How to Check if a Variable Is String in JavaScript
- Method 1: Using the typeof Operator
- Method 2: Using the instanceof Operator
- Method 3: Using Object.prototype.toString
- Method 4: Using the String.prototype.isPrototypeOf
- Conclusion
- FAQ
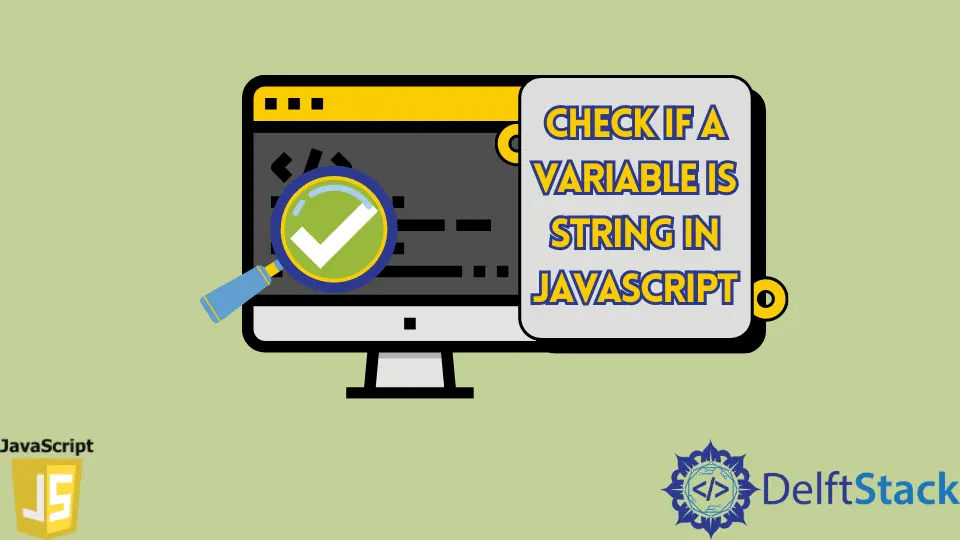
JavaScript is a versatile programming language that allows developers to manipulate data in various ways. One common task is determining whether a variable is a string. Understanding how to check if a variable is a string is crucial for data validation and ensuring that your code behaves as expected.
In this tutorial, we will explore different methods to check if a variable is a string in JavaScript. Whether you’re a beginner or an experienced developer, this guide will provide you with clear, practical examples to enhance your JavaScript skills. Let’s dive into the world of strings and learn how to effectively identify them in your code!
Method 1: Using the typeof Operator
The typeof
operator is one of the simplest and most commonly used methods to check if a variable is a string in JavaScript. This operator returns a string indicating the type of the unevaluated operand. When applied to a string variable, it will return “string”.
Here’s how you can use it:
let variable1 = "Hello, World!";
let variable2 = 42;
console.log(typeof variable1 === 'string'); // true
console.log(typeof variable2 === 'string'); // false
Output:
true
false
In this example, we declared two variables: variable1
, which is a string, and variable2
, which is a number. By using the typeof
operator, we can check the type of each variable. The first console.log
returns true because variable1
is indeed a string, while the second returns false since variable2
is not a string. This method is straightforward and effective, making it a popular choice among developers.
Method 2: Using the instanceof Operator
Another way to check if a variable is a string is by using the instanceof
operator. This operator tests whether an object is an instance of a specific constructor. In the case of strings, we can check if a variable is an instance of the String
constructor.
Here’s a practical example:
let variable1 = new String("Hello, World!");
let variable2 = "Hello, World!";
let variable3 = 42;
console.log(variable1 instanceof String); // true
console.log(variable2 instanceof String); // false
console.log(variable3 instanceof String); // false
Output:
true
false
false
In this code, we create variable1
using the String
constructor, which makes it an object. The instanceof
operator returns true for variable1
, indicating that it is indeed an instance of String
. However, for variable2
, which is a primitive string, the result is false. This method is particularly useful when working with string objects, but it’s essential to remember that primitive strings will not pass this check.
Method 3: Using Object.prototype.toString
A more robust method to check if a variable is a string is by using Object.prototype.toString.call()
. This technique returns a string that represents the type of the object. It can be especially handy when dealing with different types of objects.
Here’s how to implement it:
let variable1 = "Hello, World!";
let variable2 = new String("Hello, World!");
let variable3 = 42;
console.log(Object.prototype.toString.call(variable1) === '[object String]'); // true
console.log(Object.prototype.toString.call(variable2) === '[object String]'); // true
console.log(Object.prototype.toString.call(variable3) === '[object String]'); // false
Output:
true
true
false
In this example, both variable1
and variable2
return true because they are recognized as strings, whether as a primitive or an object. variable3
, however, returns false since it is a number. This method is particularly powerful because it can accurately identify strings regardless of how they are created, making it a reliable choice for type checking.
Method 4: Using the String.prototype.isPrototypeOf
You can also determine if a variable is a string by checking if String.prototype
is in its prototype chain. This method can be useful for ensuring that a variable is a string object.
Here’s how to apply it:
let variable1 = new String("Hello, World!");
let variable2 = "Hello, World!";
let variable3 = 42;
console.log(String.prototype.isPrototypeOf(variable1)); // true
console.log(String.prototype.isPrototypeOf(variable2)); // false
console.log(String.prototype.isPrototypeOf(variable3)); // false
Output:
true
false
false
In this code snippet, String.prototype.isPrototypeOf(variable1)
returns true because variable1
is a string object created with the String
constructor. However, for variable2
, which is a primitive string, it returns false. This method is particularly useful when you want to ensure that the variable is a string object.
Conclusion
In conclusion, checking if a variable is a string in JavaScript can be achieved through several methods, including the typeof
operator, instanceof
, Object.prototype.toString
, and String.prototype.isPrototypeOf
. Each method has its advantages and use cases, so understanding them can help you write more robust and error-free code. Whether you’re validating user input or processing data, knowing how to identify strings is an essential skill for any JavaScript developer. Armed with these techniques, you’re now ready to tackle string validation like a pro!
FAQ
-
How do I check if a variable is a string in JavaScript?
You can use thetypeof
operator,instanceof
, orObject.prototype.toString
methods to check if a variable is a string. -
What is the difference between primitive strings and String objects?
Primitive strings are simple string literals, while String objects are created using the String constructor. They behave differently in type checks. -
Can I use the
instanceof
operator with primitive strings?
No, theinstanceof
operator will return false for primitive strings. It only returns true for String objects. -
Is
Object.prototype.toString
method reliable for type checking?
Yes, it is a very reliable method for checking types, as it works for both primitive types and objects.
- Which method is the best for checking strings?
The best method depends on your specific use case, buttypeof
is the simplest for most scenarios.