How to Close the Browser Tab in a Browser Window
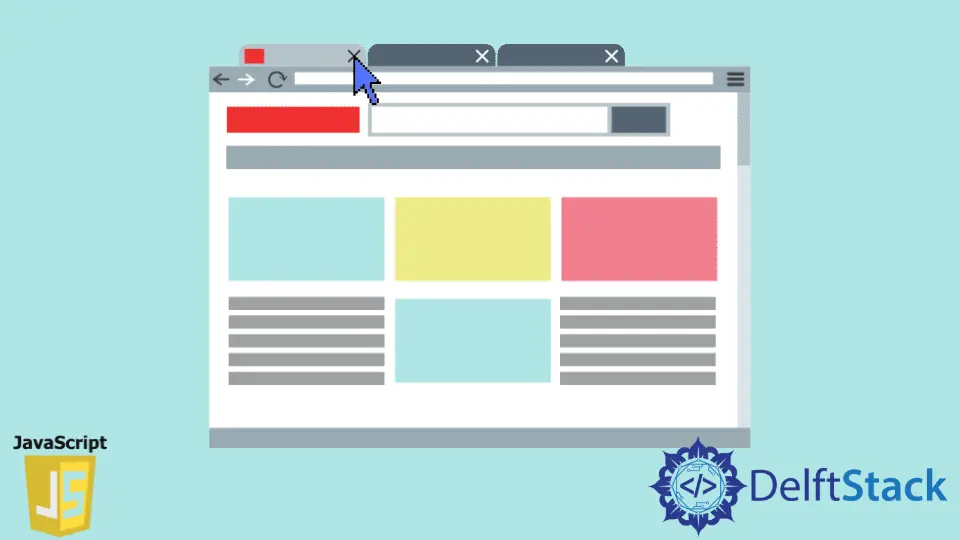
There are some specific situations where closing a browser tab can be necessary and essential, especially if you are developing websites that support payment systems (of course, there can be other possible use cases). During an online transaction, a session is created, and various things happen in the background; and during that time, the browser’s job is to display the response coming from the server so that the client or user can see what’s exactly happens.
After the transaction is completed, we are supposed to close the session currently happening inside that particular browser tab so that no misuse of personal information could occur. So, it could be done by closing that particular browser tab.
You might have seen this scenario, where you do the transaction online via the bank’s portal, and then after you log out from the net banking account, the bank displays a text on the screen which tells us to close the browser window. Of course, you can use the close button in the browser, or you can also create a close button and ask the user to click on it. Let’s see how we can achieve this in detail.
Close Browser Tab Using window.close()
Method in JavaScript
To close a browser tab, JavaScript provides us with a method associated with a window object, and that method is called window.close()
. Using this method, we can easily achieve our goal of closing a browser tab. You can also directly use the close()
method without using the keyword window
as well. This method is supported on all modern browsers like Chrome, Brave, and Firefox.
So, Let’s see how to implement this method in JavaScript and close the browser tab. Inside our HTML document, we have a body tag that contains an anchor tag and a script tag. The script will be used to link our JavaScript file with the HTML document. The anchor tag will represent the button using which we will perform the browser tab closing operation. The styling for this anchor tag, our button, is done inside the style
tag. This anchor tag points to the function close_tab()
, which is present inside our script file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body{
margin-top: 50px;
margin-left: 50px;
}
a{
width: 100px;
height: 50px;
background-color: brown;
color: #ffffff;
text-decoration: none;
padding: 10px;
}
</style>
</head>
<body>
<!-- This is a button -->
<a href="javascript:close_tab();">close browser tab</a>
<script src="script.js"></script>
</body>
</html>
Inside our script.js
file, we have a function called close_tab()
, which will be executed only when the user clicks on the close browser tab
button present in our HTML document.
function close_tab() {
if (confirm('Do you want to close this tab?')) {
window.close();
}
}
Here, we will also use another method known as confirm()
, which takes a string as a parameter. This method will show a popup or alert box with the string which we have passed to the confirm()
as a parameter, and it will also have Ok
and Cancel
buttons. And we are calling this method inside the if
statement is because this function will return either true
or false
values.
So, if the user pressed the cancel
button, then the confirm()
function will return false
. If the user presses the Ok
button, then the function will return true
. If the close()
function returns false
then our if
block will not be executed and if it returned true
then only our if
block will be executed and then our function window.close()
will execute. It will then close the browser tab.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn