How to Get Element by Name in JavaScript
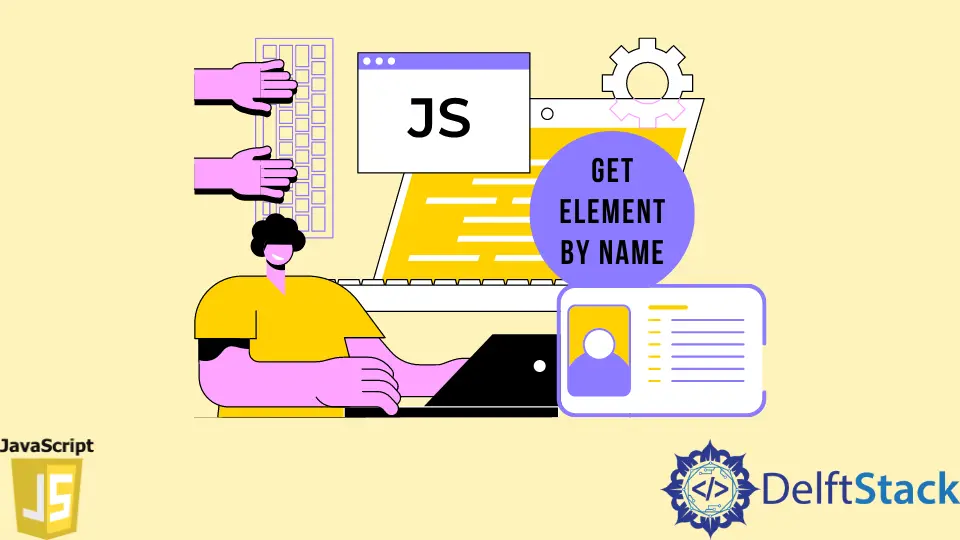
There are ways to get an element like getElementById()
and getElementByClass()
. But what if you want to select / edit all items with a specific name?
JavaScript provides the querySelectorAll()
or getElementsByName()
method to perform this task.
This article will introduce how to get elements by name in JavaScript.
Get Element by getElementsByName()
in JavaScript
getElementsByName
is a built-in document
method that returns the Objects/NodeList element whose name
attribute matches the specified name. This NodeList represents an array of nodes accessible using an index, and this index starts with 0
like any other array.
Syntax
document.getElementsByName(name);
name
is a required parameter that specifies the name
of an HTML attribute that must match. It returns the object of the corresponding DOM element if the elements are found; otherwise, it returns null
.
The difference between getElementsByName
and querySelectorAll
is that getElementsByName
first selects only those elements whose specified name attribute matches the given name. While querySelectorAll
can use any selector, providing more flexibility.
For additional information, read the documentation of the getElementById
method.
Example code:
<div>
<label>First Name</label>
<input type="text" id="firstName" name="firstName" value="John">
</div>
<div>
<label>Last Name</label>
<input type="text" id="lastName" name="lastName" value="Doe">
</div>
const firstName = document.getElementsByName('firstName');
const lastName = document.getElementsByName('lastName');
console.log(firstName[0].value);
console.log(lastName[0].value);
The code above defined two input elements with firstName
and lastName
. When we access the document with getElementsByName()
, it finds all nodes whose name attribute matches the first name and returns NodeList. Since this is an array, we can extract the first matching element with [0]
. If more than one matching item is found, you can iterate and print out all the values through the array. The output will look like this:
Output:
John
Doe
Get Element by querySelectorAll()
in JavaScript
querySelectorAll()
is a built-in document
method that returns the Element / NodeList objects whose selectors
match the specified selectors. Several selectors can also be transferred.
The difference between querySelectorAll()
and querySelector()
is that all objects of the matching item are returned first, and then the object of the single matching item. If an invalid selector is passed, a SyntaxError
shows.
Syntax
document.querySelectorAll(selectors);
selectors
is a required parameter that specifies the selector
of an HTML attribute that must match. You can pass multiple selectors by separating commas. For example, document.querySelectorAll('input[name=firstName]')
finds all input elements whose name is firstName. It returns matching DOM element objects if matching elements are found; otherwise, it returns an empty NodeList.
For additional information, read the documentation of the querySelectorAll
method.
Example code:
<div>
<label>FirstName</label>
<input type="text" id="firstName" value="John">
</div>
<div>
<label>Last Name</label>
<input type="text" id="lastName" value="Doe">
</div>
const selectors = document.querySelectorAll('input[name=firstName]');
console.log(selectors.length, selectors[0].value);
The code above defined two input elements with firstName
and lastName
. When we access the document with querySelectorAll()
, it finds all nodes whose name attribute matches the given name and returns NodeList. Since this is an array, we can extract the first matching element with [0]
. If more than one random element is found, you can iterate on the matrix and print all values. The output will look like this:
Output:
1
John
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn