How to Calculate Age Given the Birth Date in YYYY-MM-DD Format in JavaScript
- Use Native Date Methods to Calculate Age Given the Birth Date in YYYY-MM-DD Format in JavaScript
-
Use the
Moment.js
Library to Calculate Age Given the Birth Date in YYYY-MM-DD Format in JavaScript - Use Vanilla JavaScript to Calculate Age Given the Birth Date in YYYY-MM-DD Format
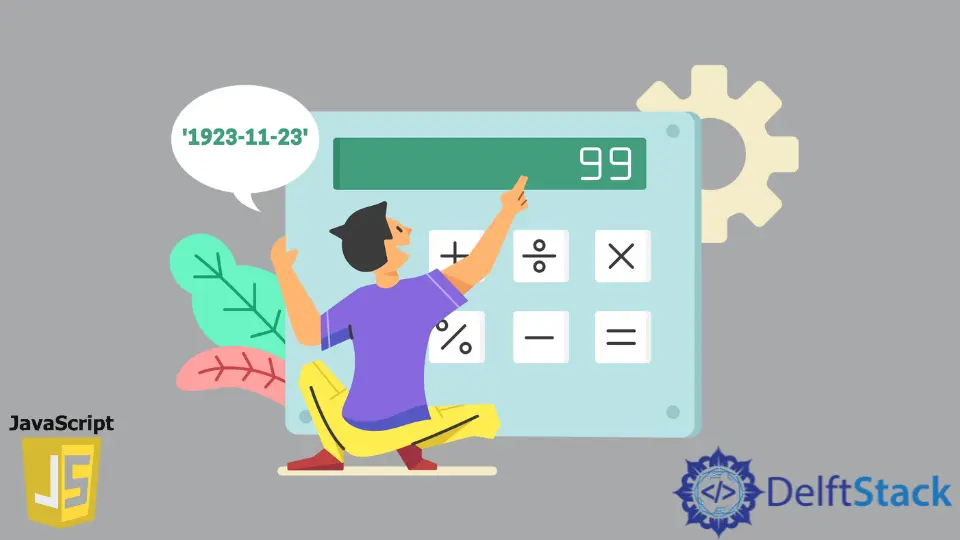
This article will describe how to calculate the age given the birth date in YYYY-MM-DD format.
Use Native Date Methods to Calculate Age Given the Birth Date in YYYY-MM-DD Format in JavaScript
We can use native date methods to compute a person’s age given a YYYY-MM-DD birth date.
const calculateAge = (birthday) => {
const ageDifMs = Date.now() - new Date(birthday).getTime();
const ageDate = new Date(ageDifMs);
return Math.abs(ageDate.getUTCFullYear() - 1970);
} console.log(calculateAge('1999-11-23'))
Output:
22
Click and visit this link to see the working code of the above example.
Explanation:
- First, we built the
calculateAge
function with the birthday input, which takes a date string to convert and use to calculate the age. - We subtracted
Date.now
from the function, which contains the timestamp of the current date-time in milliseconds. - We constructed a new Date instance. Then, we called
getTime
to get the millisecond-accurate timestamp of the birthday we passed. - The result is then assigned to
ageDifMs.
- Then, we provided
ageDifMs
to the date functionObject() {[native code]}
to receive theageDate,
which is a date formed from midnight UTC on January 1, 1970, plus the timespan defined by the time we pass in.
Use the Moment.js
Library to Calculate Age Given the Birth Date in YYYY-MM-DD Format in JavaScript
We can also use the Moment.js
library to make date calculations easier.
const calculateAge = (birthday) => {
const startDate = new Date();
const endDate = new Date(birthday);
return Math.abs(moment.duration(endDate - startDate).years());
} console.log(calculateAge('1999-11-23'))
Output:
22
Explanation:
- We used the birthday argument in the
calculateAge
function, the same as before. - Instead of utilizing native date methods, we determined the duration using the
moment.duration
method. - To acquire the instant duration object, we called it with
endDate - startDate
. - Then, we used the term years to express the length of time as the number of years.
- Then, we used
Math.abs
to double-check that the result was positive. - The console log should then display the same information as previously.
Use Vanilla JavaScript to Calculate Age Given the Birth Date in YYYY-MM-DD Format
First, we took the HTML file and created a <div>
, giving a placeholder id to it, which will be used later when the function of innerhtml
will be called in JavaScript.
<div id="placeholder">
<p>Changing Date of Birth in JavaScript</p>
</div>
Then, in the JS document, we first create a variable Date_of_Birth
to store a person’s date of birth.
var Date_of_Birth = 'November 23, 1999';
var millisecondsBetweenDOBAnd1970 = Date.parse(Date_of_Birth);
var millisecondsBetweenNowAnd1970 = Date.now();
var ageInMilliseconds =
millisecondsBetweenNowAnd1970 - millisecondsBetweenDOBAnd1970;
var milliseconds = ageInMilliseconds;
var second = 1000;
var minute = second * 60;
var hour = minute * 60;
var day = hour * 24;
var month = day * 30;
var year = day * 365;
// Age is coverted by using tag `math`
var years = Math.round(milliseconds / year);
var months = years * 12;
var days = years * 365;
var hours = Math.round(milliseconds / hour);
var seconds = Math.round(milliseconds / second);
function printResults() {
var message = 'Age in Years : ' + years + '</br>Age in Months : ' + months +
'</br>Age in Days : ' + days + '</br>Age in Hours : ' + hours +
'</br>Age in Seconds : ' + seconds +
'</br>Age in Milliseconds : ' + milliseconds;
document.getElementById('placeholder').innerHTML = message;
}
window.onload = printResults;
Output:
Age in Years : 22
Age in Months : 264
Age in Days : 8030
Age in Hours : 195551
Age in Seconds : 703983954
Age in Milliseconds : 703983954456
You can run this code using this link.
Explanation:
The Date.parse()
function parsed a date string and returned the number of milliseconds from January 1, 1970, midnight to this date. Since January 1, 1970, the Date.now()
function returns the number of milliseconds.
We are using these functions as a starting point. To hold the number of milliseconds between DOB/NOW and January 1, 1970, we established two new variables, millisecondsBetweenDOBAnd1970
and millisecondsBetweenNowAnd1970
.
Then, we subtract DOB from NOW to find a person’s age in milliseconds. When we have a person’s age in milliseconds, we must implement some basic logic to convert it to Years, Months, Days, Hours, Minutes, and Seconds.