How to Change Date Format in JavaScript
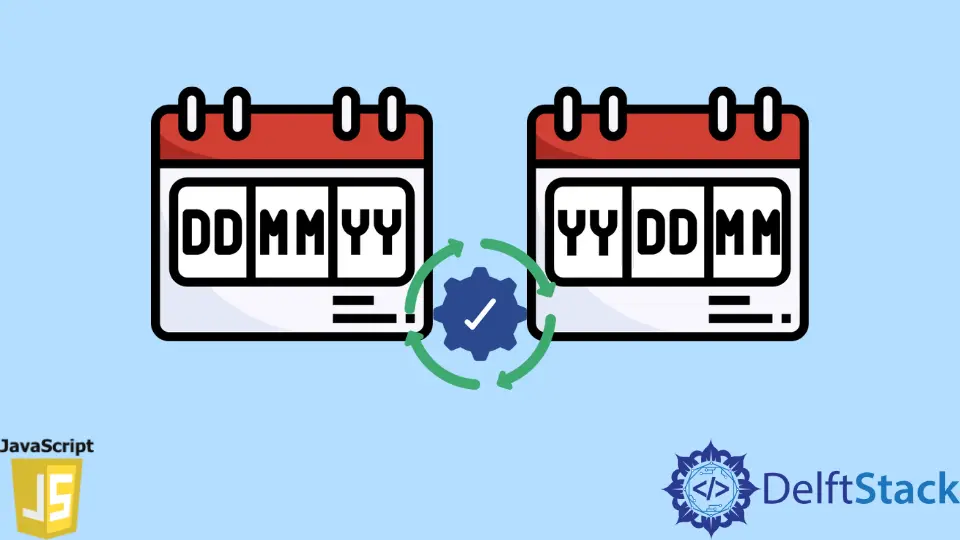
Determining the current time/moment is an important part of every application. JavaScript supports a Date
object that represents a single point in time. Date objects contain a number representing milliseconds since January 1, 1970 UTC, also known as milliseconds since the Unix epoch. JavaScript provides various ways to get a date in several formats.
Change Date Format Using Date()
in JavaScript
This is a function built into JavaScript that returns the formatted date string. When called new Date()
, it acts as a constructor and returns the Date object instead of a formatted string. It also offers various static methods like
Date.now()
Date.parse()
Date.UTC()
The Date object also supports instance methods like
Date.prototype.getDate()
: This method will return the day of the month in the range of 1-31 for the given date according to local time.Date.prototype.getMonth()
: This method will return the month in the range of 0-11 on the given date according to local time. The month will start from 0.Date.prototype.getYear()
: This method will return the year on the given date according to local time. It returns the year usually 2-3 digits.Date.prototype.getFullYear()
: This method will return the year as 4 digits for 4-digit years of the given date according to local time.
Syntax of Date()
in JavaScript
Date();
Example code:
const today = new Date();
const month = [
'January', 'February', 'March', 'April', 'May', 'June', 'July', 'August',
'September', 'October', 'November', 'December'
];
const str =
today.getDate() + ' ' + month[today.getMonth()] + ' ' + today.getFullYear();
console.log(str);
Output:
18 November 2021
The built-in Date object above allows you to change the date format to suit your needs. You can also use an external library like DateJS
.
Change Date Format Using DateJS
in JavaScript
JavaScript has an open-source Date library called DateJS
for formatting, parsing, and processing. Learn how to import DateJS
here.
Syntax of Date.parse()
in DateJS
Date.parse($string);
Parameter of Date.parse()
$string
: It is a mandatory parameter. This field accepts any date string such astoday
,tomorrow
,last Sunday
,July 4th
,t + 3d
, etc. See the official document for more information.
Example code:
const parsedDate = Date.parse('tomorrow');
console.log(parsedDate);
Output:
Fri Nov 19 2021
Syntax of Date.toString()
in DateJS
Date.parse($string);
Parameter of Date.toString()
$string
: It is an optional parameter. This field accepts any date string likeM/d/yyyy
,d-MMM-yyyy
,HH:mm
,MMMM dS, yyyy
, etc. Default, native JavaScriptDate.toString()
function will be called if no format is provided.
Example code:
const parsedDate = Date.today().toString('MMMM dS, yyyy');
console.log(parsedDate);
Output:
November 18th, 2021
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedInRelated Article - JavaScript Date
- How to Add Months to a Date in JavaScript
- How to Add Minutes to Date in JavaScript
- How to Add Hours to Date Object in JavaScript
- How to Calculate Date Difference in JavaScript
- How to Calculate Age Given the Birth Date in YYYY-MM-DD Format in JavaScript
- How to Get First and Last Day of Month Using JavaScript