How to Create A Count Up Timer in JavaScript
-
Use
setInterval()
andMath
Functions to Display JavaScript Count Up Timer -
Use
setInterval()
andpad()
Functions to Display JavaScript Count Up Timer -
Use
jQuery
to Display JavaScript Count Up Timer - Use Object and Properties to Display JavaScript Count Up Timer
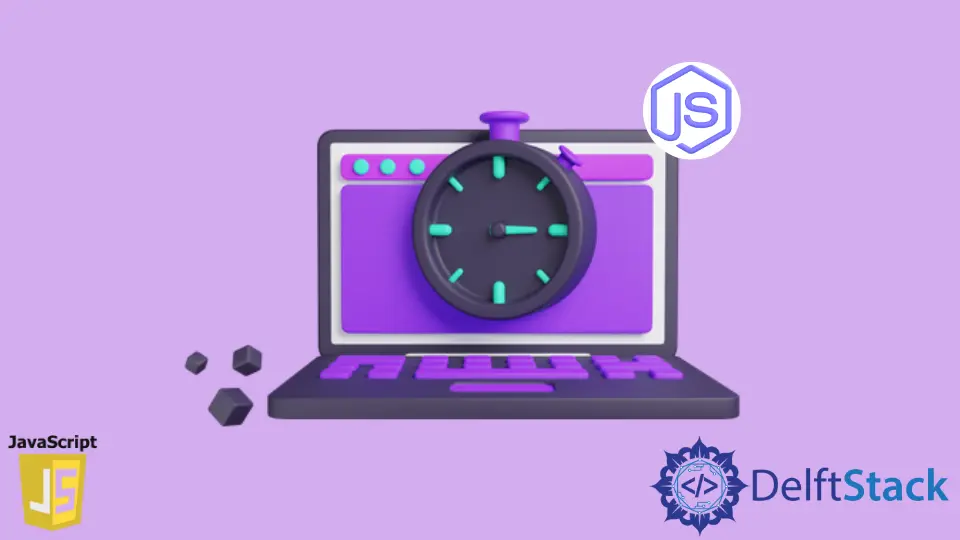
This tutorial introduces you to the JavaScript count-up timer. It uses setInterval()
, pad()
, clearInterval()
, and Math.floor()
functions and objects to create count up timer.
It also illustrates how jQuery can help you to make your code optimized.
Use setInterval()
and Math
Functions to Display JavaScript Count Up Timer
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Count Up Timer in JavaScript</title>
</head>
<body>
<div id="count_up_timer">00:00:00</div>
<button id="stop_count_up_timer" onclick="clearInterval(timerVariable)">Stop Time</button>
</body>
</html>
CSS Code:
#count_up_timer{
font-size: 100px;
font-weight: bold;
color: darkblue;
}
#stop_count_up_timer{
background-color:black;
color:white
}
JavaScript Code:
var timerVariable = setInterval(countUpTimer, 1000);
var totalSeconds = 0;
function countUpTimer() {
++totalSeconds;
var hour = Math.floor(totalSeconds / 3600);
var minute = Math.floor((totalSeconds - hour * 3600) / 60);
var seconds = totalSeconds - (hour * 3600 + minute * 60);
document.getElementById('count_up_timer').innerHTML =
hour + ':' + minute + ':' + seconds;
}
Output:
In this code example, the setInterval()
function calls the countUpTimer()
function every second (1 second = 1000 milliseconds). In the countUpTimer()
function, the variable totalSeconds
gets incremented by 1 first and then used for conversion in hour
, minute
, and seconds
.
After that, first element whose id’s value is count_up_timer
gets selected. The HTML content (innerHTML
) got replaced with the timer (hour:minute:seconds
). The count-up timer will stop as soon as you click on the Stop Timer
button.
The setInterval()
function calls a function at a given interval. It takes two parameters, and both are required. The first parameter is a function, and the other is an interval in milliseconds.
However, setInterval()
method keeps calling the function until the window is closed or clearInterval()
function is called. It returns the id
of the time that we can use with clearInterval()
function to stop the timer.
The .innerHTML
property returns or modify the HTML content of the specified element. The Math.floor()
will return the biggest integer (less than or equal to the given number).
Use setInterval()
and pad()
Functions to Display JavaScript Count Up Timer
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Count Up Timer in JavaScript</title>
</head>
<body>
<h1>Count Up Timer For One Hour</h1>
<div id = "timer">
<span id="minutes"></span>:<span id="seconds"></span>
</div>
</body>
</html>
CSS Code:
#timer{
font-size:100px;
color:green;
margin-left:80px;
}
JavaScript Code:
var second = 0;
function pad(value) {
return value > 9 ? value : '0' + value;
}
setInterval(function() {
document.getElementById('seconds').innerHTML = pad(++second % 60);
document.getElementById('minutes').innerHTML = pad(parseInt(second / 60, 10));
}, 1000);
Output:
In this example code, the pad()
function adds a leading zero. It checks if the value passed to it is a single digit (less than or equal to 9), then it adds a leading zero, otherwise not.
The setInterval()
function calls the given function at a particular interval. The interval must be in milliseconds. You can visit this link to read.
Then, the setInterval()
will call an anonymous function to get the elements whose id’s values are seconds
and minutes
. This anonymous function replaces the innerHTML
(HTML Content) with the value returned by pad()
function.
The parseInt()
function parses the given string argument and returns an integer of particular radix.
Use jQuery
to Display JavaScript Count Up Timer
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Count Up Timer in JavaScript</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
</head>
<body>
<h1>Count Up Timer For One Hour</h1>
<div id = "timer">
<span id="minutes"></span>:<span id="seconds"></span>
</div>
</body>
</html>
CSS Code:
#timer{
font-size:100px;
color:green;
margin-left:80px;
}
JavaScript Code:
var second = 0;
function pad(value) {
return value > 9 ? value : '0' + value;
}
setInterval(function() {
$('#seconds').html(pad(++second % 60));
$('#minutes').html(pad(parseInt(second / 60, 10)));
}, 1000);
Output:
What if you want a leading zero but don’t want to use the pad()
function and the jQuery library. Then, the following solution is for you.
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Count Up Timer in JavaScript</title>
</head>
<body>
<div id="count_up_timer">00:00:00</div>
<button id="stop_count_up_timer" onclick="clearInterval(timerVariable)">Stop Time</button>
</body>
</html>
CSS Code:
#count_up_timer{
font-size: 100px;
font-weight: bold;
color: darkblue;
}
#stop_count_up_timer{
background-color:black;
color:white
}
JavaScript Code:
var timerVariable = setInterval(countUpTimer, 1000);
var totalSeconds = 0;
function countUpTimer() {
++totalSeconds;
var hour = Math.floor(totalSeconds / 3600);
var minute = Math.floor((totalSeconds - hour * 3600) / 60);
var seconds = totalSeconds - (hour * 3600 + minute * 60);
if (hour < 10) hour = '0' + hour;
if (minute < 10) minute = '0' + minute;
if (seconds < 10) seconds = '0' + seconds;
document.getElementById('count_up_timer').innerHTML =
hour + ':' + minute + ':' + seconds;
}
Output:
In the above code, if
statements are used to check hour
, minute
, and seconds
. A leading zero will be added if they are less than 10 (that means they are single-digit); otherwise, not.
You may have noticed that all the count-up timers that we learned start as soon as the code is executed. What if we can have full control over the JavaScript count-up timer? Let’s learn that also.
Use Object and Properties to Display JavaScript Count Up Timer
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Count Up Timer in JavaScript</title>
</head>
<body>
<div id="timer">
<span id="minutes">00</span>:<span id="seconds">00</span>
</div>
<div id="control">
<button id="startbtn">START</button>
<button id="pausebtn">PAUSE</button>
<button id="resumebtn">RESUME</button>
<button id="resetbtn">RESET</button>
</div>
</body>
</html>
CSS Code:
#timer{
font-size: 100px;
font-weight: bold;
}
#control>#startbtn{
background-color:green;
color:white;
}
#control>#pausebtn{
background-color:blue;
color:white;
}
#control>#resumebtn{
background-color:orange;
color:white;
}
#control>#resetbtn{
background-color:red;
color:white;
}
JavaScript Code:
var Clock = {
totalSeconds: 0,
startTimer: function() {
if (!this.interval) {
var self = this;
function pad(val) {
return val > 9 ? val : '0' + val;
}
this.interval = setInterval(function() {
self.totalSeconds += 1;
document.getElementById('minutes').innerHTML =
pad(Math.floor(self.totalSeconds / 60 % 60));
document.getElementById('seconds').innerHTML =
pad(parseInt(self.totalSeconds % 60));
}, 1000);
}
},
resetTimer: function() {
Clock.totalSeconds = null;
clearInterval(this.interval);
document.getElementById('minutes').innerHTML = '00';
document.getElementById('seconds').innerHTML = '00';
delete this.interval;
},
pauseTimer: function() {
clearInterval(this.interval);
delete this.interval;
},
resumeTimer: function() {
this.startTimer();
},
};
document.getElementById('startbtn').addEventListener('click', function() {
Clock.startTimer();
});
document.getElementById('pausebtn').addEventListener('click', function() {
Clock.pauseTimer();
});
document.getElementById('resumebtn').addEventListener('click', function() {
Clock.resumeTimer();
});
document.getElementById('resetbtn').addEventListener('click', function() {
Clock.resetTimer();
});
Output:
Here, we created an object named Clock
having 5 properties named as totalSeconds
, startTimer
, resetTimer
, pauseTimer
, and resumeTimer
. The value of each property is an anonymous function with its own function body.
this
keyword refers to an object it belongs to and always holds the reference for one object.
For instance, this.resumeTimer
means resumeTimer
property of this
(Clock) object.
The clearInterval()
function is used to stop the count-up timer. Remember that each property of the Clock
object will work on the click event.