How JavaScript Export Class in JavaScript
-
Types of
export
Statements in JavaScript -
Example on
Default
Export in JavaScript -
Example on
Named
Export in JavaScript
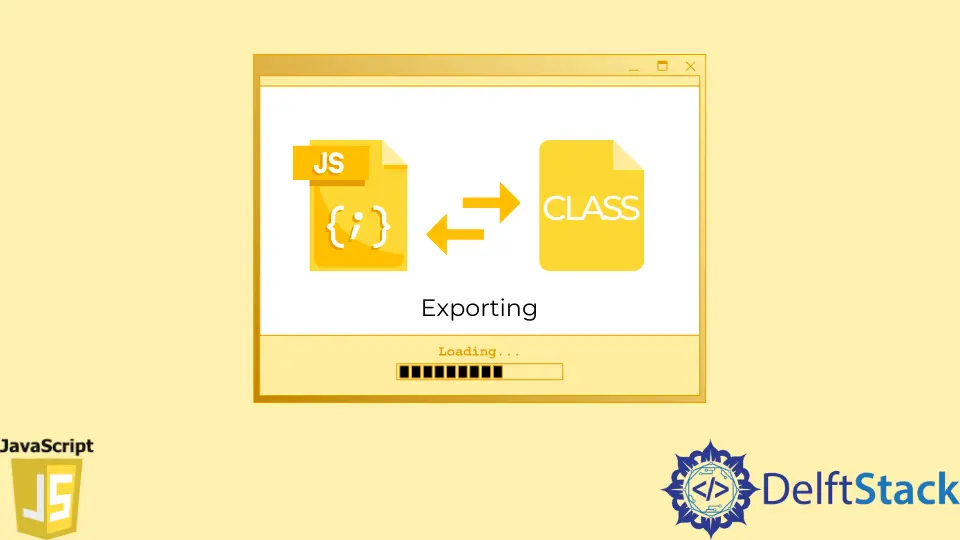
JavaScript export/import statements apply when a JavaScript module’s content is reused in another module.
There can be many ways of performing the task, from a single module to module transfer of detail and from children module to parent module for aggregating.
Types of export
Statements in JavaScript
The export
/import
statement in JavaScript is of two types. One is Named
export, and the other is Default
export.
Usually, when exporting in the Default
category, we do not use the curly braces to wrap the primitive or object. But in the case of a Named
export, we use curly braces to wrap multiple objects or primitives.
Default
only deals with one item export from a module, whereas the Named
can take zero or more than one.
We will need a file or module to follow up on the examples. And we will name them supposedly test.js
and New.js
.
But to be compatible with ES6, we will need to rename the modules as test.mjs
and New.mjs
. There are multiple ways to perform this conversion, but this is effortless.
Here we will see an instance with the Default
category and another with the Named
category. Let’s jump into the next section.
Example on Default
Export in JavaScript
In this example, we will initiate a class User
in test.mjs
, which we will export. The User
class only has the name of the favorite fruit.
Next, after declaring the constructor for the class, let’s go to the New.mjs
module and import the class. We will also trigger the exported module to work by setting some values.
Let’s check the example.
// test.mjs
export default class User {
constructor(fruit) {
this.fruit = fruit;
}
}
// New.mjs
import User from './test.mjs';
var x = new User('Mango');
console.log(x);
Output:
Example on Named
Export in JavaScript
We will take a function and a variable with dummy math for this instance. So, we will declare the export
statement before every data we want to export.
Later, in our parent module, New.mjs
, we will import and modify the value according to choice. A formal and declarative explanation is available in this thread.
Code Snippet:
// test.mjs
export function square(x) {
return x * x;
}
export var y = Math.PI * 5;
// New.mjs
import {square, y} from './test.mjs';
var x = 2;
console.log(square(x));
console.log(y);
Output: