Private Constructors in Java
- Defining Private Constructor in Java
- Private Constructor Using Singleton Pattern in Java
- Private Constructor Using Builder Pattern in Java
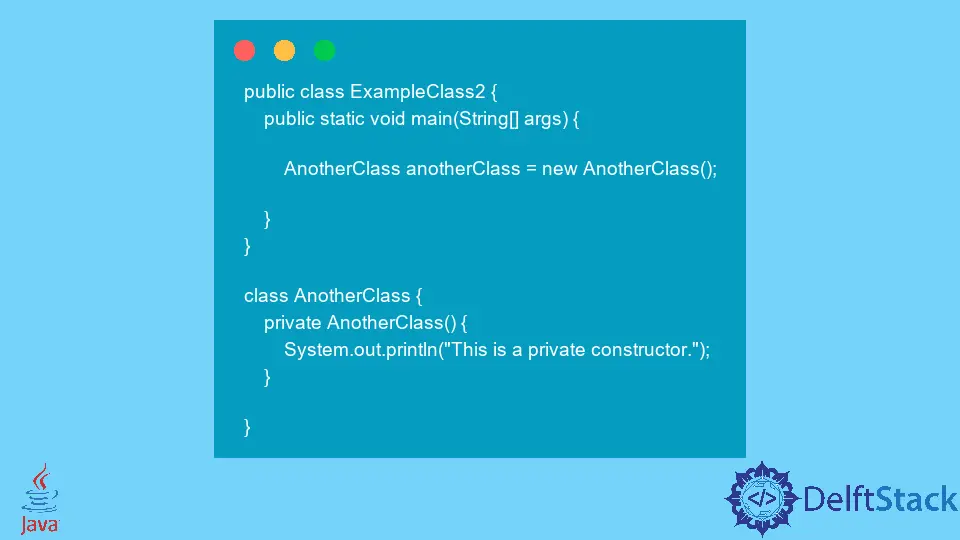
Constructor is one of the most important parts of a class as it is the first to execute and enables us to pass arguments that need to be initialized before anything in a class.
Usually, we create a constructor with a public modifier so that an object can be created in any function, but there are some scenarios when we want to make it private, and that is what we are going to look at in the following sections.
Defining Private Constructor in Java
In this section, we try to create an object of a class with a private constructor.
The program has two classes, ExampleClass2
and AnotherClass
. The AnotherClass
has a constructor with a private modifier and a print
statement.
When we create an object of this class in the ExampleClass2
class, we get an error saying that AnotherClass
has private access.
It restricts accessing the constructor, and it can be useful in certain ways that we will see in the next sections of this tutorial.
public class ExampleClass2 {
public static void main(String[] args) {
AnotherClass anotherClass = new AnotherClass();
}
}
class AnotherClass {
private AnotherClass() {
System.out.println("This is a private constructor.");
}
}
Output:
java: AnotherClass() has private access in com.tutorial.AnotherClass
Private Constructor Using Singleton Pattern in Java
We use the singleton pattern to create only a single class instance in the whole program. As a public constructor can be accessed from almost anywhere in the project, we use a private constructor.
We create a class AnotherClass
with a private constructor that displays a message in the program below. We need a function that returns an instance of the class, so we create a function called getInstance()
that is public and static to be called without creating an object.
Inside the getInstance()
method we return new AnotherClass()
and set the return type to AnotherClass
. We create another function to test if we can call the instance’s method.
Now we call the getInstance()
function that returns an instance of AnotherClass
and using this instance we call the dummyMethod()
.
public class ExampleClass2 {
public static void main(String[] args) {
AnotherClass anotherClass = AnotherClass.getInstance();
anotherClass.dummyMethod();
}
}
class AnotherClass {
private AnotherClass() {
System.out.println("This is a private constructor.");
}
public static AnotherClass getInstance() {
return new AnotherClass();
}
public void dummyMethod() {
System.out.println("This is just a dummy method");
}
}
Output:
This is a private constructor.
This is just a dummy method
Private Constructor Using Builder Pattern in Java
Another use case of a private constructor is when we use a builder pattern in our program. We create a User
class with three class variables firstName
, lastName
and age
in the following code.
We initialize all these variables in the constructor and make the constructor private.
We also make a method to print the values. We create an inner class named BuilderClass
to build the object inside the User
class.
BuilderClass
is public as another class can access it.
We create three variables in the BuilderClass
class to set the User
class’s variables. We create setter methods to set the values and then return this
with the values.
We return an object with the values by creating the buildObject()
method that returns the User
object with the new values that were set.
Now in the ExampleClass2
class, we get the BuilderClass()
class as it is public. Then, we use the UserBuilder
object to set the values using its setter methods and then call the buildObject()
method that returns the User
object.
We print the values by calling the getUserDetails()
function.
public class ExampleClass2 {
public static void main(String[] args) {
User.BuilderClass userBuilder = new User.BuilderClass();
User getUser = userBuilder.setFirstName("Micheal").setLastName("Fisk").setAge(26).buildObject();
getUser.getUserDetails();
}
}
class User {
private final String firstName;
private final String lastName;
private final int age;
private User(String firstName, String lastName, int age) {
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
}
public void getUserDetails() {
System.out.println("First Name: " + firstName);
System.out.println("Last Name: " + lastName);
System.out.println("Age: " + age);
}
public static class BuilderClass {
private String firstName;
private String lastName;
private int age;
BuilderClass setFirstName(String firstName) {
this.firstName = firstName;
return this;
}
BuilderClass setLastName(String lastName) {
this.lastName = lastName;
return this;
}
BuilderClass setAge(int age) {
this.age = age;
return this;
}
public User buildObject() {
return new User(firstName, lastName, age);
}
}
}
Output:
First Name: Micheal
Last Name: Fisk
Age: 26
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn