How to Resolve IllegalArgumentException in Java
- Hierarchy of IllegalArgumentException Class in Java
- Runtime Exceptions vs. Compile-Time Exceptions in Java
- Throw and Resolve IllegalArgumentException in Java
-
Use
Try
andCatch
to Determine IllegalArgumentException With Scanner in Java
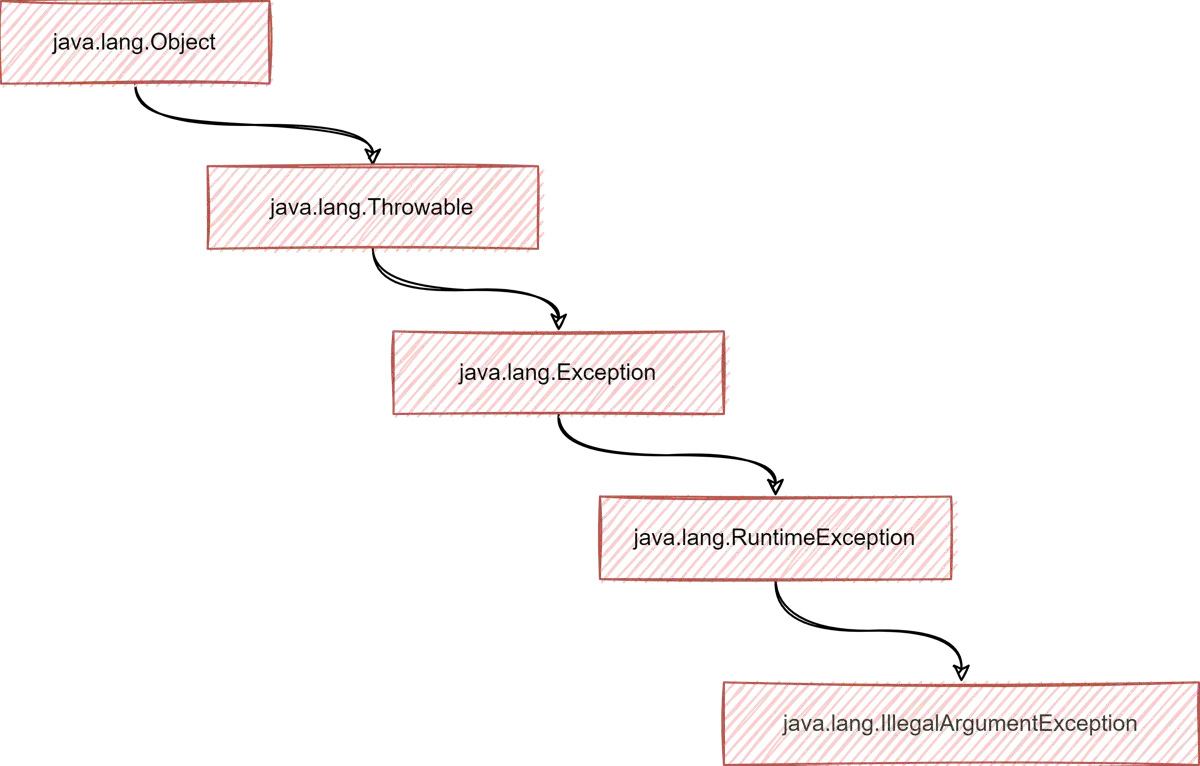
This tutorial will classify the exception hierarchy by showing the java.lang.IllegalArgumentException
class from the java.lang.object
class. After that, we will execute three Java coding blocks to demonstrate illegalargumentexceptions.
Finally, we will show how to get rid of them and explain how you can customize them.
Hierarchy of IllegalArgumentException Class in Java
This exception is triggered because we may have given an invalid parameter to a Java function. This exception extends the Runtime Exception class, as seen in the diagram below.
So, one exception can be triggered when the Java Virtual Machine is running. However, to grasp it completely, have a look at the following Java classes:
java.lang.object
: The root of the class hierarchy is the Class object.java.lang.Throwable
: The Throwable class is the superclass of all Java errors and exceptions.java.lang.Exception
: extends the Throwable class that identifies situations a practical application might want to catch.java.lang.RuntimeException
: Runtime Exception is the superclass for exceptions that can be generated when the Java Virtual Machine is running correctly.java.lang.IllegalArgumentException
: Thrown to notify that an illegal or inappropriate argument was supplied to a method.
Hierarchy diagram of illegalargumentexception:
Runtime Exceptions vs. Compile-Time Exceptions in Java
Although most people are familiar with the difference, many still do not understand the simplicity.
The table below illustrates the difference between runtime and compile-time error/exception types in Java.
No | Subject | Runtime Errors | Compile Time Errors |
---|---|---|---|
1 | Exceptions | Thrown during the normal execution of JVM | When a Java program’s semantic rules are broken, the JVM throws this error as an exception to the program. |
2 | Reference | Class Runtime Exception | Compiler Exceptions |
3 | Solution | After the execution and identification of the code. | During the development of the code. |
Throw and Resolve IllegalArgumentException in Java
The program below will throw an illegalargumentexception because we cannot pass a negative value as a parameter in the initial capacity of the array list.
Example 1:
package com.IllegalAE.util;
import java.util.ArrayList;
import java.util.List;
public class Example1 {
public static void main(String[] args) {
List<String> demoList = new ArrayList<>(-1);
demoList.add("Red");
demoList.add("Blue");
demoList.add("Green");
System.out.println(demoList);
}
}
Output:
If we do not pass a negative value in the initial list capacity like: List<String> demoList = new ArrayList<>(1);
, we get the following result.
Output:
[RED, GREEN, BLUE]
Example 2:
First, you should note the value of int a
is 1000, and the value of int b
is 0.
package com.IllegalAE.util;
// class
public class Example2 {
// main function
public static void main(String[] args) {
// constructor
Demo2 examp2 = new Demo2();
// add 2 numbers
System.out.println(" The total of A and B is: " + examp2.addTwoNums(10, 5));
}
}
class Demo2 {
public int addTwoNums(int a, int b) {
// if the value of b is less than the value of a (trigger IllegalArgumentException with a
// message)
if (b < a) {
throw new IllegalArgumentException("B is not supposed to be less than " + a);
}
// otherwise, add two numbers
return a + b;
}
} // class
Output:
The primary function contains the object passed through a constructor. The illegalargumentexception is triggered since the value of b
is less than a
.
If we swipe the values of int a
and b
just like in the code below,
System.out.println(" The total of A and B is: " + examp2.addTwoNums(0, 1000));
Output:
The total of A and B is: 15
You can also view the live demo below.
You can set your illegal argument conditions to take correct user input and control your logical flow.
Likewise, Java does not allow us to break the methods by passing illegal arguments. It is to allow users to write clean code and follow standard practices.
Another reason is to avoid illegal execution that might jeopardize the flow of the code blocks.
Use Try
and Catch
to Determine IllegalArgumentException With Scanner in Java
The program structure is written to determine the illegalargumentexception using the try
and catch
exception and Java scanner class.
Here are some points you need to consider first:
-
Understand the
java.util.Scanner
class.Scanner mySc = new Scanner(System.in); int demo = mySc.nextInt();
Note that the code above allows the user to read Java’s
System.in
. -
The
try...catch
Exception Handling:try { // It allows us to define a block of code that will be tested for errors while it runs. } catch (Exception e) { // It enables us to create a code block that will be invoked if an error occurs in the try block. }
We will be able to utilize the illegalargumentexception using the
try
andcatch
methods and modify it the way it suits us.
Example:
package com.IllegalAE.util;
import java.util.Scanner;
public class IllegalArgumentExceptionJava {
public static void main(String[] args) {
String AddProduct = "+";
execute(AddProduct);
}
static void execute(String AddProduct) {
// allows user to read System.in
// tries code block for testing errors
try (Scanner nxtLINE = new Scanner(System.in)) {
// to ignore user specified case consideration
while (AddProduct.equalsIgnoreCase("+")) {
// The code inside try block will be tested for errors
try {
System.out.println("Please enter a new product ID: ");
int Productid = nxtLINE.nextInt();
if (Productid < 0 || Productid > 10)
throw new IllegalArgumentException();
System.out.println(
"You entered: " + Productid); // if try block has no error, this will print
}
// The code within the catch block will be run if the try block has any error
catch (IllegalArgumentException i) {
System.out.println(
"Invalid Entry Detected!. Do you want to try it again?"); // if try block had an
// error, this would print
AddProduct = nxtLINE.next();
if (AddProduct.equalsIgnoreCase("AddProduct"))
execute(AddProduct);
}
}
}
}
}
Output:
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn