JavaScript 从另一个 JS 文件调用函数
- 来自 HTML 文件的 JavaScript 调用函数
- JavaScript 调用函数从一个 JS 文件到另一个 JS 文件
-
使用 ES6
Import
和Export
的 JavaScript 调用函数 - 使用 jQuery 从一个 JS 文件到另一个 JS 文件的 JavaScript 调用函数
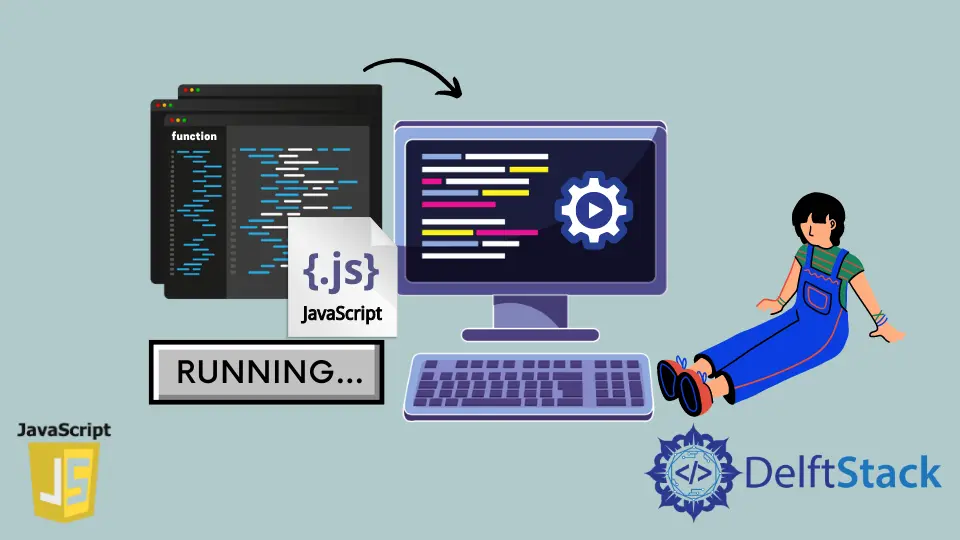
本课是关于从另一个 JS 文件调用 JavaScript 函数。它演示了如何使用本地机器和实时服务器调用 HTML 文件中的 JavaScript 函数以及从一个 JS 文件到另一个 JS 文件。
来自 HTML 文件的 JavaScript 调用函数
HTML 代码:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Function Call</title>
<script src="./first.js"></script>
</head>
<body>
<input
id="circleArea"
type="button"
value="Show Circle Area"
onclick="circleArea()"
/>
</body>
</html>
JavaScript 代码:
const PI = 3.14
let radius = 3;
function circleArea() {
var inputField = document.createElement('INPUT');
inputField.setAttribute('type', 'text');
inputField.setAttribute('value', (PI * radius * radius));
document.body.appendChild(inputField);
}
只要你单击类型 button
的 input
元素,circleArea()
函数就会运行。它计算圆形区域并将其显示在浏览器中。
createElement()
方法创建一个具有特定名称的元素节点。该名称可以是 INPUT
、BUTTON
、P
或其他名称。
我们在此示例代码中创建了一个 input
元素,并使用 setAttribute()
方法设置了两个属性(type
和 value
)。
我们将其 type
设置为 text
和 value
并带有一个圆形区域。appendChild()
函数附加一个新节点,其行为类似于节点的最后一个子节点。
JavaScript 调用函数从一个 JS 文件到另一个 JS 文件
HTML 代码:
<!DOCTYPE html>
<html>
<head>
<title>Call Function from One JS file into another</title>
<script src="first.js"></script>
<script src="second.js"></script>
</head>
<body>
<input
id="circleArea"
type="button"
value="Show Circle Area"
onclick="circleArea()"
/>
</body>
</html>
first.js
代码:
const PI = 3.14;
let radius = 3;
function calcArea() {
return PI * radius * radius;
}
second.js
代码:
function circleArea() {
document.write((calcArea()).toFixed(2));
}
我们再次计算圆的面积,但将 first.js
中的 calcArea
函数调用到 second.js
文件中。
document.write()
方法将输出写入浏览器,toFixed()
函数将数字转换为字符串,然后将其四舍五入为给定的小数位数。在这个例子中,我们将圆的面积打印到小数点后两位。
使用 ES6 Import
和 Export
的 JavaScript 调用函数
使用 ECMAScript6 (ES6),你可以使用 import
/export
功能。使用它,你可以导入/导出函数、变量、类。
HTML 代码:
<!DOCTYPE html>
<html>
<head>
<title>Call Function from One JS file into another</title>
<script type="module" src="./second.js"></script>
</head>
<body>
<button id="btn" onclick="circleArea()">Show Circle Area</button>
</body>
</html>
first.js
代码:
const PI = 3.14;
let radius = 3;
function calcArea() {
return PI * radius * radius;
}
export {calcArea};
second.js
代码:
import {calcArea} from './first.js';
document.getElementById('btn').addEventListener('click', function circleArea() {
var inputField = document.createElement('INPUT');
inputField.setAttribute('type', 'text');
inputField.setAttribute('value', (calcArea()).toFixed(2));
document.body.appendChild(inputField);
});
此示例代码使用 export {calcArea};
从 first.js
文件中导出 calcArea()
函数。second.js
文件首先从 first.js
文件导入此函数;只有这样它才能调用它。请记住将带有 type="module"
的文件添加到 import
和 export
。
你不需要同时添加两个 .js
文件;添加导入函数的文件就足够了。例如,我们在 HTML 文件中添加一个 second.js
文件。
要使用 import
和 export
,你必须在服务器上运行你的应用程序(你可以使用 Node.js),因为它不能在本地机器上运行。你可以在 Visual Studio 代码中使用 live server
扩展,并使用 Open with Live Server
运行你的代码以用于学习目的。
有关 import
和 export
的更多信息,你可以查看 this 链接。
使用 jQuery 从一个 JS 文件到另一个 JS 文件的 JavaScript 调用函数
HTML 代码:
<!DOCTYPE html>
<html>
<head>
<title>Call Function from One JS file into another</title>
<script src="second.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
</head>
<body>
<input
id="circleArea"
type="button"
value="Show Circle Area"
onclick="circleArea()"
/>
</body>
</html>
first.js
代码:
const PiValue = 3.14;
let radius = 3;
function calcArea() {
return PiValue * radius * radius;
}
second.js
代码:
function circleArea() {
$.getScript('first.js', function() {
document.write(calcArea());
});
}
此示例代码还计算圆的面积,但使用 jQuery 将函数从 first.js
文件调用到 second.js
文件。
在 second.js
文件中,一旦 first.js
脚本使用 getScript()
方法完全加载,回调函数就会被触发。document.write
方法将输出写入 calcArea()
方法返回的浏览器中。