How to Convert Date to UTC in JavaScript
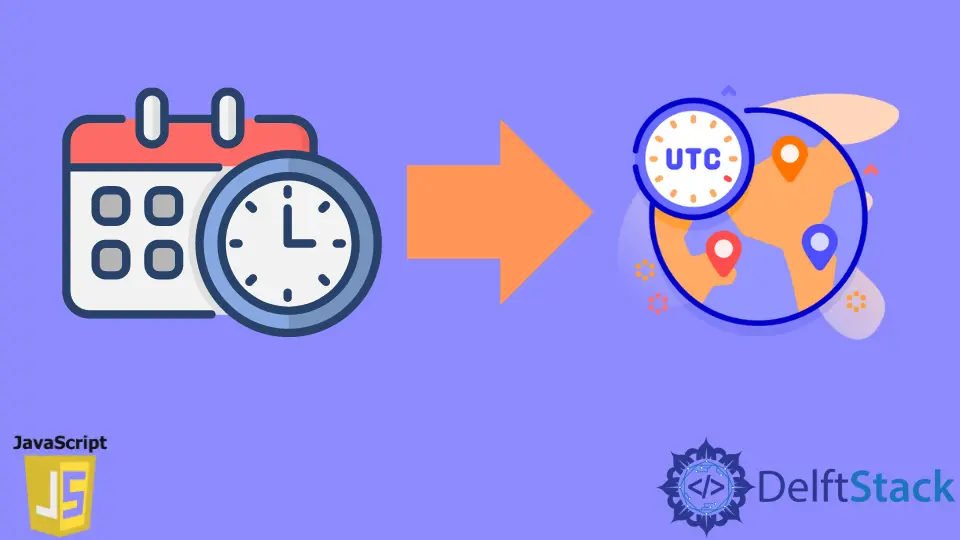
This tutorial will discuss how to convert date to UTC format using the toISOString()
function in JavaScript.
Convert Date to UTC Format Using the toISOString()
Function in JavaScript
First of all, let’s talk about how to create a date object in JavaScript. To make this object type, we can use the Date()
function, which returns the current date, time, GMT offset, and time zone.
For example, let’s create a date object and check the current date using the Date()
function. See the code below.
var myDate = new Date();
console.log(myDate);
Output:
Sun Jun 20 2021 10:13:09 GMT+0500 (Pakistan Standard Time)
In the output, we can see that the full date, time, offset, and time zone are returned. If we only want to extract only one element from the date object, we can use the get
property. For example, let’s extract the month from the above date object using the getUTCDate()
property. See the code below.
var myDate = new Date();
var month = myDate.getUTCMonth();
console.log(month);
Output:
5
As you can see in the output, 5
means the current month is June.
There are other properties that you might use to get your desired part like getFullYear()
, getDate()
, getHours()
, and getSeconds()
. If you want to make a date object with your desired date, you must pass the desired date in the Date()
function.
For example, let’s create a date object with our desired date in the Date()
function. See the code below.
var myDate = new Date(1990, 05, 10);
console.log(myDate);
Output:
Sun Jun 10 1990 00:00:00 GMT+0500 (Pakistan Standard Time)
In the output, the time is zero, but you can also set the time by passing it in the Date()
function. There are many formats to pass the date in the Date()
function; some are listed below.
var myDate = new Date(year, month, day, hours, minutes, seconds, milliseconds);
var myDate = new Date('YYYY-MM-DD');
var myDate = new Date('MM/DD/YYYY');
For example, let’s create a time object using the third format. See the code below.
var myDate = new Date('10/5/1990');
console.log(myDate);
Output:
Fri Oct 05 1990 00:00:00 GMT+0500 (Pakistan Standard Time)
Now let’s convert the current date to UTC using the toISOString()
function. See the code below.
var myDate = new Date().toISOString();
console.log(myDate);
Output:
2021-06-20T06:09:09.043Z
In the output, we observe that the date is in UTC format. Note that your output might change depending on your date.
Related Article - JavaScript Date
- How to Add Months to a Date in JavaScript
- How to Add Minutes to Date in JavaScript
- How to Add Hours to Date Object in JavaScript
- How to Calculate Date Difference in JavaScript
- How to Calculate Age Given the Birth Date in YYYY-MM-DD Format in JavaScript
- How to Get First and Last Day of Month Using JavaScript