How to Throw Multiple Exception in Java
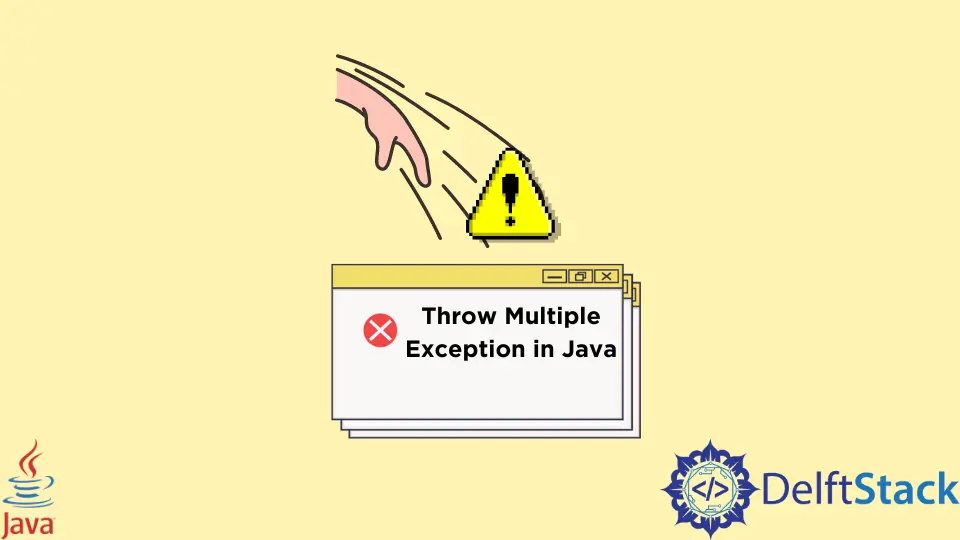
This tutorial will demonstrate how to throw multiple exceptions in Java.
Exceptions are the unwanted & unexpected events that disrupt the normal flow of the instructions during the program’s execution. The root class of all Java exceptions is java.lang.Throwable
. This class is inherited by Error and exception subclasses.
To handle exceptions, we use the try...catch
blocks.
In the try
block, we place the code which may raise some exception. The try
block is not used alone. It must always be followed by either catch
or finally
. The catch
block is used to catch the exception. This block can be followed by the finally block. The finally
block is used to execute the important code of the program no matter whether there is an exception or not.
In Java, we may have to deal with multiple exceptions. It is not possible to throw numerous exceptions in Java. We can specify multiple exceptions, but only one of them will be thrown.
However, we have a few alternatives that we may use to simulate throwing multiple exceptions.
We can use chained exceptions for dealing with multiple exceptions. Such exceptions indicate that the exception raised is caused by another exception.
For example,
public class Main {
public static void main(String[] args) {
try {
NumberFormatException ex = new NumberFormatException("NumberFormatException is thrown");
ex.initCause(new NullPointerException("NullPointerException is the main cause"));
throw ex;
}
catch (NumberFormatException ex) {
System.out.println(ex);
System.out.println(ex.getCause());
}
}
}
Output:
java.lang.NumberFormatException: NumberFormatException is raised
java.lang.NullPointerException: NullPointerException is the main cause
Note the use of initCause()
and getCause()
methods. The initCause()
function sets the cause of exception as another exception and the getCause()
will return the cause of the exception, in our case being NullPointerException
.
We can also use suppressed exceptions. Here we attach suppressed exceptions to the primary exception. It was added in Java 7.
To attach suppressed exceptions, we use the addSuppressed()
function. We use this function with the main exception object.
For example,
import java.io.*;
class Main {
public static void main(String[] args) throws Exception {
try {
Exception suppressed = new NumberFormatException();
Exception suppressed2 = new NullPointerException();
final Exception exe = new Exception();
exe.addSuppressed(suppressed);
exe.addSuppressed(suppressed2);
throw exe;
}
catch (Throwable e) {
Throwable[] suppExe = e.getSuppressed();
System.out.println("Suppressed:");
for (int i = 0; i < suppExe.length; i++) {
System.out.println(suppExe[i]);
}
}
}
}
Output:
Suppressed:
java.lang.NumberFormatException
java.lang.NullPointerException
In the above example, we attached two exceptions to the main object and printed the suppressed exceptions. To get all the suppressed exceptions, we use the getSuppressed()
function.