How to Update Value in Hashmap in Java
-
Update Value in Hashmap Using
hashmap.put()
in Java -
Update Value in Hashmap Using
hashmap.replace()
in Java
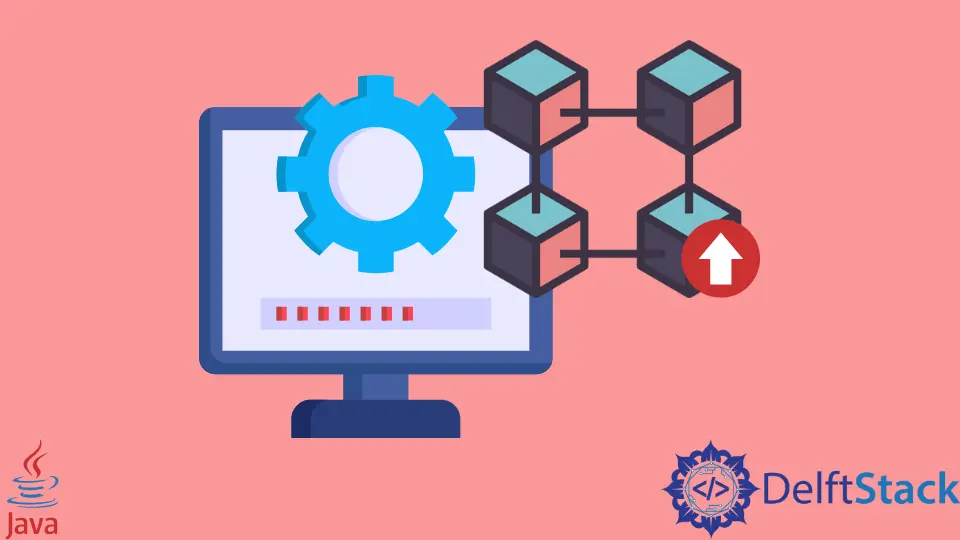
This article introduces how to update a value in a HashMap in Java using two methods - put()
and replace()
included in the HashMap class.
Update Value in Hashmap Using hashmap.put()
in Java
We use the put()
method with HashMap
when we want to insert a value into the HashMap
. And we can also use it to update the value inside the HashMap
. In the example below, we create an object of HashMap
, which is made up of key-value pairs and it is required to define the data type of both the key and the value during the initialization.
We use the string type for both key and value and we can find or do operations on the value using the key. Below, we replace the value that has the key three
with a new value. If there is no existing presence in the HashMap
that we want to update and use the put()
method, it will insert a new value. The output shows the updated value.
import java.util.HashMap;
public class UpdateHashmap {
public static void main(String[] args) {
HashMap<String, String> ourHashmap = new HashMap<>();
ourHashmap.put("one", "Alex");
ourHashmap.put("two", "Nik");
ourHashmap.put("three", "Morse");
ourHashmap.put("four", "Luke");
System.out.println("Old Hashmap: " + ourHashmap);
ourHashmap.put("three", "Jake");
System.out.println("New Hashmap: " + ourHashmap);
}
}
Output:
Old Hashmap: {four=Luke, one=Alex, two=Nik, three=Morse}
New Hashmap: {four=Luke, one=Alex, two=Nik, three=Jake}
Update Value in Hashmap Using hashmap.replace()
in Java
Another method that comes with the HashMap
class is replace()
that can update or replace an existing value in a HashMap
. The big difference between put()
and replace()
is that when a key does not exist in the HashMap
, the put()
method inserts that key and the value inside the HashMap
, but the replace()
method will return null. This makes replace()
safer to use when updating a value in a HashMap
.
In the following example, we create a HashMap
and insert some key-value pairs. Then to update the value attached to the key three
, we use ourHashMap.replace(key, value)
that takes two arguments, the first key that we want to update and the second the value.
import java.util.HashMap;
public class UpdateHashmap {
public static void main(String[] args) {
HashMap<String, String> ourHashmap = new HashMap<>();
ourHashmap.put("one", "Alex");
ourHashmap.put("two", "Nik");
ourHashmap.put("three", "Morse");
ourHashmap.put("four", "Luke");
System.out.println("Old Hashmap: " + ourHashmap);
ourHashmap.replace("three", "Jake");
System.out.println("New Hashmap: " + ourHashmap);
}
}
Output:
Old Hashmap: {four=Luke, one=Alex, two=Nik, three=Morse}
New Hashmap: {four=Luke, one=Alex, two=Nik, three=Jake}
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn