How to Calculate Date Difference in JavaScript
- Method 1: Using the Date Object
- Method 2: Using Date.UTC for Greater Accuracy
- Method 3: Using a Library like Moment.js
- Conclusion
- FAQ
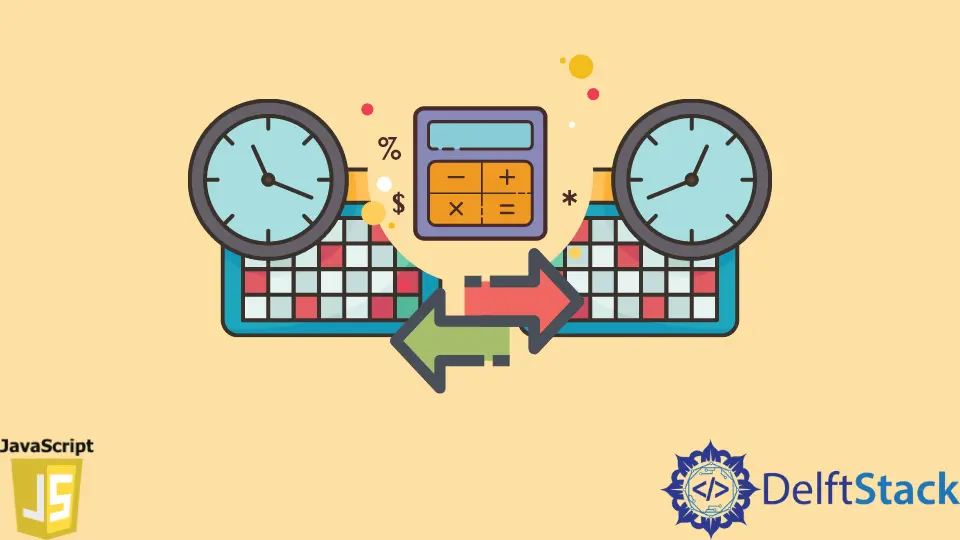
In today’s post, we’ll learn to find the difference between two dates in JavaScript. Understanding how to manipulate dates is crucial for web developers, as it can help in various applications such as scheduling events, tracking deadlines, or displaying time-sensitive information. JavaScript provides powerful tools for working with dates, allowing you to easily calculate the difference between two dates in various formats. Whether you’re building a simple web application or a complex system, mastering date calculations will enhance your coding skills and improve your project’s functionality. Let’s dive into the methods to calculate the date difference in JavaScript!
Method 1: Using the Date Object
JavaScript’s built-in Date object is a straightforward way to calculate the difference between two dates. This method involves creating Date instances for both dates and then utilizing the built-in methods to find the difference in milliseconds. Here’s how you can do it:
const date1 = new Date('2023-10-01');
const date2 = new Date('2023-10-15');
const timeDifference = date2 - date1;
const daysDifference = timeDifference / (1000 * 3600 * 24);
console.log(daysDifference);
Output:
14
This code snippet starts by creating two Date objects for October 1, 2023, and October 15, 2023. The time difference is calculated by subtracting the two dates, resulting in a difference measured in milliseconds. To convert this value into days, we divide by the number of milliseconds in a day (1000 milliseconds per second, 3600 seconds per hour, and 24 hours per day). The final result, which is 14 days, is logged to the console.
Using the Date object is a simple yet effective way to calculate date differences. However, keep in mind that this method can be affected by time zones and daylight saving time changes. For most applications, though, this method provides a quick solution for basic date calculations.
Method 2: Using Date.UTC for Greater Accuracy
For scenarios where you need greater accuracy, especially when dealing with different time zones, the Date.UTC method comes in handy. This method allows you to create a date in UTC format, ensuring that your calculations are not affected by local time variations. Here’s how to use it:
const date1 = Date.UTC(2023, 9, 1); // October is month 9
const date2 = Date.UTC(2023, 9, 15);
const timeDifference = date2 - date1;
const daysDifference = timeDifference / (1000 * 3600 * 24);
console.log(daysDifference);
Output:
14
In this example, we use Date.UTC to create two dates in UTC format. The month argument is zero-based, meaning October is represented as 9. The time difference is calculated in the same way as before, and the result is again 14 days. This method is particularly useful for applications that require precise date calculations across different time zones, ensuring that the results remain consistent regardless of the user’s locale.
Method 3: Using a Library like Moment.js
For more complex date manipulations, using a library like Moment.js can significantly simplify the process. Moment.js provides a comprehensive set of functions for parsing, validating, manipulating, and displaying dates and times. Here’s how you can calculate the date difference using Moment.js:
const moment = require('moment');
const date1 = moment('2023-10-01');
const date2 = moment('2023-10-15');
const daysDifference = date2.diff(date1, 'days');
console.log(daysDifference);
Output:
14
In this code snippet, we first import the Moment.js library. We then create two moment objects for our dates. The diff
method is used to calculate the difference between the two dates in days. The result is logged to the console, showing a difference of 14 days. Moment.js handles various edge cases, such as leap years and time zones, making it a powerful tool for developers who need to perform extensive date calculations.
While Moment.js is a fantastic library, it’s worth noting that it has been deprecated in favor of modern alternatives like date-fns and Luxon. However, it remains popular for its ease of use and wide adoption in existing projects.
Conclusion
Calculating the difference between two dates in JavaScript is an essential skill for any developer. Whether you choose to use the built-in Date object, the UTC method for accuracy, or a library like Moment.js for more complex scenarios, each method has its strengths. By understanding these techniques, you can effectively manage date calculations in your applications, leading to better user experiences and more robust functionality. As you continue to work with dates in JavaScript, you’ll find that these skills will serve you well in a variety of programming tasks.
FAQ
- how do I calculate the difference between two dates in JavaScript?
You can calculate the difference by creating Date objects and subtracting them, then converting the result from milliseconds to days.
-
can I use libraries to handle date differences?
Yes, libraries like Moment.js or date-fns can simplify date calculations and provide additional features for manipulation and formatting. -
does the time zone affect date calculations?
Yes, time zones can affect date calculations, especially when using the local Date object. Using UTC methods can help mitigate these issues. -
what is the best method for calculating date differences?
The best method depends on your needs. For simple calculations, the built-in Date object is sufficient. For more complex scenarios, consider using libraries. -
is Moment.js still recommended for new projects?
While Moment.js is still widely used, it has been deprecated. Consider using alternatives like date-fns or Luxon for new projects.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn