How to Create A Countdown Timer in JavaScript
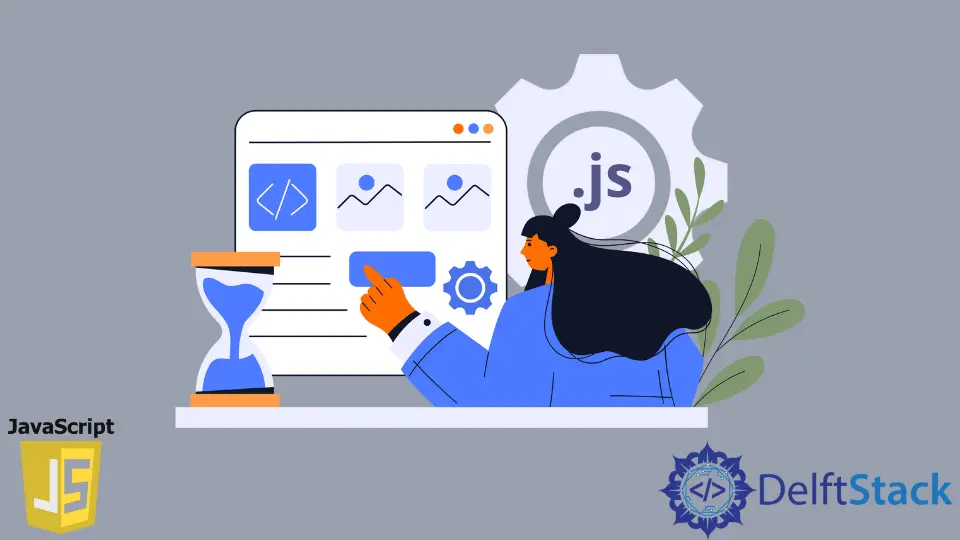
This article will give you an easy way to create a countdown timer in JavaScript.
Following is the code for a timer for a minute and a half.
<h1 class="text-center" id="count-down-timer"></h1>
function paddedFormat(num) {
return num < 10 ? '0' + num : num;
}
function startCountDown(duration, element) {
let secondsRemaining = duration;
let min = 0;
let sec = 0;
let countInterval = setInterval(function() {
min = parseInt(secondsRemaining / 60);
sec = parseInt(secondsRemaining % 60);
element.textContent = `${paddedFormat(min)}:${paddedFormat(sec)}`;
secondsRemaining = secondsRemaining - 1;
if (secondsRemaining < 0) {
clearInterval(countInterval)
};
}, 1000);
}
window.onload = function() {
let time_minutes = 1; // Value in minutes
let time_seconds = 30; // Value in seconds
let duration = time_minutes * 60 + time_seconds;
element = document.querySelector('#count-down-timer');
element.textContent =
`${paddedFormat(time_minutes)}:${paddedFormat(time_seconds)}`;
startCountDown(--duration, element);
};
Example Code of Countdown Timer in JavaScript
-
Set the HTML tag for the timer
In the code above, the
<h1>
tag holds the timer display. Use this code in your webpage HTML. Theid="count-down-timer"
is required as the element is accessed in JavaScript with thecount-down-timer
id to change the element text dynamically on run time.<h1 class="text-center" id="count-down-timer"></h1>
-
Set the timer value
Once the tag is in place, the next step is to set the count time value. Usually, web pages use a hardcoded time built into their code, which is not customizable. You can set the timer in the
window.onload
method intime_minutes
andtime_seconds
. For instance, if you wish to set a timer for one and a half minutes, then set the code as follows:let time_minutes = 1; // Value in minutes let time_seconds = 30; // Value in seconds
If you wish to set for 3 minutes, then set
time_minutes = 3
and leave thetime_seconds = 0
. Now once you run your code or reload your HTML, you can get the updated output.
Explanation of Countdown Timer Code in JavaScript
-
Execution starts with the
window.onload
function. It takes the timer value set by you and calculates the total duration of time in seconds.let time_minutes = 1; // Value in minutes let time_seconds = 30; // Value in seconds let duration = time_minutes * 60 + time_seconds;
-
document.querySelector('#count-down-timer')
gets the HTML node of the element. This node will be used to refresh the timer value.element = document.querySelector('#count-down-timer');
-
Once the element is available, it initializes with the value set by us. For instance, in the code, it is 1 minute and 30 seconds. It is set in a padded format.
function paddedFormat(num) { return num < 10 ? '0' + num : num; } console.log(paddedFormat(3)); console.log(paddedFormat(12));
Output:
03 12
-
The
paddedFormat(num)
function returns the padded number. For minutes and seconds, the single-digit values are padded by prefixing a0
to them. For example, 2 minutes and 5 seconds is padded as 02 and 05, respectively.element.textContent = `${paddedFormat(time_minutes)}:${paddedFormat(time_seconds)}`;
-
Next, we invoke the
startCountDown(--duration, element)
function passingduration
in seconds, and the HTML node of the display element. We displayed the value01:30
now. Hence, we need the timer to start from01:29
. That is the reason why we use the decrement unary operator with duration--duration
.startCountDown(--duration, element);
-
startCountDown(duration, element)
is the heart of the timer. In this, we use thesetInterval(function(){}, 1000)
function of JavaScript to execute the timer after each second (1000 millisecond) until it is cleared off or killed by usingclearInterval(countInterval)
.function startCountDown(duration, element) { let secondsRemaining = duration; let min = 0; let sec = 0; let countInterval = setInterval(function() { min = parseInt(secondsRemaining / 60); sec = parseInt(secondsRemaining % 60); element.textContent = `${paddedFormat(min)}:${paddedFormat(sec)}`; secondsRemaining = secondsRemaining - 1; if (secondsRemaining < 0) { clearInterval(countInterval) }; }, 1000); }
-
Inside the
setinterval()
function, the minutes and seconds for display are calculated. The minutes are calculated by dividing theremainingSeconds
value by 60 to give the minute value and taking its integer part.let secondsRemaining = 89; let min = parseInt(secondsRemaining / 60); let sec = parseInt(secondsRemaining % 60); console.log(secondsRemaining + 'seconds'); console.log(min); console.log(sec);
Output:
89 // Equivalent to 1 min and 29 seconds 1 29
-
Next, we display the minutes and seconds calculated to the webpage by setting the text using the
textContent
attribute of the HTML node element.element.textContent = `${paddedFormat(min)}:${paddedFormat(sec)}`;
-
Next, the value reduces for the
secondsRemaining
, and the timer functionality executes each second with thesetInterval()
method. -
Finally, once the
secondsRemaining
value is 0, theclearInterval(countInterval)
is triggered to stop the timer. It also ensures the timer is not restarted or goes in minus values.