How to Read JSON File in Java
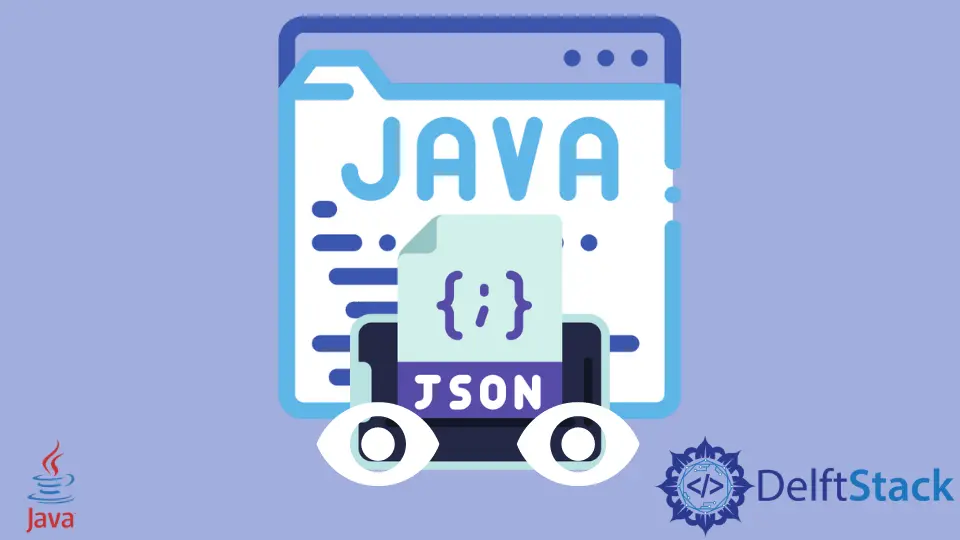
JavaScript Object Notation is light and text-based language for storing and transferring data. Objects and arrays are two data types represented by the JSON file.
This tutorial demonstrates how to read a JSON file in Java.
To read the JSON file, we will use the FileReader()
function to initiate a FileReader
object and read the given JSON file.
In our example, we will read the following file.
{
"firstName": "Ram",
"lastName": "Sharma",
"age": 26
},
"phoneNumbers": [
{
"type": "home",
"phone-number": "212 888-2365"
}
]
}
To parse the content of this file, we will use the json.simple
java library. We need to import two classes from the java.simple
library, the org.json.simple.JSONArray
and org.json.simple.JSONObject
class. The JSONArray
helps us read elements in the form of an array, and the JSONObject
helps us read the objects present in the JSON text.
In the code below, we have read a JSON file that is already there on the system, and from that, we will print the first and last name from the file.
For example,
import org.json.simple.JSONArray;
import org.json.simple.JSONObject;
import org.json.simple.parser.*;
public class JSONsimple {
public static void main(String[] args) throws Exception {
// parsing file "JSONExample.json"
Object ob = new JSONParser().parse(new FileReader("JSONFile.json"));
// typecasting ob to JSONObject
JSONObject js = (JSONObject) ob;
String firstName = (String) js.get("firstName");
String lastName = (String) js.get("lastName");
System.out.println("First name is: " + firstName);
System.out.println("Last name is: " + lastName);
}
}
Output:
First name is: Ram
Last name is: Sharma
We can see the first and last name that we have read from the JSON file in the above example.
Here we have used the JSONParser().parse()
present in the org.json.simple.parser.*
class in Java, which parses the JSON text from the file. The js.get()
method here gets the first and the last name from the file.
We can parse the JSON content using different methods, but the main file is read using the FileReader()
.
We can also parse JSON directly from strings. There are other methods also which can parse the JSON strings.
The below code demonstrates the org.json
method. Here we will be copying and pasting the complete file in the static string json
. Then we will make an object which will be then used in reading the JSON object and array from the file.
import org.json.JSONArray;
import org.json.JSONObject;
public class JSON2 {
static String json = "{\"contacDetails\": {\n" + // JSON text in the file is written here
" \"firstName\": \"Ram\",\n"
+ " \"lastName\": \"Sharma\"\n"
+ " },\n"
+ " \"phoneNumbers\": [\n"
+ " {\n"
+ " \"type\": \"home\",\n"
+ " \"phone-number\": \"212 888-2365\",\n"
+ " }\n"
+ " ]"
+ "}";
public static void main(String[] args) {
// Make a object
JSONObject ob = new JSONObject(json);
// getting first and last name
String firstName = ob.getJSONObject("contacDetails").getString("firstName");
String lastName = ob.getJSONObject("contacDetails").getString("lastName");
System.out.println("FirstName " + firstName);
System.out.println("LastName " + lastName);
// loop for printing the array as phoneNumber is stored as array.
JSONArray arr = obj.getJSONArray("phoneNumbers");
for (int i = 0; i < arr.length(); i++) {
String post_id = arr.getJSONObject(i).getString("phone-number");
System.out.println(post_id);
}
}
}
Output:
FirstName Ram
LastName Sharma
212 888-2365