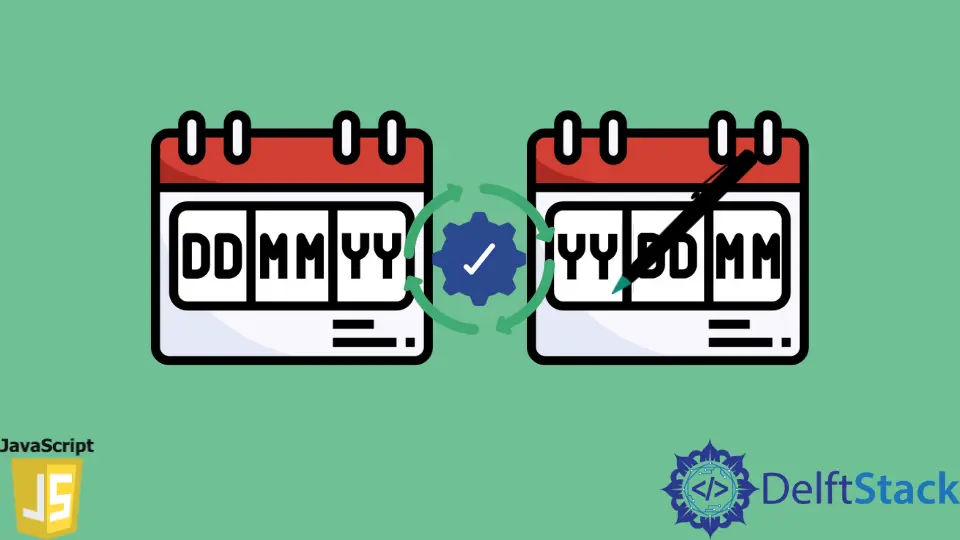
This tutorial explains how to format a JavaScript date.
We can get the current date and time by using the JavaScript Date
object. We can format the date by writing our own custom functions and using libraries like moment.js
.
First, we create a variable named date
using the Date
object to get the current date and time.
var date = new Date();
We will show the result of all formatting functions applied to this variable.
Format a JavaScript Data Using toTimeString()
in JavaScript
toTimeString()
helps extract just the string containing information about time from the date
variable.
var date = new Date();
result = date.toTimeString();
console.log(result);
Output:
"20:07:37 GMT+0100 (Central European Standard Time)"
Format a JavaScript Data Using toUTCString()
in JavaScript
This method returns the Greenwich Mean Time or the Coordinated Universal Time from the current country’s time stored in the variable.
var date = new Date();
result = date.toUTCString();
console.log(result);
Output:
"Thu, 18 Mar 2021 19:09:40 GMT"
Format a JavaScript Data Using toDateString()
in JavaScript
This method extracts the date and returns it in the form of a string.
var date = new Date();
result = date.toDateString();
console.log(result);
Output:
"Thu Mar 18 2021"
Format a JavaScript Data Using toISOString()
in JavaScript
It returns a string containing the date/time in ISO 8601 format.
var date = new Date();
result = date.toISOString();
console.log(result);
Output:
"2021-03-18T19:11:35.957Z"
Format a JavaScript Data Using toLocaleString()
in JavaScript
It converts the date object to a string using the locale settings.
var date = new Date();
result = date.toLocaleString();
console.log(result);
Output:
"3/18/2021, 8:13:03 PM"
Format a JavaScript Data Using toLocaleTimeString()
in JavaScript
It converts the date object but extracts only the time to a string using the locale settings.
var date = new Date();
result = date.toLocaleTimeString();
console.log(result);
Output:
"8:14:22 PM"
Use the Custom Functions to Format the Date in JavaScript
Date Format dd-mm-yyyy
or dd/mm/yyyy
and Similar Patterns
We use the methods getDate()
, getMonth()
, and getFullYear()
to get the parts of the date in pieces and concatenate them using the symbol we want and in the order we want.
For example we can get dd/mm/yyyy
, dd-mm-yyyy
, mm-dd-yyyy
, mm/yyyy
in whatever way we like by writing a custom function like we have written below.
var date = new Date();
const formatDate = (date) => {
let formatted_date =
date.getDate() + '-' + (date.getMonth() + 1) + '-' + date.getFullYear()
return formatted_date;
} console.log(formatDate(date));
Output:
"18-3-2021"
We can also put the month’s name like January
, February
, March
, inside the date string.
var date = new Date();
const months = [
'JAN', 'FEB', 'MAR', 'APR', 'MAY', 'JUN', 'JUL', 'AUG', 'SEP', 'OCT', 'NOV',
'DEC'
];
const formatDate = (date) => {
let formatted_date =
date.getDate() + '-' + months[date.getMonth()] + '-' + date.getFullYear()
return formatted_date;
} console.log(formatDate(date));
Output:
"18-MAR-2021"
Date Format yyyy-mm-dd hh:mm:ss
and Similar Patterns
We use all the methods getDate()
, getMonth()
and getFullYear()
, getHour()
, getminutes()
, getsecond()
to get the parts of date and time individually and concatenate them using the symbol we want and in the order we want.
var date = new Date();
const formatDate =
(current_datetime) => {
let formatted_date = current_datetime.getFullYear() + '-' +
(current_datetime.getMonth() + 1) + '-' + current_datetime.getDate() +
' ' + current_datetime.getHours() + ':' +
current_datetime.getMinutes() + ':' + current_datetime.getSeconds();
return formatted_date;
}
console.log(formatDate(date));
Output:
"2021-3-18 20:21:2"
Use Moment.js
Package With Node.js
to Format the Date in JavaScript
It is considered the best date and time library in JavaScript. It is straightforward to use, and one does not need to memorize and build all these different functions. Users can easily write a string template according to their formatting needs.
const moment = require('moment');
let m = moment();
m.format('[Time: ] h:mm:ss a'); // output of the form `Time: 11:39:03 pm`
The above code demonstrates one of the ways moment.js
allows us to format date and time using the Node REPL.
Use the dateformat
Package With Node.js
Another package like Moment.js
can help us to format Date. It works with both Node.js
and Browser-side JavaScript
. It extends the Date object by including a .format()
method.
Browser Side
Day = new Date();
Day.format('dd-m-yy'); // Returns '16-3-21'
The above code displays the simplicity of using dateformat
package with the Date object.
Node.js
const dateformat = require('dateformat');
let now = new Date();
dateformat(now, 'dddd, mmmm dS, yyyy, h:MM:ss TT'); // returns 'Tuesday, March
// 16th, 2021, 11:32:08 PM'
The above code demonstrates the use of dateformat
package to format date with Node REPL.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedInRelated Article - JavaScript Date
- How to Add Months to a Date in JavaScript
- How to Add Minutes to Date in JavaScript
- How to Add Hours to Date Object in JavaScript
- How to Calculate Date Difference in JavaScript
- How to Calculate Age Given the Birth Date in YYYY-MM-DD Format in JavaScript
- How to Get First and Last Day of Month Using JavaScript