Virtual Function in Java
- Virtual Function in Java
- Virtual Functions in Java Bean
- Non-Virtual Functions in Java
- Virtual Functions in Java interface
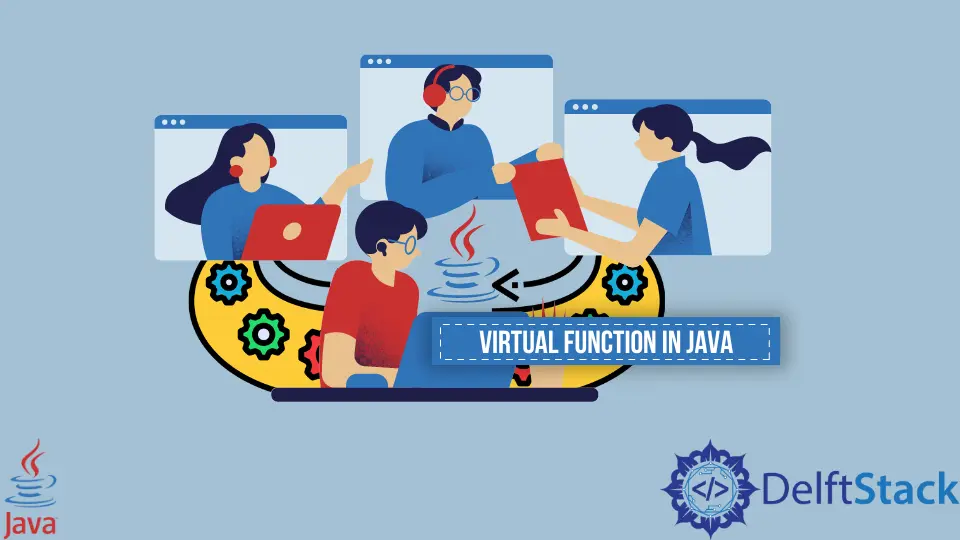
This tutorial introduces what a virtual function/method is in Java and how to use the virtual function in Java.
A function that is defined in a base class and can be overridden in a derived class is known as a virtual function. The concept of virtual function was used in C++ and in Java by default; all the non-private and non-final methods are virtual methods.
In C++, we use a virtual keyword to make virtual functions, but Java has no such keyword. And besides that, all the non-private and non-final methods are virtual.
The concept of a virtual function is useful in terms of object-oriented programming concepts and polymorphism. Let’s understand by some examples.
Virtual Function in Java
In this example, we created a class Human
that contains a virtual eat()
method. Since it is a virtual method, it can be overridden in the derived/child class, as we did in the example below. Both methods have the same signature, and when we call the function, only the child class method executes. See the example below.
class Human {
void eat(String choice) {
System.out.println("I would like to eat - " + choice + " now");
}
}
public class SimpleTesting extends Human {
void eat(String choice) {
System.out.println("I would like to eat - " + choice);
}
public static void main(String[] args) {
SimpleTesting simpleTesting = new SimpleTesting();
simpleTesting.eat("Pizza");
simpleTesting.eat("Chicken");
}
}
Output:
I would like to eat - Pizza
I would like to eat - Chicken
Virtual Functions in Java Bean
Getter functions of a bean/POJO class are also virtual and can be overridden by the child class. See the example below.
public class SimpleTesting extends Student {
public int getId() {
System.out.println("Id : " + id);
return id;
}
public String getName() {
System.out.println("Name : " + name);
return name;
}
public int getAge() {
System.out.println("Age : " + age);
return age;
}
public static void main(String[] args) {
SimpleTesting student = new SimpleTesting();
student.setId(101);
student.setName("Rohan");
student.setAge(50);
student.getId();
student.getName();
student.getAge();
}
}
class Student {
int id;
String name;
int age;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
Output:
Id : 101
Name : Rohan
Age : 50
Non-Virtual Functions in Java
Functions that are either private or final are non-virtual, so they cannot be overridden by the child class. In this example, we created two functions, one is final, and the second is private. We tried to override these into child class, but the java compiler raised an error due to the non-virtual function. See the example and output below.
class Human {
// non-virtual method
final void eat(String choice) {
System.out.println("I would like to eat - " + choice);
}
// non-virtual method
private void buy(String item) {
System.out.println("Buy me a " + item);
}
}
public class SimpleTesting extends Human {
// non-virtual method
void eat(String choice) {
System.out.println("I would like to eat - " + choice);
}
// non-virtual method
void buy(String item) {
System.out.println("Buy me a " + item);
}
public static void main(String[] args) {
SimpleTesting simpleTesting = new SimpleTesting();
simpleTesting.eat("Pizza");
simpleTesting.buy("Pizza");
simpleTesting.eat("Chicken");
simpleTesting.buy("Chicken");
}
}
Output:
java.lang.IncompatibleClassChangeError: class SimpleTesting overrides final method Human.eat(Ljava/lang/String;)
---Cannot override the final method from Human---
Virtual Functions in Java interface
An interface’s functions/methods are virtual by default because they are public by default and are supposed to be overridden by the child class. In the example below, we created a method in the interface, overridden it in a class, and called it successfully. See the example below.
interface Eatable {
void eat(String choice);
}
public class SimpleTesting implements Eatable {
public void eat(String choice) {
System.out.println("I would like to eat - " + choice);
}
void buy(String item) {
System.out.println("Buy me a " + item);
}
public static void main(String[] args) {
SimpleTesting simpleTesting = new SimpleTesting();
simpleTesting.eat("Pizza");
simpleTesting.buy("Pizza");
simpleTesting.eat("Chicken");
simpleTesting.buy("Chicken");
}
}
Output:
I would like to eat - Pizza
Buy me a Pizza
I would like to eat - Chicken
Buy me a Chicken