Timer in JavaScript
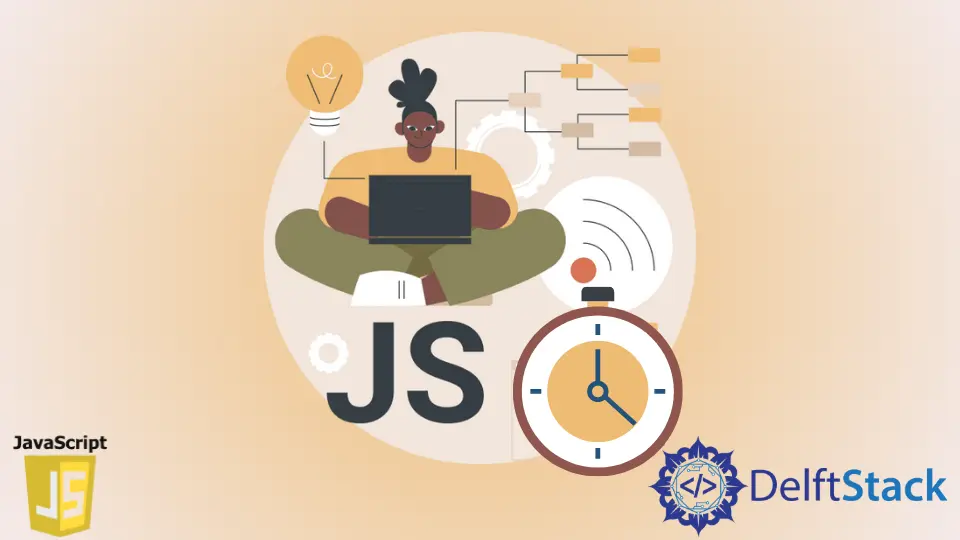
JavaScript provides two timer functions for executing non-blocking code. JavaScript provides the functions setTimeout()
and setInterval()
, which execute the specified expression or function after a certain time interval.
In today’s post, we are going to learn more about the JavaScript timer.
Create a Timer With setTimeout()
in JavaScript
This is an asynchronous function provided by JavaScript that executes a specific function or section of code after the timer expires. Various reasons cause timeouts to be delayed, like nested timeouts, timeouts in inactive tabs, throttling of tracking scripts, and deferral of timeouts during page load.
Syntax for setTimeout()
in JavaScript
setTimeout(function [, delay, arg1, arg2, ...]);
setTimeout(code[, delay]);
Parameter
function
: It is a mandatory parameter. It specifies the function that should be executed after the time has elapsed.code
: It is a mandatory parameter. If the user does not pass the function, the user can pass a character string, an alternative to the function.delay
: This is an optional parameter. This parameter accepts the numeric value as a timer in milliseconds before executing the specified code or function. If no value is passed, the default value is0
, which causes execution to occur immediately.arg1, ..., argN
: This is an optional parameter. If the function is passed, the values of the function can be passed as an additional argument.
Return Value
This setTimeout()
method returns a positive timeoutID
which helps to identify the timer. This value can be used to clearTimeout()
.
Example code:
<button onclick="setTimeoutFunction()">Try set Timeout</button>
function addFn(a, b) {
console.log(a + b);
return a + b;
}
function setTimeoutFunction() {
setTimeout(addFn, 5000, 5, 10);
}
Output:
15
Create a Timer With setInterval()
in JavaScript
The setInterval()
method is provided by JavaScript. This method is offered on the worker
and the window
interfaces. It repeatedly calls a function or executes a section of code. This code is executed at a fixed time delay between each call.
The sole difference between the setInterval()
and the setTimeout()
functions is that setInterval()
calls the function repeatedly with a delay between each call whereas setTimeout()
executes a function after a delay.
Syntax for setTimeout()
in JavaScript
setInterval(function [, delay, arg1, arg2, ...]);
Parameter
function
: It is a mandatory parameter. It specifies the function that should be executed after the time has elapsed.code
: It is a mandatory parameter. If the user does not pass the function, the user can pass a string alternative to the function.delay
: This is an optional parameter. This parameter accepts the numeric value that serves as the timer in milliseconds before executing the specified code or function. If no value is passed,0
is the default, which causes execution to occur immediately.arg1, ..., argN
: This is an optional parameter. If the function is passed, the values of the function can be passed as an additional argument.
Return Value
This setInterval()
method returns a positive intervalID
which helps to identify the timer. This value can be used to clearInterval()
.
Example code:
<button onclick="setIntervalFunction()">Try set Interval</button>
function addFn(a, b) {
console.log(a + b);
return a + b;
}
function setIntervalFunction() {
setInterval(addFn, 5000, 5, 10);
}
Output:
15
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn