How to Sort a List in Java
-
Use the
Collections.sort()
Method to Sort a List in Java -
Use the
Collections.reverseorder()
Method to Sort a List in Java -
Use the
Stream.sorted()
Method to Sort a List in Java -
Use
Comparator.naturalOrder()
Method to Sort an ArrayList in Java
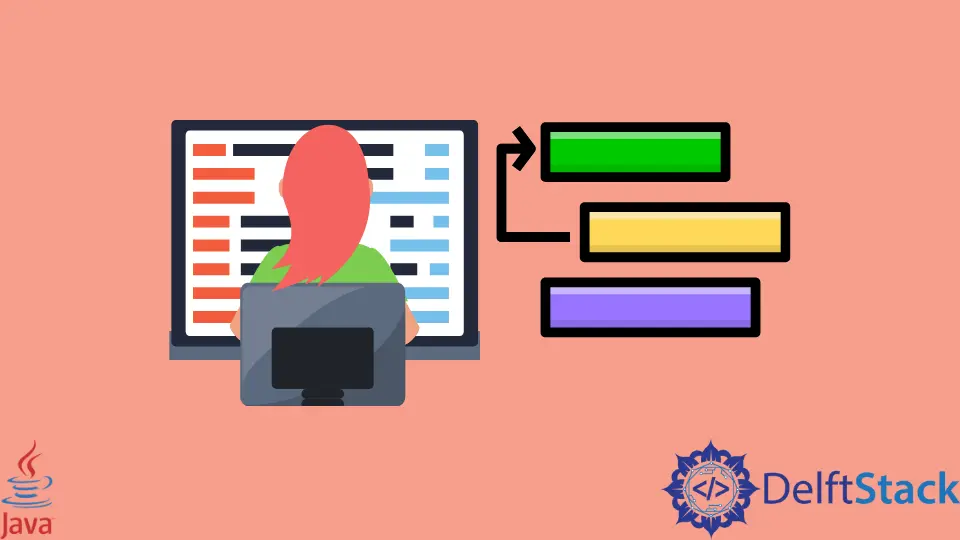
A list is an ordered collection and can store items in any order. We can apply traditional algorithms to a list.
This tutorial will demonstrate how to sort a list in Java using different functions.
Use the Collections.sort()
Method to Sort a List in Java
We can use the sort()
function from the Collections
Class to sort a list. We can take the list object, and it modifies the order of the elements. It sorts the list in ascending order.
For example,
import java.util.*;
import java.util.stream.*;
public class Main {
public static void main(String[] args) {
List<Integer> slist = Arrays.asList(4, 5, 1, 2, 8, 9, 6);
Collections.sort(slist);
System.out.println("After Sorting: " + slist);
}
}
Output:
After Sorting: [1, 2, 4, 5, 6, 8, 9]
We can see that the original list gets modified and sorted in the appropriate order in the above code.
Note that there should be the same types of elements in the list; otherwise, it will throw CLassCastException
.
Use the Collections.reverseorder()
Method to Sort a List in Java
We use the Collections.reverseorder()
method to sort the ArrayList in descending order. We don’t use this method directly. First, the Collections.sort()
method is used to sort in ascending order, and then the Collections.reverseorder()
method is used to sort in descending order.
See the code below.
import java.util.*;
import java.util.stream.*;
public class Main {
public static void main(String[] args) {
List<Integer> slist = Arrays.asList(4, 5, 1, 2, 8, 9, 6);
Collections.sort(slist, Collections.reverseOrder());
System.out.println("After Sorting: " + slist);
}
}
Output:
After Sorting: [9, 8, 6, 5, 4, 2, 1]
We have created a list in the above code and then sorted it using the Collections.sort()
method. Then it is sorted in descending order using the Collections.reverseorder()
method.
Use the Stream.sorted()
Method to Sort a List in Java
The sorted()
function is defined in the Stream
interface present in java.util package
. By using this method, the list gets sorted in ascending order. If the elements in the list are not of the same type, then it throws java.lang.ClassCastEcxeption
.
Here, we will use the stream()
function to manage the collection of elements in the list, collect()
will receive and store the elements, and the tolist()
function will return the final list in the sorted order.
For example,
import java.util.*;
import java.util.stream.*;
public class Main {
public static void main(String[] args) {
List<Integer> slist = Arrays.asList(4, 5, 1, 2, 8, 9, 6);
List<Integer> sortedList = slist.stream().sorted().collect(Collectors.toList());
System.out.println("After Sorting: " + sortedList);
}
}
Output:
After Sorting: [1, 2, 4, 5, 6, 8, 9]
Note that a new list is created in the above example.
Use Comparator.naturalOrder()
Method to Sort an ArrayList in Java
The Comparator
interface in Java can sort the objects of classes created by the user based on the desired data member. We can use it to sort lists also.
The naturalOrder()
function will sort the elements in ascending order.
See the code given below.
import java.util.*;
import java.util.stream.*;
public class Main {
public static void main(String[] args) {
List<Integer> slist = Arrays.asList(4, 5, 1, 2, 8, 9, 6);
slist.sort(Comparator.naturalOrder());
System.out.println("After Sorting: " + slist);
}
}
Output:
After Sorting: [1, 2, 4, 5, 6, 8, 9]
Null elements are sorted at the top by using this method. When it comes to alphabets, words that start with Capital Letters are sorted first then the words that start with small letters are sorted in ascending order.
We can use the reverseOrder()
function as discussed earlier to sort in descending order.
For example,
import java.util.*;
import java.util.stream.*;
public class Main {
public static void main(String[] args) {
List<Integer> slist = Arrays.asList(4, 5, 1, 2, 8, 9, 6);
slist.sort(Comparator.reverseOrder());
System.out.println("After Sorting: " + slist);
}
}
Output:
After Sorting: [9, 8, 6, 5, 4, 2, 1]