How to Check Whether an Element Contains a Class in JavaScript
-
Use the Built-In
element.classList.contains()
Method to Check if an Element Contains a Class in JavaScript -
Use a Query Selector and the
Element.matches()
Method to Check if an Element Contains a Class in JavaScript -
Use
indexOf()
to Check if an Element Contains a Class in JavaScript
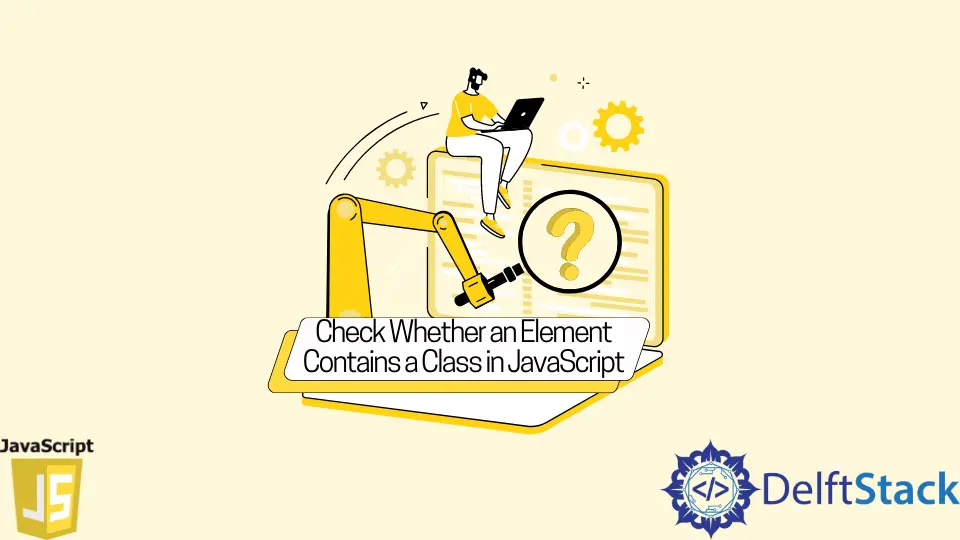
Using classes in HTML allows you to group elements and perform the same operations on all of them in terms of styling or functionality. As such, you can easily change the behavior of all the elements that share a class in a single line of code.
There are situations where you may want one element to be part of multiple classes. HTML allows you to do this, but there are certain rules you have to follow to avoid unexpected behavior.
In addition, selecting elements by class in JavaScript becomes a bit more tricky if they are tagged with multiple classes. Don’t worry; we can check if an element contains a specific class.
Use the Built-In element.classList.contains()
Method to Check if an Element Contains a Class in JavaScript
Unsurprisingly, this use case is common enough to have its native method in JavaScript, which should be your first option if no other special requirements are involved.
The gist of this method is to retrieve an element, iterate through all the classes it belongs to, and then check whether the class you give it as an argument is indeed part of the list. Here is how this works in practice:
<div id="mydiv" class="myclass1 myclass2">My Element</div>
let element = document.getElementById('mydiv');
console.log(element.classList.contains('myclass1'));
Output:
true
The JavaScript code above is compatible with all modern browsers, although some issues may arise in very old Internet Explorer versions.
Use a Query Selector and the Element.matches()
Method to Check if an Element Contains a Class in JavaScript
Query selectors are very powerful JavaScript tools, especially since they can usually do everything jQuery does, except you don’t need a third-party library.
In this case, the query selector allows you to select the element you want to perform the check on, while the Element.matches()
method allows you to yet again check if the class is present or not.
<div id="mydiv" class="myclass1 myclass2">My Element</div>
let element = document.querySelector('#mydiv');
console.log(element.matches('.myclass1'));
Output:
true
As you can see, the code required for both of these methods is more or less the same in terms of complexity and length. However, the query selector requires a more modern browser than the classList.contains()
method, so keep that in mind as well.
Use indexOf()
to Check if an Element Contains a Class in JavaScript
While modern browsers support both methods detailed above, you may not have that luxury. Thus, if your code has to be compatible with very old browser versions, you can also achieve the same result by using the indexOf()
method. Here is how it works:
<div id="mydiv" class="myclass1 myclass2">My Element</div>
let element = document.getElementById('mydiv');
function containsClass(element, className) {
return (' ' + element.className + ' ').indexOf(' ' + className + ' ') > -1;
}
console.log(containsClass(element, 'myclass1'));
Output:
true
Naturally, this method is more convoluted, even though the results are the same. In essence, we create our method called containsClass
, which iterates over the list of classes for our element.
If the class we are looking for is found, then it will have an index of 0 or greater, and the method will return true
.