How to Create a Class in JavaScript
- Classes in JavaScript
- Create a Class in JavaScript
- Declaration of a Method in a Class in JavaScript
-
Use of
get
andset
in Classes in JavaScript
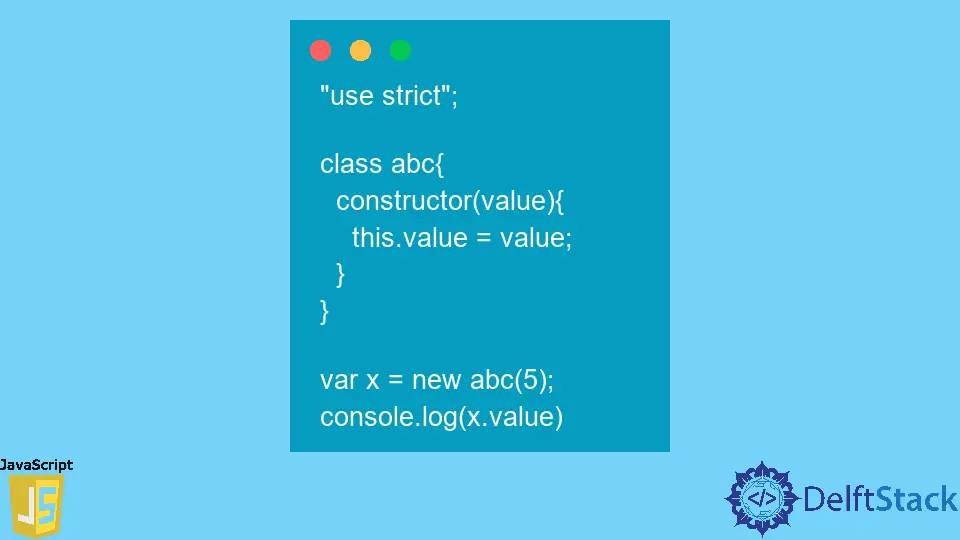
In JavaScript, we have the basic function declaration process to enable specific tasks, but functions do not go on with the concept of working to create an object. More like a function is an independent code block to perform a particular task.
Classes in JavaScript
What does the class do? The concept of class was introduced via the ES6 updates, which has an assertive approach to building something of value.
Suppose we are creating a game with multiple characters. The characters have a human-like figure, two eyes, two ears, two hands, etc.
Defining explicit functions for each character will cause a mess and unease of modifications. So, we make a class that will hold the common features of the figures; thus, a class works as a blueprint for an object.
Here, we will discuss the basic initialization of a class and demonstrate some significant ways it works. Also, some points to be noted: the class works in strict mode in JavaScript, so we will initiate that before our driver codes.
Another key point is that, unlike functions, we cannot call a class before it has been declared. In functions and methods, we can call the method first and later describe its detail.
But in classes, we will always have to describe the contents and declarations and then call them explicitly. Otherwise, we face a referenceError
.
So, let’s jump onto the code lines for a better preview of the topic.
Create a Class in JavaScript
We will always use the class
keyword to create a class in JavaScript. And after that, the name that will entitle the class will be defined.
In this example, we will take a class abc
with its constructor
method having the value
parameter. This implies that even if no other methods or expressions are declared, the class will always have this constructor as its content.
Constructors are like the default or the basic portion.
Later after the completion of the class and its constructor, we will initiate an instance or object for the class. And when we call the object along with the constructor parameter, it will result in the value that is the property of the class.
And thus, it implies that the object created under the class owns all the properties of that class abc
. Let’s check the code.
Code Snippet:
'use strict';
class abc {
constructor(value) {
this.value = value;
}
}
var x = new abc(5);
console.log(x.value)
Output:
Declaration of a Method in a Class in JavaScript
In the previous code example, we have seen how the constructor works. Other than that, we can also initiate private properties and basic methods.
In the case of private declarations, those cannot be accessed by the instances. But methods that are declared without an explicit declaration of private
can be accessed and altered by instances.
The example below supports the above statement.
Code Snippet:
'use strict';
class abc {
constructor(value) {
this.value = value
}
math() {
return this.value + 5
}
}
var x = new abc(5);
console.log(x.value);
console.log(x.math());
Output:
Use of get
and set
in Classes in JavaScript
The get
and set
keywords, often referred to as the getter and setter, are used to define functions more explicitly. Here, the setter part sets the value or operates any calculation based on the requirement.
Later, the getter returns the value to the class. This process makes the task more flexible.
Let’s visualize this concept with an example.
Code Snippet:
'use strict';
class abc {
constructor(val) {
this.val = val
}
get math() {
return this.value
}
set math(y) {
this.val = y + 10
}
}
var x = new abc()
x.math = 32
console.log(x.val)
Output: