How to Add Minutes to Date in JavaScript
- Understanding the JavaScript Date Object
- Method 1: Using setMinutes()
- Method 2: Using Date Constructor with Timezone Adjustments
- Method 3: Using a Utility Function
- Conclusion
- FAQ
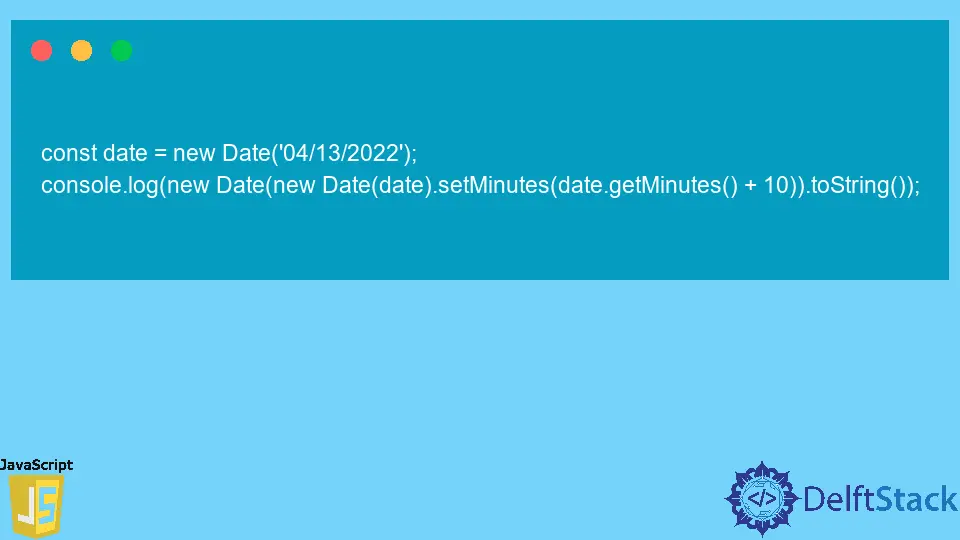
In today’s post, we’ll learn to add minutes to the date in JavaScript. Working with dates and times can sometimes feel daunting, especially when you need to manipulate them for various applications. Whether you’re building a scheduling app or just need to adjust timestamps, knowing how to add minutes to a date is essential. JavaScript provides powerful tools to handle date and time manipulation through its built-in Date
object.
In this article, we will explore different methods to add minutes to a date, complete with clear examples and explanations. By the end of this post, you will have a solid understanding of how to effectively manage date and time in your JavaScript projects.
Understanding the JavaScript Date Object
Before diving into adding minutes, it’s crucial to understand the Date
object in JavaScript. The Date
object represents a single moment in time in a platform-independent format. You can create a new date object using the new Date()
constructor, which can take various arguments, including a date string or timestamp.
Here’s how you can create a new date object:
let currentDate = new Date();
Output:
Current date and time
This currentDate
object now holds the current date and time. You can access various components of this date, such as the year, month, day, hours, minutes, and seconds. Understanding this will help you manipulate the date effectively.
Method 1: Using setMinutes()
One of the simplest ways to add minutes to a date in JavaScript is by using the setMinutes()
method. This method sets the minutes for a specified date according to local time. You can easily get the current minutes using getMinutes()
and then add the desired amount.
Here’s a straightforward example:
let currentDate = new Date();
let minutesToAdd = 15;
currentDate.setMinutes(currentDate.getMinutes() + minutesToAdd);
Output:
Updated date and time after adding minutes
In this example, we first create a new date object for the current date and time. We specify how many minutes we want to add—in this case, 15. The setMinutes()
method takes the current minutes, adds the specified number, and updates the currentDate
object accordingly. This method is particularly useful because it automatically handles overflow, meaning if the addition of minutes exceeds 60, it will adjust the hours and days accordingly.
Method 2: Using Date Constructor with Timezone Adjustments
Another method to add minutes to a date is by creating a new date object using the timestamp. You can calculate the new timestamp by adding the desired number of minutes (in milliseconds) to the current timestamp. This method is advantageous when dealing with time zones, as it allows for precise adjustments.
Here’s how you can do it:
let currentDate = new Date();
let minutesToAdd = 30;
let newDate = new Date(currentDate.getTime() + minutesToAdd * 60000);
Output:
New date and time after adding minutes
In this example, we first get the current date and time. We then calculate the new date by adding the minutes (converted to milliseconds) to the current timestamp. The getTime()
method returns the number of milliseconds since January 1, 1970, which allows for precise calculations. This method is particularly beneficial when you need to ensure that you’re accounting for time zone differences.
Method 3: Using a Utility Function
If you find yourself needing to add minutes to dates frequently, creating a utility function can simplify your code and improve reusability. A utility function encapsulates the logic, allowing you to call it whenever necessary without repeating code.
Here’s an example of a utility function:
function addMinutes(date, minutes) {
return new Date(date.getTime() + minutes * 60000);
}
let currentDate = new Date();
let updatedDate = addMinutes(currentDate, 45);
Output:
Updated date and time after adding minutes
In this code snippet, we define a function called addMinutes
, which takes a date object and the number of minutes to add. Inside the function, we calculate the new date by adding the minutes (converted to milliseconds) to the current timestamp. This approach keeps your code clean and easy to manage, especially in larger applications where date manipulation is common.
Conclusion
Adding minutes to a date in JavaScript is a straightforward process, whether you use built-in methods like setMinutes()
, calculate timestamps, or create utility functions. Each method has its advantages, and the choice largely depends on your specific use case. By mastering these techniques, you can handle date and time manipulation in your projects with confidence. Now that you have the tools to add minutes to dates, you can enhance your applications and improve user experience.
FAQ
-
How can I add hours instead of minutes in JavaScript?
You can use a similar approach by multiplying the number of hours by 3600000 (the number of milliseconds in an hour) and adding that to the current timestamp. -
Does adding minutes account for daylight saving time?
Yes, using thesetMinutes()
method adjusts for daylight saving time automatically based on the local time zone. -
What if I want to subtract minutes instead of adding them?
You can simply subtract the desired number of minutes by using a negative value or by adjusting the calculations accordingly. -
How can I format the updated date for display?
You can use thetoLocaleString()
method or libraries like Moment.js or date-fns for more complex formatting options. -
Can I add minutes to a specific date instead of the current date?
Yes, you can create a date object for any specific date and then apply the same methods to add minutes to that date.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn