Static Class in Java
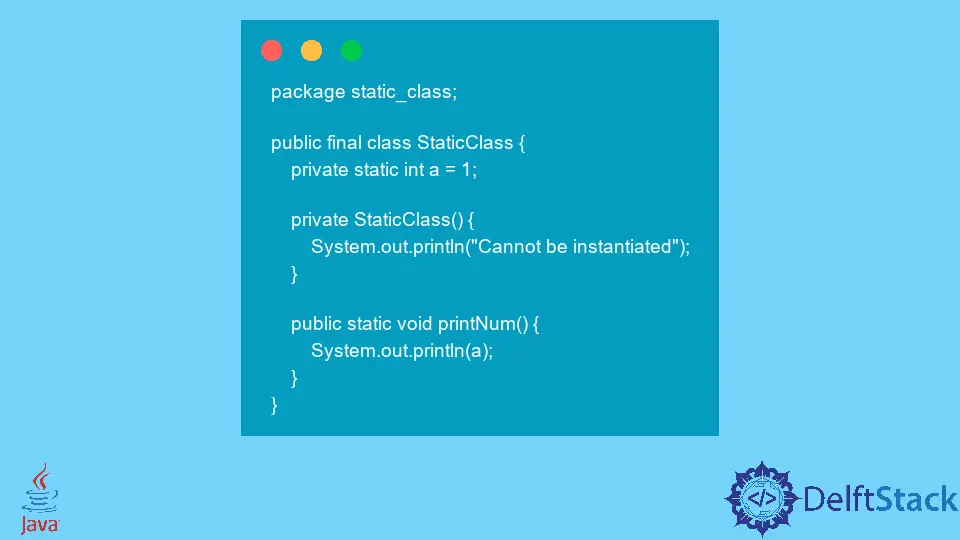
In the Java language, we cannot create an outer class as a static class, but there is a privilege to create a nested inner class as a static class. Properties of a static class are as follows.
A static inner class can never access a non-static member of the outer class.
A static inner class does not need any outer class reference to access the member variables.
Static class can never get instantiated. Hence, methods are directly accessible by the class name.
Below is the code that demonstrates the above two lines.
package static_class;
public class StaticInnerClass {
static int i = 1;
int j = 9;
public static void main(String[] args) {
StaticInnerClass s = new StaticInnerClass();
// s.InnerClass.printNum();
InnerClass.printNum();
}
public static class InnerClass {
public static void printNum() {
System.out.println(i);
// System.out.println(j);
}
}
}
In the above code, we have created an outer class with the StaticInnerClass
name. This class has a static field, non-static field, static method, and a static inner class. In the static main method, we have created an instance of an outer class named s
object. When we try to access the inner class printNum()
method with an outer class object, that is, s.InnerClass.printNum();
IDE gives a compile-time error, which states that static inner class does not need any reference of the outer class reference object to access inner class methods. Hence, we can directly access the inner class methods by class name.
Now when we see the definition of the printNum()
method. It has two print statements. First prints the static field of the outer class, and second tries to print the non-static variable of the same. The statement System.out.println(i);
is valid, which means that one can only access static members from the static context. But looking at the second statement, System.out.println(j);
is invalid, as one can never access non-static members from the static context.
In the Java class, there is no possibility to create an outer class static
class. But, we can imitate the behavior using some defined keywords.
The use of the final
keyword is to make the class final. This keyword prohibits the class from further extending. The reason being there is no use in the extension of a static class.
Use the private
keyword to make the constructor private since this prevents instantiation of a static class. As the property of the static class says, one can never instantiate it.
Make use of the static
keyword with member variables and member methods. Since no object gets created, one can never access non-static member fields and functions.
Below is a static class defined and its usage finds in making the Utility
class, where object creation of this class is not required.
package static_class;
public final class StaticClass {
private static int a = 1;
private StaticClass() {
System.out.println("Cannot be instantiated");
}
public static void printNum() {
System.out.println(a);
}
}
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn