How to Import Function From Another JS File
- Import JavaScript Functions From Another File Using the CommonJS Approach in Node.js
- Import JavaScript Functions From Another File Using ECMAScript Modules (ES6 Modules)
- Import JavaScript Function From Another File Using jQuery
- Conclusion
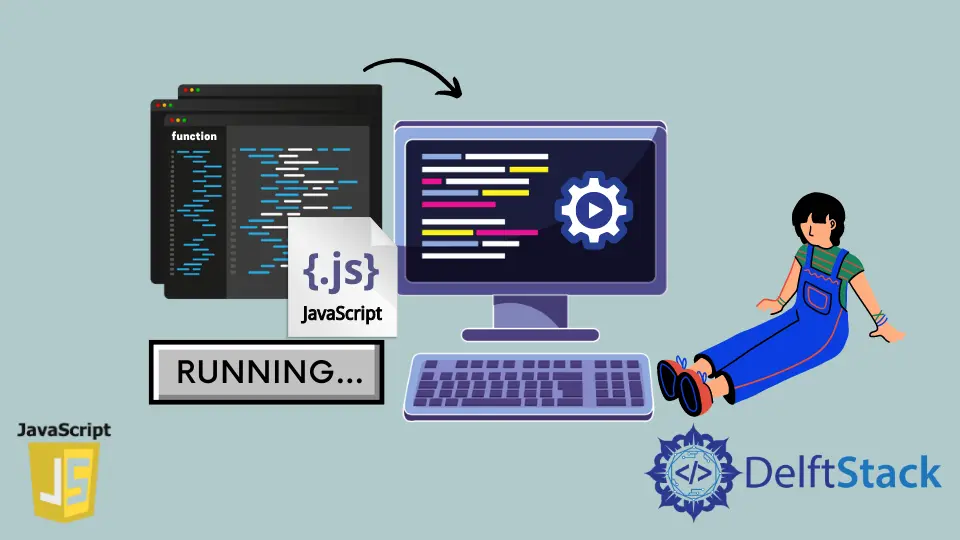
In this article, we’ll explore various methods for achieving this, covering both the CommonJS approach, commonly used in Node.js, and the ECMAScript Modules (ES6 Modules) approach, widely used in modern web development.
Import JavaScript Functions From Another File Using the CommonJS Approach in Node.js
In Node.js, a popular runtime for server-side JavaScript, the CommonJS module system is widely used for managing dependencies and structuring code.
CommonJS is a module system for JavaScript that enables developers to split their code into reusable modules. Each module encapsulates its scope, preventing the pollution of the global namespace. Node.js, built on the V8 JavaScript engine, supports CommonJS modules, making it easy to structure and organize code.
A CommonJS module typically consists of the following elements:
require
Function: Used to import JavaScript modules.module.exports
Object: Used to expose functionality from a module.
Creating a Simple Module
Let’s start by creating a simple JavaScript module that contains a function we want to import into another JS file. Create a new file, let’s say mathUtils.js
, with the following code:
// mathUtils.js
function add(a, b) {
return a + b;
}
module.exports = add;
In this example, the mathUtils.js
file defines a function called add
that takes two parameters and returns their sum. The module.exports
statement is used to make the add
function available for use in other files.
Importing the Function
Now, let’s create another file, say main.js
, where we want to import and use the add
function from mathUtils.js
:
// main.js
// Require the 'add' function from 'mathUtils.js'
const addFunction = require('./mathUtils');
// Use the 'add' function
const result = addFunction(5, 3);
console.log('Result:', result);
In this first JS import file, the require
function is used to import the add
function from mathUtils.js
. The path to the module is specified using a relative path (in this case, ./mathUtils
). Once imported, the add
function can be used as if it were defined in the main.js
file.
To run this, open your terminal, navigate to the directory containing both mathUtils.js
and main.js
, and execute the script with the following command:
node main.js
If everything is set up correctly, you should see the following console output:
This result is obtained by calling the add function with arguments 5 and 3.
Extending Functionality with Multiple Functions
Now that we’ve covered the basics of importing a single function let’s explore how to import multiple functions from a module. We can extend our mathUtils.js
module to include additional functions and then import and use them in the main.js
file.
// mathUtils.js
function add(a, b) {
return a + b;
}
function subtract(a, b) {
return a - b;
}
module.exports = {
add,
subtract
};
In this updated version, the mathUtils.js
file now exports both the add
and subtract
functions as properties of an object assigned to module.exports
.
Importing Multiple Functions
Now, in the main.js
file, we can import and use both the add
and subtract
functions:
// main.js
const mathUtils = require('./mathUtils');
const sumResult = mathUtils.add(5, 3);
// Use the 'subtract' function
const differenceResult = mathUtils.subtract(8, 3);
console.log('Sum Result:', sumResult);
console.log('Difference Result:', differenceResult);
Here, the entire mathUtils.js
module is imported, and then we can access its functions as properties of the imported object (mathUtils.add
and mathUtils.subtract
).
You should see the following output:
Handling Default Exports
In addition to named exports, CommonJS also supports default exports. Let’s explore how to use default exports to further enhance our modular approach.
// mathUtils.js
function add(a, b) {
return a + b;
}
function subtract(a, b) {
return a - b;
}
// Export the 'add' function as the default export
module.exports = add;
// Export the 'subtract' function as a named export
module.exports.subtract = subtract;
In this updated version, the add
function is set as the default export, while the subtract
function is exported as a named export.
Importing with Default Export
Now, in the main.js
file, we can import the default export and the named export separately:
// main.js
// Require the default export ('add' function)
const addFunction = require('./mathUtils');
// Require the named export ('subtract' function)
const subtractFunction = require('./mathUtils').subtract;
// Use the 'add' function
const sumResult = addFunction(5, 3);
// Use the 'subtract' function
const differenceResult = subtractFunction(8, 3);
console.log('Sum Result:', sumResult);
console.log('Difference Result:', differenceResult);
By using the default export and named exports method together, you can achieve a flexible and expressive module structure.
The output should be the same as before:
Utilizing default exports can make importing a module more concise, especially when you primarily use one main function from a module. This approach enhances code readability and simplifies the import
statement.
Import JavaScript Functions From Another File Using ECMAScript Modules (ES6 Modules)
Another way to organize and manage code efficiently is by using ECMAScript modules, also known as ES6 modules, which provide a modular approach to structuring JavaScript code. Unlike the traditional approach of using scripts with global scope, modules encapsulate their variables and functions, preventing them from interfering with other parts of the code.
To get started, ensure that your JavaScript files have the .mjs
extension to indicate that they are module files. Note that the use of modules is supported in modern browsers and Node.js versions that support ECMAScript modules.
Creating a Module
Let’s begin by creating a simple module with a function that we want to import into another JS file. Define the function with the export
statement:
// mathFunctions.mjs
// use the export keyword
export function addNumbers(a, b) {
return a + b;
}
export function multiplyNumbers(a, b) {
return a * b;
}
In the example above, we have created a module named mathFunctions.mjs
that exports two functions: addNumbers
and multiplyNumbers
.
Importing Functions in Another File
Now, let’s create another JS file where we will import and use the functions defined in our module. Use the import
statement to bring in the function:
// main.mjs
// Use import keyword to import function from another JavaScript file
import { addNumbers, multiplyNumbers } from './mathFunctions.mjs';
const sum = addNumbers(5, 3);
const product = multiplyNumbers(4, 2);
console.log(`Sum: ${sum}`);
console.log(`Product: ${product}`);
In the main.mjs
file, we use the import
statement to bring in specific functions from the mathFunctions.mjs
module. The syntax { addNumbers, multiplyNumbers }
allows us to import only the functions we need.
To run the code, you can use a tool like Node.js. Ensure that you have a version that supports ECMAScript modules.
Execute the following commands in your terminal:
node --experimental-specifier-resolution=node main.mjs
This command tells Node.js to run the main.mjs
file using the experimental specifier resolution for ECMAScript modules.
Output:
Handling Default Exports
In addition to named exports, ECMAScript modules also support default exports. A module can have at most one default export, and it is imported without using curly braces.
Let’s modify our mathFunctions.mjs
module to include a default export:
// mathFunctions.mjs
// Named exports
export function addNumbers(a, b) {
return a + b;
}
export function multiplyNumbers(a, b) {
return a * b;
}
// Default export
export default function squareNumber(a) {
return a * a;
}
Now, let’s update the main.mjs
file to import the default export:
// main.mjs
// Import named exports and the default export from the mathFunctions module
import { addNumbers, multiplyNumbers } from './mathFunctions.mjs';
import squareNumber from './mathFunctions.mjs'; // Note: No curly braces for the default export
// Use the imported functions from another JavaScript file
const sum = addNumbers(5, 3);
const product = multiplyNumbers(4, 2);
const square = squareNumber(3);
console.log(`Sum: ${sum}`);
console.log(`Product: ${product}`);
console.log(`Square: ${square}`)
By including a default export in the mathFunctions.mjs
module, we can import it in the main.mjs
file using the import squareNumber from './mathFunctions.mjs';
syntax. The absence of curly braces around the imported value indicates that we are importing the default export.
Output:
Note: While modern browsers generally support ECMAScript modules, it’s important to be aware of potential compatibility issues. Check the compatibility table on platforms like MDN Web Docs to ensure that your target browsers fully support the module syntax.
Importing the Entire Module
If you want to import all exports from a module, you can use the * as
syntax. This approach is suitable when you need most or all of the exports from a module:
// main.mjs
// Use import statement to import all the exports from mathFunctions
import * as mathFunctions from './mathFunctions.mjs';
// Use the imported functions
const resultSum = mathFunctions.addNumbers(5, 3);
const resultProduct = mathFunctions.multiplyNumbers(4, 2);
const resultSquare = mathFunctions.default(3);
console.log(`Sum: ${resultSum}`);
console.log(`Product: ${resultProduct}`);
console.log(`Square: ${resultSquare}`);
In this example, mathFunctions
is an object containing all exports from the mathFunctions.mjs
module.
Output:
Dynamic Imports
Dynamic imports allow you to load JavaScript modules asynchronously, which can be beneficial for performance optimization, especially in web applications. The import()
function returns a Promise that resolves to the module’s namespace object.
Here’s a basic example:
// main.mjs
// Dynamically import and load modules
const mathModule = import('./mathFunctions.mjs');
// Use the imported functions after the Promise resolves
mathModule.then((mathFunctions) => {
const resultSum = mathFunctions.addNumbers(5, 3);
const resultProduct = mathFunctions.multiplyNumbers(4, 2);
const resultSquare = mathFunctions.default(3);
console.log(`Sum: ${resultSum}`);
console.log(`Product: ${resultProduct}`);
console.log(`Square: ${resultSquare}`);
});
Dynamic imports can be especially useful when you only need a module under certain conditions or when the module is not critical for the initial loading of the application.
Output:
Cross-Origin Module Loading
You might encounter scenarios where you need to load modules from a different origin (cross-origin). To achieve this, you can use the import()
function with the import.meta.url
property.
Take a look at the code below:
// main.mjs
// Dynamically import a module from a different origin
const externalModule = import(new URL('https://example.com/external-module.mjs'));
// Use the imported functions after the Promise resolves
externalModule.then((module) => {
// Do something with the imported module
});
Keep in mind that cross-origin module loading might require the server to send appropriate Cross-Origin Resource Sharing (CORS) headers.
Import JavaScript Function From Another File Using jQuery
jQuery simplifies the process of interacting with the Document Object Model (DOM) and provides utility functions for common tasks. While jQuery itself doesn’t have a built-in module system like CommonJS, it’s still possible to create modular code by leveraging jQuery’s ability to handle asynchronous tasks and load scripts dynamically.
Creating a Simple Module
Let’s start by creating a simple JavaScript module that contains a function to be imported into another file. Create a new JavaScript file, say mathUtils.js
, with the following content:
// mathUtils.js
// Function to add two numbers
function add(a, b) {
return a + b;
}
// Expose the 'add' function globally
window.mathUtils = {
add: add
};
In this example, the mathUtils.js
file defines an add
function and exposes it globally using the window
object. This allows us to access the function from other JS files.
Function Import With jQuery
Now, let’s write another JavaScript file, say main.js
, where we want to import and use the add
function from mathUtils.js
using jQuery:
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>jQuery Import Example</title>
<!-- Include jQuery -->
<script src="https://code.jquery.com/jquery-3.6.4.min.js"></script>
<!-- Include the mathUtils.js JavaScript file -->
<script src="mathUtils.js"></script>
<!-- Include the main.js JavaScript file -->
<script src="main.js"></script>
</head>
<body>
<!-- Your HTML file content here -->
</body>
</html>
In this HTML document, we include the jQuery library and the mathUtils.js
and main.js
files using <script>
tags. The order of inclusion is important, as main.js
should be loaded after mathUtils.js
.
Now, let’s look at the content of the main.js
file:
// main.js
// Use the 'add' function from mathUtils.js
const result = mathUtils.add(5, 3);
$(document).ready(function() {
$('body').append('<p>Result: ' + result + '</p>');
});
In this JavaScript file, the add
function from mathUtils.js
is used, and the result is displayed in the HTML body using jQuery.
To run the code, open the index.html
file in a web browser:
You should see the result displayed on the web page, indicating that the add
function from mathUtils.js
has been successfully imported and used.
Handling Asynchronous Loading
In a real-world scenario, your application may require the loading of JavaScript files asynchronously, especially when dealing with larger projects or when dependencies need to be loaded on demand. jQuery provides a convenient method for handling asynchronous loading through the $.getScript()
function.
Let’s extend our example to demonstrate how to asynchronously load the mathUtils.js
module in the main.js
file:
// main.js
// Use the 'add' function from mathUtils.js
const result = mathUtils.add(5, 3);
// Display the result using jQuery
$(document).ready(function() {
$('body').append('<p>Result: ' + result + '</p>');
// Asynchronously load mathUtils.js
$.getScript('mathUtils.js', function() {
// Use the 'add' function again after the script is loaded
const asyncResult = mathUtils.add(8, 2);
$('body').append('<p>Async Result: ' + asyncResult + '</p>');
});
});
In this updated main.js
file, the $.getScript()
function is used to asynchronously load the mathUtils.js
script. The provided callback function is executed once the script is loaded, allowing us to use the add
function again in an asynchronous context.
Output:
Conclusion
Importing JavaScript functions from one file to another is crucial for maintaining a clean and modular codebase. Whether you opt for the CommonJS approach, commonly used in Node.js, or the ECMAScript modules approach, favored in modern web development, understanding these methods is essential.
Choose the approach that aligns with your project’s environment and enjoy the benefits of modular, organized, and maintainable code.