super in Java
- What is the super Keyword?
- Using super() to Call Superclass Constructors
- Accessing Superclass Methods with super
- Accessing Superclass Fields
- Conclusion
- FAQ
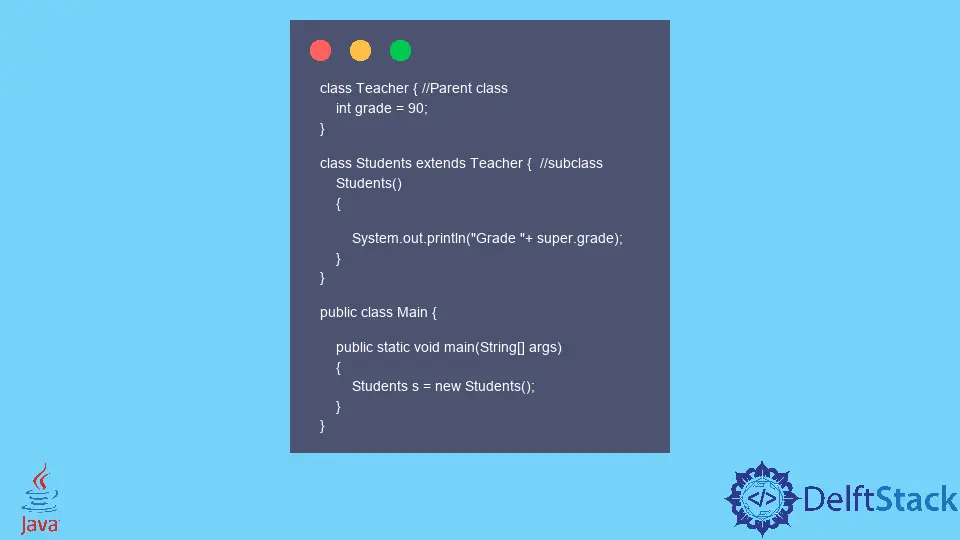
Understanding the concept of super
in Java is essential for anyone looking to grasp object-oriented programming in this versatile language. The super
keyword serves as a powerful tool that allows developers to access methods and variables from a parent class, enhancing code reusability and maintainability.
In this tutorial, we will delve into how to effectively use super()
in Java. Whether you are a beginner or an experienced programmer, this guide will provide you with clear examples and explanations to help solidify your understanding of this important feature. Let’s dive into the world of Java and explore the capabilities of the super
keyword.
What is the super Keyword?
In Java, the super
keyword is used to refer to the superclass (parent class) of the current object. It can be utilized in various contexts, such as calling a superclass constructor, accessing superclass methods, and accessing superclass fields. This keyword plays a pivotal role in inheritance, allowing derived classes to leverage the properties and behaviors of their parent classes.
For instance, when you create a subclass, it inherits all the accessible members (fields and methods) from its superclass. However, if the subclass has its own members with the same name, you can use super
to differentiate between them. This not only prevents naming conflicts but also promotes clarity in your code.
Using super() to Call Superclass Constructors
One of the primary uses of super()
is to call a constructor from the superclass. This is particularly useful when the superclass has parameters in its constructor that need to be initialized. When you create a subclass, you can invoke the superclass constructor using super()
to ensure that the inherited properties are properly set up.
Here’s an example to illustrate this concept:
class Animal {
String name;
Animal(String name) {
this.name = name;
}
}
class Dog extends Animal {
Dog(String name) {
super(name);
}
void display() {
System.out.println("Dog's name is: " + name);
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog("Buddy");
dog.display();
}
}
Output:
Dog's name is: Buddy
In this example, the Animal
class has a constructor that takes a String
parameter for the animal’s name. The Dog
class, which extends Animal
, calls the superclass constructor using super(name)
. This ensures that the name
field in the Animal
class is initialized correctly. The display
method then prints out the dog’s name, demonstrating successful inheritance and initialization.
Accessing Superclass Methods with super
Another important aspect of the super
keyword is its ability to access methods defined in the superclass. This can be particularly useful when you want to override a method in the subclass but still retain the functionality of the original method.
Let’s take a look at an example:
class Vehicle {
void start() {
System.out.println("Vehicle is starting");
}
}
class Car extends Vehicle {
void start() {
super.start();
System.out.println("Car is starting");
}
}
public class Main {
public static void main(String[] args) {
Car car = new Car();
car.start();
}
}
Output:
Vehicle is starting
Car is starting
In this case, the Vehicle
class has a start
method that prints a message indicating that the vehicle is starting. The Car
class overrides this method but also calls the superclass method using super.start()
. This allows the Car
class to add its own functionality while still retaining the behavior of the Vehicle
class. The output clearly shows both messages, demonstrating the effective use of super
to access superclass methods.
Accessing Superclass Fields
In addition to calling constructors and methods, the super
keyword can also be used to access fields defined in the superclass. This is particularly useful when there are fields in the subclass that have the same name as those in the superclass.
Here’s an example to illustrate this:
class Person {
String name = "John Doe";
}
class Employee extends Person {
String name = "Jane Doe";
void displayNames() {
System.out.println("Employee name: " + name);
System.out.println("Person name: " + super.name);
}
}
public class Main {
public static void main(String[] args) {
Employee emp = new Employee();
emp.displayNames();
}
}
Output:
Employee name: Jane Doe
Person name: John Doe
In this example, both the Person
and Employee
classes have a field named name
. When the displayNames
method is called, it uses super.name
to access the name
field from the Person
class, while name
without super
refers to the Employee
class’s field. This demonstrates how super
can be effectively used to avoid naming conflicts and clarify which field you are referring to.
Conclusion
The super
keyword in Java is a vital component of object-oriented programming, providing a way to access superclass methods, fields, and constructors. By understanding how to use super()
, you can enhance your Java programming skills, leading to cleaner and more maintainable code. Whether you are calling a constructor, accessing a method, or differentiating between fields, the super
keyword is an essential tool in your programming arsenal. As you continue to explore Java, keep the concept of super
in mind, and leverage its power to create more robust applications.
FAQ
-
What does the super keyword do in Java?
The super keyword in Java is used to refer to the superclass of the current object, allowing access to its methods, fields, and constructors. -
Can you use super() to call a superclass constructor?
Yes, you can use super() in a subclass constructor to call a constructor from its superclass, ensuring proper initialization. -
How do I access superclass methods using super?
You can call a superclass method in a subclass by using super.methodName(), which allows you to extend or modify the functionality of the superclass method. -
What happens if a subclass has a field with the same name as a superclass field?
If a subclass has a field with the same name as a superclass field, you can use super.fieldName to access the superclass field, avoiding naming conflicts. -
Is it mandatory to use super() in a subclass constructor?
It is not mandatory to use super() in a subclass constructor; if you don’t, the default constructor of the superclass will be called automatically. However, if the superclass does not have a default constructor, you must explicitly call a superclass constructor using super().