How to Add Hours to Date Object in JavaScript
-
Use the
setHours()
Method to Add Hours toDate
Object in JavaScript -
Use the
getHours()
andsetHours()
Method to Update the Current Hours Value in JavaScript
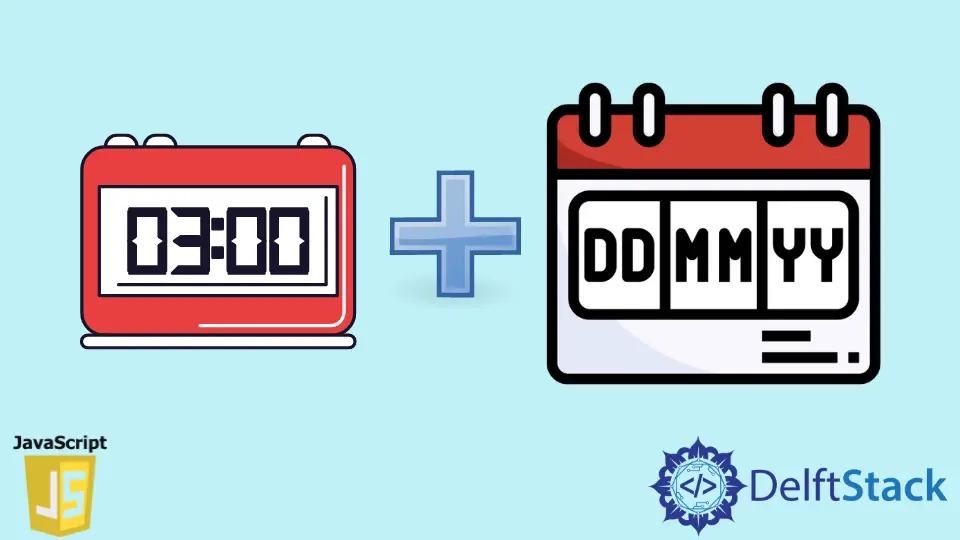
The Date
object in JavaScript provides you with your system’s current week of the day, date, and time (with timezone). The default timezone of the Date
object is UTC, but the basic methods to fetch the date and time or its components all work based on the local (system’s) timezone.
The Date
object also provides us with various methods like setHours()
, setMonth()
, getTime()
, getDay()
, etc., using which we can access or modify the values of the Date
object like time, hours, minutes, timezone and many more. To see the full list of methods, visit the [Date
](Date - JavaScript | MDN) object docs.
Using some of these methods, let’s now see how to add hours to the Date
object in JavaScript.
Use the setHours()
Method to Add Hours to Date
Object in JavaScript
To update the hour’s value of the Date
object, we will be using the built-in setHours()
method. This method will only update the hour’s value, not the minutes and seconds.
Let’s now take an example and see how we can update the hour’s value using the setHours()
method. First, to get the system’s current time, you have to create an object of the Date
using the new
keyword and then store it inside some variable currentDateTime
and then print that variable.
This will give you the local time of your system.
Code Snippet - JavaScript:
var currentDateTime = new Date();
function addHours(hr) {
currentDateTime.setHours(hr);
}
console.log(currentDateTime);
addHours(5);
console.log(currentDateTime);
Output:
Mon May 5 2022 00:28:11 GMT+0530 (India Standard Time)
Mon May 5 2022 05:28:11 GMT+0530 (India Standard Time)
To add the hour’s value, we will create a function called addHours()
, which will take a single parameter hr
that will be an integer value representing the hour value that we want to add to the current time.
Inside this function, we will call the setHours()
method on the variable currentDateTime
that holds the current local date and time, and then we will pass the hr
inside it.
This will set whatever hr
argument we pass to the addHours()
function. We are passing the integer value 5
to the addHours()
function.
Finally, if you print the time before and after calling the addHours
function, you will see that it has added the value 5
to the current time, as shown in the output above.
With this method, you can replace the existing or the current hour value with a new one. But what if you want to update the current hour value instead of replacing it entirely with a new value.
Let’s now see how to update the current hour values using some predefined methods of the Date
object inside JavaScript.
Use the getHours()
and setHours()
Method to Update the Current Hours Value in JavaScript
We are directly replacing the hour’s value with the new value until now. But what if you want to update the current hour’s value and not replace it?
You must simultaneously use both the getHours()
and setHours()
methods.
Code Snippet - JavaScript:
var currentDateTime = new Date();
console.log(currentDateTime);
currentDateTime.setHours(currentDateTime.getHours() + 5);
console.log(currentDateTime);
Output:
Mon May 5 2022 20:29:17 GMT+0530 (India Standard Time)
Tue May 6 2022 01:29:17 GMT+0530 (India Standard Time)
Here, also we have to first store the current date and time returned from the Date
object inside a variable currentDateTime
. Then we will make use of both the setHours()
and getHours()
function simultaneously.
Since we want to set the hours value, we will use the setHours()
method on the currentDateTime
variable. We will call the getHours()
method on the currentDateTime
variable inside this method.
The getHours()
method will give us the current value, and then we will add 5
to that value, and then the setHours()
method will set that value to the currentDateTime
variable.
And if you print the time before and after using the setHours()
method, you see that the current time is 20
; we add the value 5
to this value, and then it becomes 1
.
This is because the entire day is 24
hours, and 20
plus 5
becomes 25
, so after the 24
hours are over, it will start with 1
, as shown in the output above.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn